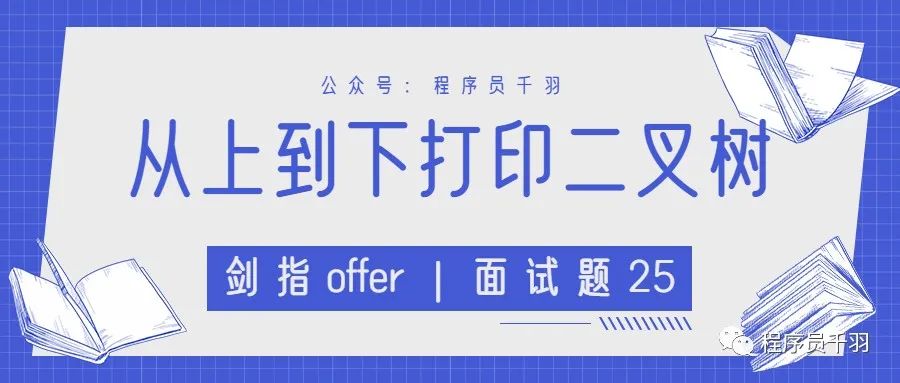
Knock algorithm series articles
- Ten classic sorting algorithms for dry goods and hand tearing
- Sword finger offer | understanding interview
- Sword finger offer | interview question 2: realizing Singleton mode
- Sword finger offer | interview question 3: finding two-dimensional array
- Sword finger offer | interview question 4: replace spaces
- Sword finger offer | interview question 5: print linked list from end to end
- Jianzhi offer | interview question 6: Rebuilding binary tree
- Sword finger offer | interview question 7: realizing queue with two stacks
- Sword finger offer | interview question 8: minimum number of rotation array
- Sword finger offer | interview question 9: Fibonacci sequence
- Sword finger offer | interview question 10: frog jumping steps
- Sword finger offer | interview question 11: matrix coverage
- Sword finger offer | interview question 12: the number of 1 in binary
- Sword finger offer | interview question 13: integer power of value
- Sword finger offer | interview question 14: print from 1 to the maximum n digits
- Sword finger offer | interview question 15: delete the node of the linked list
- Sword finger offer | interview question 16: put the odd number in the array before the even number
- Sword finger offer | interview question 17: the penultimate node in the lin k ed list
- Sword finger offer | interview question 18: reverse linked list
- Sword finger offer | interview question 19: merge two ordered linked lists
- Sword finger offer | interview question 20: judge whether binary tree A contains subtree B
- Sword finger offer | interview question 21: mirror image of binary tree
- Sword finger offer | interview question 22: print matrix clockwise
- Sword finger offer | interview question 23: stack containing min function
- Sword finger offer | interview question 24: Press in and pop-up sequence of stack
"Leetcode :
- I: https://leetcode-cn.com/problems/cong-shang-dao-xia-da-yin-er-cha-shu-lcof
- II: https://leetcode-cn.com/problems/cong-shang-dao-xia-da-yin-er-cha-shu-ii-lcof
- III: https://leetcode-cn.com/problems/cong-shang-dao-xia-da-yin-er-cha-shu-iii-lcof
"GitHub : https://github.com/nateshao/leetcode/blob/main/algo-notes/src/main/java/com/nateshao/sword_offer/topic_25_levelOrder/Solution.java
Sword finger Offer 25 Print binary tree I from top to bottom
Title Description: print each node of the binary tree from top to bottom, and the nodes of the same layer are printed from left to right.
For example: given binary tree: [3,9,20,null,null,15,7],
3 / \ 9 20 / \ 15 7
return:
[3,9,20,15,7]
Prompt: total number of nodes < = 1000
Problem solving ideas:
- The top-down printing of binary tree required by the topic (i.e. printing by layer), also known as breadth first search of binary tree (BFS).
- BFS is usually implemented by means of the first in first out feature of the queue.
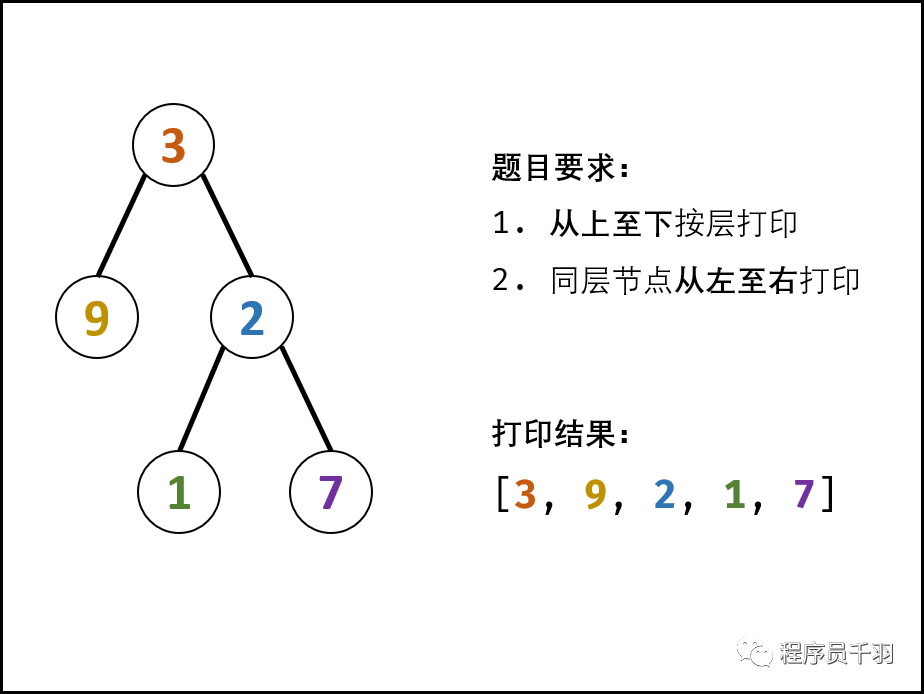
Algorithm flow:
- Special case handling: when the root node of the tree is empty, the empty list [] is returned directly;
- Initialization: print result list res = [], queue containing root node = [root];
- BFS cycle: jump out when the queue is empty;
- Out of the team: the first element out of the team is recorded as node;
- Printing: node Val is added to the tmp tail of the list;
- Add child node: if the left (right) child node of node is not empty, the left (right) child node will be added to the queue;
- Return value: return the print result list res.
Complexity analysis:
- Time complexity O(N): N is the number of nodes in the binary tree, that is, BFS needs to cycle N times.
- Spatial complexity O(N): in the worst case, that is, when the tree is a balanced binary tree, there are at most N/2 tree nodes in the queue at the same time, and the additional space of O(N) size is used.
package com.nateshao.sword_offer.topic_25_levelOrder; import java.util.ArrayList; import java.util.LinkedList; import java.util.Queue; /** * @date Created by Shao Tongjie on 2021/11/29 14:57 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: The idea of printing binary tree from top to bottom: assisted by queue (linked list). * * add Add a meta cable. If the queue is full, an iiiegaislabeeplian exception will be thrown * remove Remove and return the element at the head of the queue. If the queue is empty, a NoSuchElementException is thrown * element Returns the element of the queue header. If the queue is empty, a NoSuchElementException is thrown * offer Add an element and return true. If the queue is full, return false * poll Remove and return the element of the queue header. If the queue is empty, null is returned * peek Returns the element of the queue header. If the queue is empty, null is returned * put Add an element and block if the queue is full * take Removes and returns the element at the head of the queue. If the queue is empty, it is blocked */ public class Solution { /** * queue * * @param root * @return */ public int[] levelOrder(TreeNode root) { if (root == null) return new int[0];//An empty tree returns an empty array ArrayList<Integer> list = new ArrayList<>();// Apply for a dynamic array ArrayList to dynamically add node values Queue<TreeNode> queue = new LinkedList<>(); queue.offer(root);// The root node joins the team first while (!queue.isEmpty()) { TreeNode node = queue.poll();// Take out the current team leader element list.add(node.val); if (node.left != null) queue.offer(node.left);// Left child node queue if (node.right != null) queue.offer(node.right);// Right child node queue } // Convert ArrayList to an int array and return int[] res = new int[list.size()]; for (int i = 0; i < res.length; i++) { res[i] = list.get(i); } return res; } /************ Recursion*************/ public ArrayList<Integer> PrintFromTopToBottom2(TreeNode root) { ArrayList<Integer> list = new ArrayList<Integer>(); if (root == null) { return list; } list.add(root.val); levelOrder(root, list); return list; } public void levelOrder(TreeNode root, ArrayList<Integer> list) { if (root == null) { return; } if (root.left != null) { list.add(root.left.val); } if (root.right != null) { list.add(root.right.val); } levelOrder(root.left, list); levelOrder(root.right, list); } public class TreeNode { int val; TreeNode left; TreeNode right; TreeNode(int x) { val = x; } } }
Sword finger Offer 25 - II Print binary tree II from top to bottom
Topic Description: print the binary tree by layer from top to bottom. The nodes of the same layer are printed from left to right, and each layer is printed to one line.
"For example: given binary tree: [3,9,20,null,null,15,7],
3 / \ 9 20 / \ 15 7
Return its hierarchy traversal result:
[ [3], [9,20], [15,7] ]
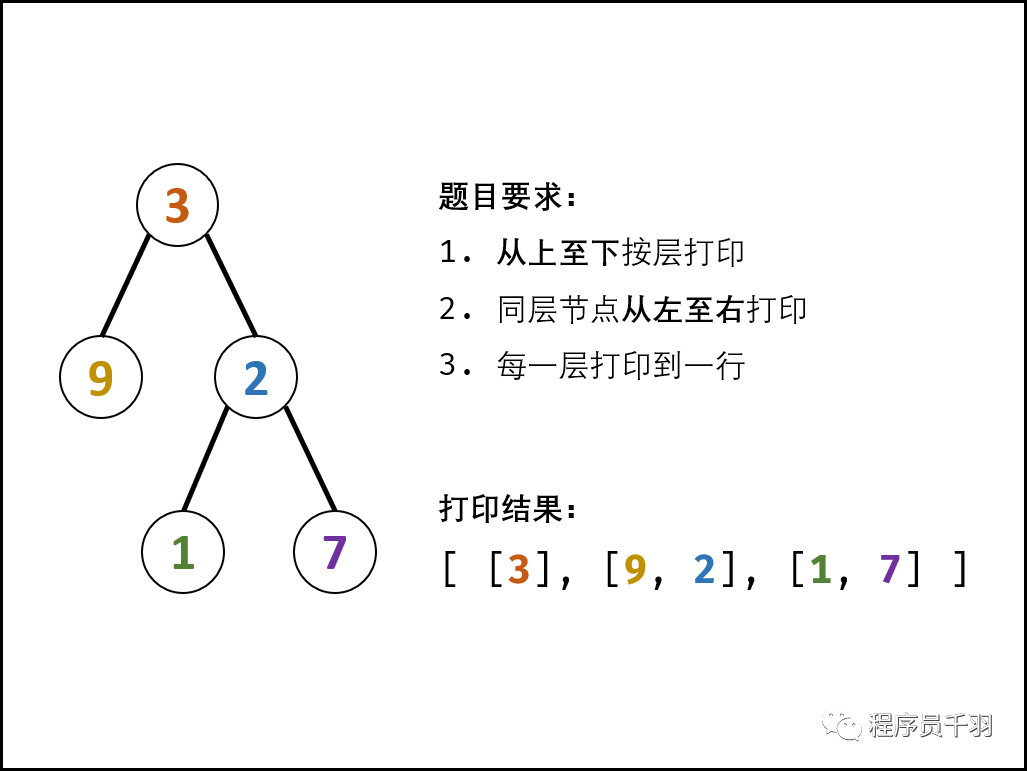
public List<List<Integer>> levelOrder(TreeNode root) { Queue<TreeNode> queue = new LinkedList<>(); List<List<Integer>> res = new ArrayList<>(); if(root != null) queue.add(root); while(!queue.isEmpty()) { List<Integer> tmp = new ArrayList<>(); for(int i = queue.size(); i > 0; i--) { TreeNode node = queue.poll(); tmp.add(node.val); if(node.left != null) queue.add(node.left); if(node.right != null) queue.add(node.right); } res.add(tmp); } return res; }
Sword finger Offer 25 - III. print binary tree III from top to bottom
Title Description: please implement a function to print the binary tree in zigzag order, that is, the first line is printed from left to right, the second layer is printed from right to left, the third line is printed from left to right, and so on.
For example: given binary tree: [3,9,20,null,null,15,7],
3 / \ 9 20 / \ 15 7
Return its hierarchy traversal result:
[ [3], [20,9], [15,7] ]
class Solution { public List<List<Integer>> levelOrder(TreeNode root) { Deque<TreeNode> deque = new LinkedList<>(); List<List<Integer>> res = new ArrayList<>(); if(root != null) deque.add(root); while(!deque.isEmpty()) { // Print odd layers List<Integer> tmp = new ArrayList<>(); for(int i = deque.size(); i > 0; i--) { // Print from left to right TreeNode node = deque.removeFirst(); tmp.add(node.val); // Join the lower layer nodes from left to right if(node.left != null) deque.addLast(node.left); if(node.right != null) deque.addLast(node.right); } res.add(tmp); if(deque.isEmpty()) break; // If it is empty, it will jump out in advance // Print even layers tmp = new ArrayList<>(); for(int i = deque.size(); i > 0; i--) { // Print right to left TreeNode node = deque.removeLast(); tmp.add(node.val); // Join the lower layer node from right to left if(node.right != null) deque.addFirst(node.right); if(node.left != null) deque.addFirst(node.left); } res.add(tmp); } return res; } }
Reference connection:
- https://leetcode-cn.com/problems/cong-shang-dao-xia-da-yin-er-cha-shu-lcof/solution/mian-shi-ti-32-i-cong-shang-dao-xia-da-yin-er-ch-4
- https://leetcode-cn.com/problems/cong-shang-dao-xia-da-yin-er-cha-shu-ii-lcof/solution/mian-shi-ti-32-ii-cong-shang-dao-xia-da-yin-er-c-5
- https://leetcode-cn.com/problems/cong-shang-dao-xia-da-yin-er-cha-shu-iii-lcof/solution/mian-shi-ti-32-iii-cong-shang-dao-xia-da-yin-er--3