Interface Isolation Principle
In a video you see a very good example of the principle of interface isolation described by the story line, which is described in text here.
Story Start
In one scenario, a couple of couples, the boy bought a sports car for the girl; the girl reported to the driving school to prepare for the driving license test.
So let's start with a program to define this sports car.A sports car belongs to a kind of car and has the property of running. So we define a running interface for the sports car to perform this running function.
- Define the interface for running:
public interface IVehicle { void Run (); }
- Define a sports car class to achieve a running interface:
public class SportsCar : IVehicle { string name = "Sports car"; public void Run () { Console.WriteLine (name + "-Ruing : " + ">>>>>>>>>>>>"); } }
- Girls sign up for a school driver's license
public class Driver { private IVehicle _vehicle; public Driver (IVehicle vehicle) { _vehicle = vehicle; } public void Drive () { _vehicle.Run (); } }
- The girl drove her sports car up the street
class Program { static void Main (string[] args) { var driver=new Driver(new SportsCar()); driver.Drive(); } }
- The output is as follows:
Sports Car-Ruing:>>>>>>>>
Scenario 2: My sister chased the end of the sports car and called the man to cry. The man comforted me. Don't cry. My brother bought you a tank. My sister smiled with joy.
In the program, male-owned tanks are defined. Tanks are divided into three types according to component, namely, light tank, medium tank and heavy tank.Tanks can run and fire.
- Define the interface for running and firing
public interface ITank { void Run (); void Fire (); }
- Define lightweight tank class to implement ITank interface
public class LightTank : ITank { string name = "Light Tank"; public void Fire () { Console.WriteLine (name + "-Fire :" + ">>>"); } public void Run () { Console.WriteLine (name + "-Fire" + ">>>>>>>>>"); } }
- Define medium tank class to implement ITank interface
public class MediumTank : ITank { string name = "Medium tank"; public void Fire () { Console.WriteLine (name + "-Fire :" + ">>>>>>"); } public void Run () { Console.WriteLine (name + "-Runing :" + ">>>>>>"); } }
- Define heavy tanks for ITank interface
public class WeightTank : ITank { string name = "Heavy Tank"; public void Fire () { Console.WriteLine (name + "-Fire :" + ">>>>>>>>>"); } public void Run () { Console.WriteLine (name + "-Runing :" + ">>>"); } }
Scenario 3. The girl is ready to open a tank to the street
- The original license to learn to drive a sports car is as follows:
public class Driver { private IVehicle _vehicle; public Driver (IVehicle vehicle) { _vehicle = vehicle; } public void Drive () { _vehicle.Run (); } }
We found that we could not use the original driver's license to drive the tank.Looking back at our ITank interface, we found that this interface not only defines the Run method, but also the Fire method. When your sister can't go out of the street, fire the tank at will, so firing this function is not available to your sister.Then we need to fire this function, define a separate interface, and implement it in the class we need to use.
- Define an interface to fire:
public interface IWeapon { void Fire (); }
- Revamping the original ITank interface, ITank implements IVehicle, IWeapon interface
public interface ITank :IVehicle,IWeapon{}
- At this time, the girl could use her original license to drive the tank without changing her driver's license.The younger sister was happy to walk down the street with tanks of all sizes.
class Program { static void Main (string[] args) { var driver_SportsCar=new Driver(new SportsCar()); var driver_LightTank=new Driver(new LightTank()); var driver_weightTank = new Driver (new WeightTank ()); var driver_MediumTank=new Driver(new MediumTank()); driver_SportsCar.Drive(); driver_LightTank.Drive(); driver_MediumTank.Drive(); driver_weightTank.Drive(); } }
- I have it all in my hand; the output is as follows:
Sports Car-Ruing:>>>>>>>> Light Tank-Fire >>>>> Medium Tank-Runing: >>>> Heavy Tank-Runing: >>
Scenario 4: Add to the play, my sister suspects that she is not addicted to running tanks and wants to fly an airplane
- Define on IFly interface and inherit IVehicle interface
public interface IFly : IVehicle { void Fly (); }
- Implement Aircraft Class
public class AirPlane : IFly { string name = "aircraft"; public void Fly () { Console.WriteLine (name + "-Fly " + ">>>>>>>>>>>>>>>>>>"); } public void Run () { Console.WriteLine (name + "Ronglong:" + ">>>>>>>>>>>>>>>"); } }
- Sister flying
class Program { static void Main (string[] args) { var driver_Airplane = new Driver (new AirPlane ()); driver_Airplane.Drive (); } }
The output is as follows:
Airplane boom: >>>>>>>>>>>>>>>.
summary
-
Clients should not depend on interfaces they do not need to use
-
Class-to-class dependencies should be based on minimal interfaces
-
Use multiple specialized interfaces instead of a single overall interface. As mentioned above, the ITank interface inherits from the IVehicle and IWeapon interfaces, which implement the Run and Fire methods, respectively.When my sister drives a sports car, he doesn't need the firing feature, that is, the client shouldn't rely on interfaces it doesn't need.
More welcome:
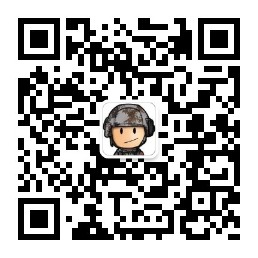