Why do you need a collection? The array has shortcomings: it is troublesome to expand the capacity, and the element type is fixed. The same array cannot be changed -------- -- > leads to the concept of set
Set system
Collections are divided into two categories: Collection (a set of objects) and Map (a set of key value pairs)
Underlying maintenance implementation relationship
ArrayList/Vector implements the List interface of Collection. The bottom layer is the variable type array Object []
LinkedList implements the List interface of Collection. The bottom layer is a two-way linked List
TreeSet implements the Set interface of Collection, and the underlying layer is TreeMap
HashSet implements the Set interface of Collection. The bottom layer is HashMap
LinkedHashSet implements the Map interface, and the underlying layer is LinkedHashMap
Hashtable implements the underlying Entry array of the Map interface
HashMap implements the Map interface. The bottom layer is a Node type array table. The implementation form in JDK7 is array + linked list. After JDK8, the implementation form is array + linked list + red black tree
LinkedHashMap implements the Map interface. The bottom layer is array + bidirectional linked list
choice
1. Judge the type of storage
A set of objects / a set of key value pairs
2. A group of objects [single column]
Collection interface
Allow duplicates: List
Additions and deletions: LinkedList[[bidirectional linked list] Modified query: ArrayList/Vector[Variable type Object Array]
Duplicate is not allowed: the bottom layer of Set is the implementation class of Map interface, and the Key of Map cannot be duplicate
Disorder: HashSet Sort: TreeSet The insertion and removal sequences are the same: LinkedHashSet
3. A set of key value pairs
Map interface keys are not allowed to be repeated and can be null. Values can be repeated and can be null (multiple). A pair of K-V -- > is placed in HashMap $node. Node implements the Entry interface. It can be said that a pair of K-V is an Entry
1. k-v Finally HashMap$Node 2. k-v In order to facilitate the programmer's traversal, it will also be created EntrySet Collection the type of element that the collection holds Entry And one Entry There are objects k,v EntrySet<Entry<k,v>> Namely transicent Set<Map.Entry<k,v>> entrySet; 3. entrySet The type defined in is Map.Entry But it's actually stored HashMap$Node that is because static class Node<K,V> implements Map.Entry<K,V> 4. Dangba HashMap$Node Object stored in entrySet It is convenient for us to traverse K getKey(); V getValue();
Key unordered: HashMap
Key sorting: TreeMap
Key insertion and extraction are consistent: LinkedHashMap
Read file: Properties
Parsing class
Vector Low safety and efficiency---ArrayList Unsafe and efficient !!!Capacity expansion mechanism
Complete process of ArrayList capacity expansion
TreeSet--TreeMap emphasizes custom sorting (anonymous inner class)
HashSet--HashMap thread is unsafe!!! Capacity expansion mechanism
Override hashCode equals to control that when the conditions are the same and the same hashCode is returned, no element can be added
LinkedHashSet
The order of adding is consistent with the order of taking out elements / data
The underlying layer of LinkedHashSet maintains a LinkedHashMap (a subclass of HashMap)
LinkedHashSet underlying structure (array + bidirectional linked list)
When adding for the first time, directly expand the array table to the node type LinkedHashMap\(Entry) stored in 16
The array is the element / data stored in HashMap\)Node [] and is of type dHashMap$EntrLinkey
The inheritance relationship is completed in the inner class static class Entry<K,V> extends HashMap.Node<K,V> { Entry<K,V> before, after; Entry(int hash, K key, V value, Node<K,V> next) { super(hash, key, value, next); } }
HashTable
Hashtable bottom
There is an array Hashtable$Entry [] in the bottom layer. The initialization size is 11. The threshold value is 8 = 11 * 0.75. The capacity expansion is carried out according to its own capacity expansion mechanism, and the method addEntry(hash, key, value, index) can be executed; Add k-v to the Entry. When if (count > = threshold) is satisfied, expand the capacity according to int newcapacity = (oldcapacity < < 1) + 1;
method
Collection:
Collections tool class method
List/Set method
add
remove
contains
size
isEmpty
addAll
containsAll
removeAll
get List interface has Set interface none
Map:
put
remove
get
size
isEmpty
clear
containsKey
ergodic
Collection:
Iterator
Enhanced for
Ordinary for
The Set interface implementation class does not have a get method and cannot be traversed by ordinary for
Map
Collections class
Collections are closely related ----- > use with generics
Traditional methods can not constrain the elements added to the set, which may have security risks Traversal requires type conversion. When the amount of data is large, it will affect the efficiency 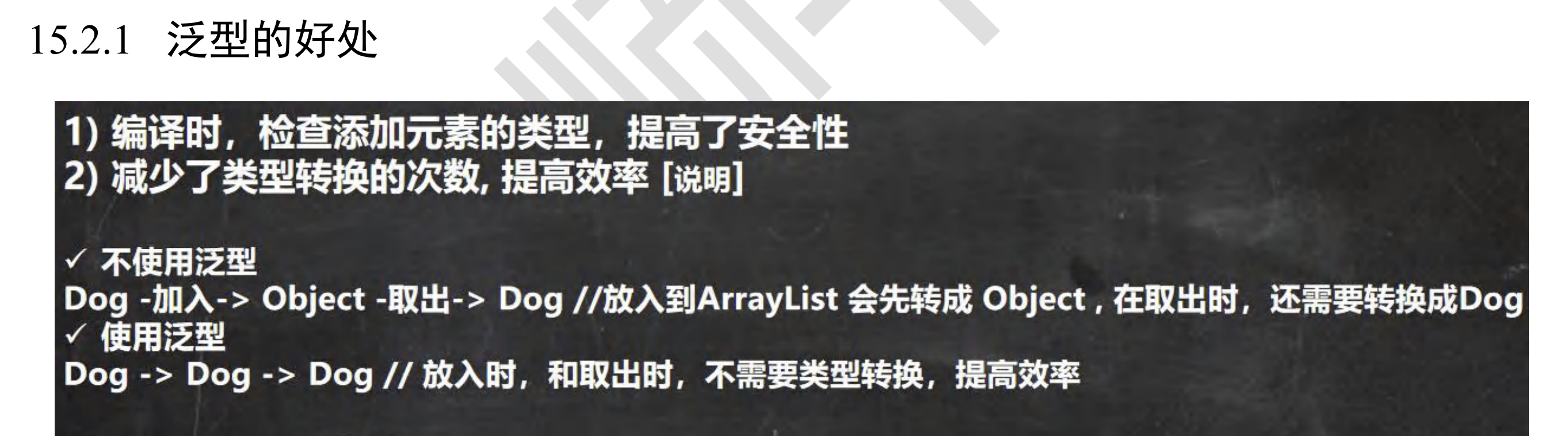
Classic topics
public class Main { public static void main(String[] args) { ArrayList<Employee> employees = new ArrayList<>(); Employee em1 = new Employee("zhc", 20000, new MyDate(2002, 7, 9)); Employee em2 = new Employee("myh", 10000, new MyDate(2000, 4, 2)); Employee em3 = new Employee("zhc", 5000, new MyDate(2001, 2, 5)); employees.add(em2); employees.add(em1); employees.add(em3); System.out.println("Before sorting"); System.out.println(employees); employees.sort(new Comparator<Employee>() { @Override public int compare(Employee emp1, Employee emp2) { //Sort by name first. If the names are the same, sort by birthday date. [i.e. customized sorting] //Verify the passed in parameters first if(!(emp1 instanceof Employee && emp2 instanceof Employee)) { System.out.println("Incorrect type.."); return 0; } //Compare name int i = emp1.getName().compareTo(emp2.getName()); if(i != 0) { return i; } return emp1.getBirthday().compareTo(emp2.getBirthday());//compareto method dynamically bound to MyDate class } }); System.out.println("After sorting"); System.out.println(employees); } } @SuppressWarnings({"all"}) class Employee { private String name; private double sal; private MyDate birthday; public Employee(String name, double sal, MyDate birthday) { this.name = name; this.sal = sal; this.birthday = birthday; } public String getName() { return name; } public void setName(String name) { this.name = name; } public double getSal() { return sal; } public void setSal(double sal) { this.sal = sal; } public MyDate getBirthday() { return birthday; } public void setBirthday(MyDate birthday) { this.birthday = birthday; } @Override public String toString() { return "\nEmployee{" + "name='" + name + '\'' + ", sal=" + sal + ", birthday=" + birthday + '}'; } } public class MyDate implements Comparable<MyDate> { private int year; private int month; private int day; public MyDate(int year, int month, int day) { this.year = year; this.month = month; this.day = day; } public int getMonth() { return month; } public void setMonth(int month) { this.month = month; } public int getDay() { return day; } public void setDay(int day) { this.day = day; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } @Override public String toString() { return "MyDate{" + "month=" + month + ", day=" + day + ", year=" + year + '}'; } @Override public int compareTo(MyDate o) { //year - month - day //If year is the same, compare Month int yearMinus = year - o.getYear(); if (yearMinus != 0) { return yearMinus; } //If year is the same, compare month int monthMinus = month - o.getMonth(); if (monthMinus != 0) { return monthMinus; } return day - o.getDay(); } }
Custom generics
Custom generic class
·Basic grammar
Class class name < T, R >{
member
}
·Details
Generic attribute methods can be used by ordinary members
Arrays that use generics cannot be initialized
Generics of classes cannot be used in static methods
The type of a generic class is determined when the object is created, because the type needs to be specified when the object is created
If no type is specified when creating an Object, it defaults to Object
Custom generic interface
·Basic grammar
Interface interface name < T, R >{
}
·Details
The members in the interface are static, so generics cannot be used
The type of generic interface is determined in the inheritance interface or implementation interface
No type is specified. The default is Object
Custom generic method
·Details
Generic methods can be defined in ordinary classes or in generic classes
When a generic method is called, the type is determined
There is no < T, R > eat method after the public void eat (E, e) modifier. It is not a generic method, but a generic method is used