demand
The user-defined TabBar has been implemented before, as shown in the figure:
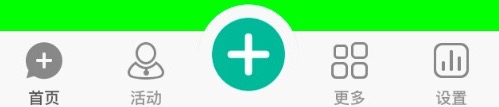
Now you need to implement a function similar to today's headline TabBar - if you continue to click the selected item of the current TabBar, the UI table view needs to be refreshed.
Analysis
Now that TabBar has been customized, the simplest thing is to add an event to the UI tabbarbutton needed in TabBar in customization - click to send a notification and pass out the current index. The corresponding interface listens for the notice and gets the index comparison. If it is consistent with the current index, the corresponding operation will be performed.
Realization
- Customize the binding events in the layoutSubviews of TabBar
- (void)layoutSubviews { [super layoutSubviews]; for (UIButton * tabBarButton in self.subviews) { if ([tabBarButton isKindOfClass:NSClassFromString(@"UITabBarButton")]) { //Monitor tabbar Click //Bind tag ID tabBarButton.tag = index; //Monitor tabbar Click [tabBarButton addTarget:self action:@selector(tabBarButtonClick:) forControlEvents:UIControlEventTouchUpInside]; } } }
- Listening events, sending notifications
- (void)tabBarButtonClick:(UIControl *)tabBarBtn{ //Judge whether the current button is the previous button //Click the same item again and send a notice to capture and judge the corresponding VC if (self.previousClickedTag == tabBarBtn.tag) { [[NSNotificationCenter defaultCenter] postNotificationName: @"DoubleClickTabbarItemNotification" object:@(tabBarBtn.tag)]; } self.previousClickedTag = tabBarBtn.tag; }
- Corresponding UIViewController listens for notifications
- (void)viewDidLoad { [super viewDidLoad]; [[NSNotificationCenter defaultCenter]addObserver:self selector:@selector(doubleClickTab:) name:@"DoubleClickTabbarItemNotification" object:nil]; }
- Listen to the notice and perform the operation after comparison
-(void)doubleClickTab:(NSNotification *)notification{ //There's a hole here. If you use NSInteger directly, there will be a problem. The number is wrong //Because the last interface is encapsulated as an object when it is transmitted, it is received with NSNumber and then retrieved NSNumber *index = notification.object; if ([index intValue] == 1) { //Refresh } }
Supplement on April 28, 2018
After this article is reprinted, there are many good readers to criticize and correct: this way is not elegant enough, not simple enough. How is it easiest? In fact, as long as the agent of uittabbarcontroller is rewritten, it can be implemented as follows
//This is the agent method of uitabarcontroller - (BOOL)tabBarController:(UITabBarController *)tabBarController shouldSelectViewController:(UIViewController *)viewController{ // To determine which interface needs to be refreshed by clicking again, take the first VC as an example if ([tabBarController.selectedViewController isEqual:[tabBarController.viewControllers firstObject]]) { // Judge whether the re selected controller is the current controller if ([viewController isEqual:tabBarController.selectedViewController]) { // Execution operation NSLog(@"Refresh interface"); return NO; } } return YES; }