1. Declare control
There are two styles of progress bars
- bar
- default
The two styles work as follows:
self.view.backgroundColor = UIColor.red progressView = UIProgressView(progressViewStyle: .default) progressView.frame = CGRect(x: 0, y: 0, width: 200, height: 10) progressView.layer.position = CGPoint(x: self.view.frame.width/2, y: 90) progressView.progress = 0.5 self.view.addSubview(progressView) progressView2 = UIProgressView(progressViewStyle: .bar) progressView2.frame = CGRect(x: 0, y: 0, width: 200, height: 20) progressView2.layer.position = CGPoint(x: self.view.frame.width/2, y: 110) progressView2.progress = 0.5 self.view.addSubview(progressView2)
Operation effect:
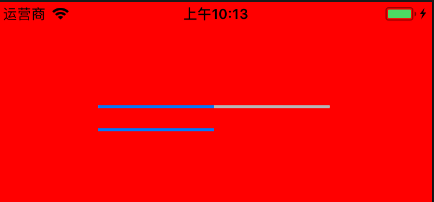
Progress bar style
2. Enable progress bar loading animation
progressView.setProgress(0.5, animated: true)
3. Change the progress bar color
progressView.progressTintColor = UIColor.green //Progress color progressView.trackTintColor = UIColor.yellow //Remaining progress colors
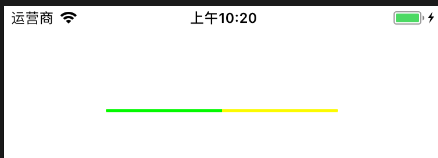
Set progress bar color
4. Set the height of progressView
In fact, careful partners should have found that when declaring controls, the height of the first height:10 and the second height: 20 have no difference from the screenshot, so setting the height of progressView cannot achieve the goal, but we can change the scale of progressView to achieve the height change
progressView = UIProgressView(progressViewStyle: .default) progressView.frame = CGRect(x: 0, y: 0, width: 200, height: 10) progressView.layer.position = CGPoint(x: self.view.frame.width/2, y: 90) progressView.setProgress(0.5, animated: true) progressView.progressTintColor = UIColor.green //Progress color progressView.trackTintColor = UIColor.yellow //Remaining progress colors //By changing the height of the progress bar (the width remains the same, and the height becomes twice the default) progressView.transform = CGAffineTransform(scaleX: 1.0, y: 2.0) self.view.addSubview(progressView) progressView2 = UIProgressView(progressViewStyle: .bar) progressView2.frame = CGRect(x: 0, y: 0, width: 200, height: 20) progressView2.layer.position = CGPoint(x: self.view.frame.width/2, y: 110) progressView2.progress = 0.5 self.view.addSubview(progressView2)
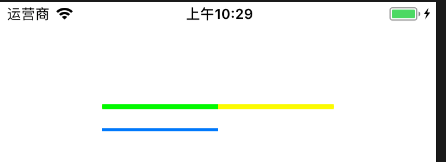
Change progress bar height
5. Others
Recommend a custom progress bar style: http://www.code4app.com/thread-6304-1-1.html It is implemented in OC. You may need to be familiar with the use of OC control in swift first
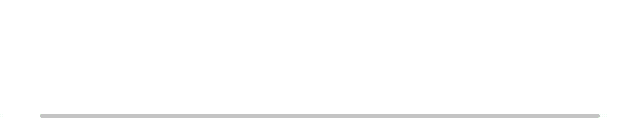
Progress bar with small label