catalogue
1, Preliminary understanding of classes and objects
2.private implementation encapsulation
1, Preliminary understanding of classes and objects
1.C language is process oriented, focusing on the process, analyzing the steps to solve the problem, and gradually solving the problem through function call
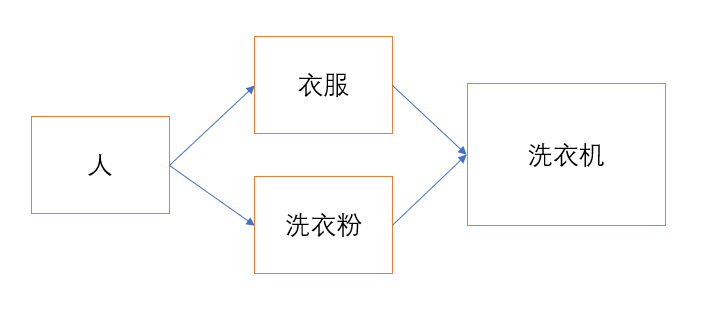
Object oriented is a way of thinking and a kind of thought. For example: concepts and examples. Theory and practice. Name and reality, etc. Its advantage is to make complex things simple, just face an object. Object oriented design holds an important experience: who owns the data and who provides external methods to operate the data (private) (the passive party is the owner of the data and the active party is the executor). During development: find objects, build objects, use objects, and maintain the relationship between objects
// Create class class <class_name>{ field;//Member properties method;//Member method } // Instantiate object <class_name> <Object name> = new <class_name>();
class Person { public int age;//Member property instance variable public String name; public String sex; public void eat() {//Member method System.out.println("having dinner!"); } public void sleep() { System.out.println("sleep!"); } } public class Main{ public static void main(String[] args) { Person person = new Person();//Instantiate objects through new Person person2 = new Person();//Generate object # instantiate object person.eat();//Member method calls need to be called through the reference of the object person.sleep(); } }
Compile and run the code, and the output is as follows:
having dinner!
sleep
2, Member of class
Class members can include the following: fields, methods, code blocks, internal classes and interfaces. However, this note focuses on the first three
1. field
class Person { public String name; // field public int age; } class Test { public static void main(String[] args) { Person person = new Person(); System.out.println(person.name); System.out.println(person.age); } }
Compile and run the code, and the output is as follows:
null
0
(1) use The field of the access object. Access includes both read and write
class Person { public String name; public int age; } class Test { public static void main(String[] args) { Person person = new Person(); person.name = "Zhang San"; person.age = 18; System.out.println(person.name); System.out.println(person.age); } }
Compile and run the code, and the output is as follows:
Zhang San
18
In fact, it can also be initialized inside Person, but we don't recommend this, because when we new multiple objects, not every object is called Zhang San, who is 18 years old
2. Method
Used to describe the behavior of an object
Methods are divided into ordinary methods and static methods. The following classes are ordinary methods. Static methods will be encountered later in the notes
class Person { public int age; public String name; public void show() { System.out.println("My name is" + name + ", this year" + age + "year"); } } class Test { public static void main(String[] args) { Person person1 = new Person(); Person person2 = new Person(); person1.age = 18; person1.name = "Zhang San"; person2.age = 19; person2.name = "Li Si"; person1.show(); person2.show(); } }
Compile and run the code, and the output is as follows:
My name is Zhang San. I'm 18 years old
My name is Li Si. I'm 19 years old
3.static keyword
It can modify attributes, methods, code blocks and classes
(1) Decorated attributes (Java static attributes are related to classes and independent of specific instances. In other words, different instances of the same class share the same static attribute)
class Test{ public int a;//Ordinary member variables and instance variables are stored in the object public static int count;//Class variables are also called static member variables public final SIZE;//What is modified by final is called a constant, which also belongs to an object. It is modified by final and cannot be changed later public static final int COUNT = 99;//Static constants belong to the class itself. Only one is modified by final and cannot be changed later } public class Main{ public static void main(String[] args) { Test t1 = new Test(); t1.a++; Test.count++; System.out.println(t1.a); System.out.println(Test.count); System.out.println("---------------"); Test t2 = new Test(); t2.a++; Test.count++; System.out.println(t2.a); System.out.println(Test.count); } }
Compile and run the code, and the output is as follows:
1
1
---------------
1
2
Note: count is modified by static and shared by all classes. And it does not belong to the object. The access method is: class name Attributes
Memory resolution is as follows:
(2) modification method
class TestDemo{ public int a; public static int count; public static void change() { count = 100; //a = 10; error non static data members cannot be accessed } } public class Main{ public static void main(String[] args) { TestDemo.change();//Can be called without creating an instance object System.out.println(TestDemo.count); } }
Compile and run the code, and the output is as follows:
100
3, Encapsulation
1. What is encapsulation
2.private implementation encapsulation
class Person { private String name = "Zhang San"; private int age = 18; public void show() { System.out.println("My name is" + name + ", this year" + age + "year"); } } class Test { public static void main(String[] args) { Person person = new Person(); //person.name = "Zhang San"// error person.show(); } }
3.getter and setter methods
- When the formal parameter name of the set method is the same as the name of the member attribute in the class, if this is not used, it is equivalent to self assignment This indicates the reference of the current instance
- Not all fields must be provided with setter / getter methods, but which method should be provided according to the actual situation
- In IDEA, you can use alt + insert (or alt + F12) to quickly generate setter / getter methods In VSCode, you can use the right mouse button menu - > source code operation to automatically generate setter / getter methods
class Person { private String name;//Instance member variable private int age; public void setName(String name){ //name = name;// It cannot be written like this. At this time, all three names are the same name, because local variables take precedence this.name = name;//this reference represents the object that calls the method } public String getName(){ return name; } public void show(){ System.out.println("name: "+name+" age: "+age); } } public static void main(String[] args) { Person person = new Person(); person.setName("xiaoxiao"); String name = person.getName(); System.out.println(name); person.show(); }
Compile and run the code, and the output is as follows:
xiaoxiao
name: xiaoxiao age: 0
4, Construction method
class Person { private String name;//Instance member variable private int age; private String sex; public Person() { //The default constructor constructs the object. The public here cannot be modified to private. Once modified in this way, the object cannot be instantiated outside the class, but it can be instantiated inside the class this.name = "caocao"; this.age = 10; this.sex = "male"; } public Person(String name,int age,String sex) { //A constructor with three parameters constitutes an overload between two constructors this.name = name; this.age = age; this.sex = sex; } public void show(){ System.out.println("name: "+name+" age: "+age+" sex: "+sex); } } public class Main{ public static void main(String[] args) { Person p1 = new Person();//Call the constructor without parameters. If the program does not provide it, it will call the constructor without parameters p1.show(); Person p2 = new Person("zhangfei",80,"male");//Call the constructor with 3 parameters p2.show(); } }
Compile and run the code, and the output is as follows:
class Person{ private String name; public Person(){ //this();//error calls other construction methods of the object, forming infinite recursion this("xiaoxiao");//Calling a constructor with one parameter must be placed on the first line System.out.println("Person()-->Construction method without parameters"); } public Person(String name){ this.name = name; System.out.println("Person(String)-->Construction method with one parameter"); } public void show() { System.out.println("name: "+name); } } public class Test{ public static void main(String[] args){ Person person = new Person(); person.show(); System.out.println(person); } }
Compile and run the code, and the output is as follows:
xiaoxiao
Person (string) -- > construction method with 1 parameter
Person() -- > constructor without parameters
5, Code block
1. The initialization methods of fields are: (1) local initialization, (2) initialization using construction methods, and (3) initialization using code blocks. The first two methods have been summarized earlier. Next, we introduce the third method, which uses code block initialization
2. According to the location and keywords defined by the code block, it can be divided into four types: local code block (ordinary code block), static code block, construction code block and synchronous code block
(1) Native code block: a code block defined in a method
public class Main{ public static void main(String[] args) { { //Directly use {} definition, common method block int x = 10 ; System.out.println("x1 = " +x); } int x = 100 ; System.out.println("x2 = " +x); } }
Compile and run the code, and the output is as follows:
x1 = 10
x2 = 100
This usage is rare
(2) Construct code block
class Person{ private String name;//Instance member variable private int age; private String sex; public Person() { System.out.println("I am Person init()!"); } //Instance code block { this.name = "bit"; this.age = 12; this.sex = "man"; System.out.println("I am instance init()!"); } public void show(){ System.out.println("name: "+name+" age: "+age+" sex: "+sex); } } public class Main { public static void main(String[] args) { Person p1 = new Person(); p1.show(); } }
Compile and run the code, and the output is as follows:
class Person{ private String name;//Instance member variable private int age; private String sex; private static int count = 0;//Static member variables are shared by classes in the data method area public Person(){ System.out.println("I am Person init()!"); } //Instance code block { this.name = "bit"; this.age = 12; this.sex = "man"; System.out.println("I am instance init()!"); } //Static code block static { count = 10;//Only static data members can be accessed System.out.println("I am static init()!"); } public void show(){ System.out.println("name: "+name+" age: "+age+" sex: "+sex); } } public class Main { public static void main(String[] args) { Person p1 = new Person(); Person p2 = new Person();//The static code block will not be executed } }
Compile and run the code, and the output is as follows:
I am instance init()!
6, Supplement
1.toString method:
(1) The toString method will be called automatically at println
(2) The operation of converting an object into a string is called serialization
(3) ToString is the method provided by the Object class. The Person class created by ourselves inherits from the Object class by default. You can override toString method to implement our own version of conversion string method
(4) Shortcut key of toString method for IDEA to quickly generate Object: alt+f12(insert)
You don't need shortcut keys. Right click to find generate, then find toString(), and then click ok
class Person { private String name; private int age; public Person(String name,int age) { this.age = age; this.name = name; } public void show() { System.out.println("name:"+name+" " + "age:"+age); } } public class Main { public static void main(String[] args) { Person person = new Person("caocao",19); person.show(); //We found that the hash value of an address is printed here. The reason: the toString method of Object is called System.out.println(person); } }
Compile and run the code, and the output is as follows:
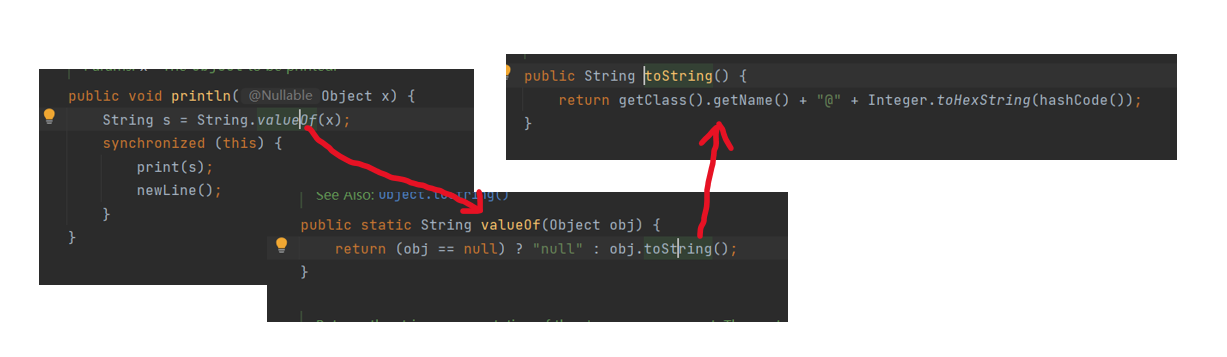
Override toString method:
class Person { private String name; private int age; public Person(String name,int age) { this.age = age; this.name = name; } public void show() { System.out.println("name:"+name+" " + "age:"+age); } //Override the toString method of Object @Override//@Override is called "Annotation" in Java. Here @ override means that the toString method implemented below is a method that overrides the parent class public String toString() { return "Person{" + "name='" + name + '\'' + ", age=" + age + '}'; } } public class Main { public static void main(String[] args) { Person person = new Person("caocao",19); person.show(); System.out.println(person); } }
Compile and run the code, and the output is as follows:
2. Anonymous object
(1) Objects that are not referenced are called anonymous objects
(2) Anonymous objects can only be used when creating objects
(3) If an object is used only once and does not need to be used later, consider using anonymous objects
class Person { private String name; private int age; public Person(String name,int age) { this.age = age; this.name = name; } public void show() { System.out.println("name:"+name+" " + "age:"+age); } } public class Main { public static void main(String[] args) { new Person("caocao",19).show();//Calling methods through anonymous objects } }
Compile and run the code, and the output is as follows: