Hello, I'm[ 🌑 This is the back of the moon.
Today, I'd like to introduce how to use Python to build a reservation elevator control system. The directory is as follows:
- Design platform
- Design of independent control elevator system
- Character model class
- Random floor generation class
- Elevator class
- Buildings
- Design of group control elevator system
- summary
Recently, I found that the elevator system is also an interesting project in the process of commuting. The independent control elevator may not be so attractive, but the system design in the group control elevator seems interesting. How to effectively use all elevators in the elevator system to accurately, time-saving and comfortable pick up and deliver all passengers on the waiting floor of the building to the target floor has become a direction for thinking.
Design platform
- windows 10
- python 3.8
Design of independent control elevator system
In essence, the operation of each elevator room of the group control elevator is the independent operation of a single elevator. Therefore, the independent elevator operation system is designed first, and the flow chart is drawn simply as follows:
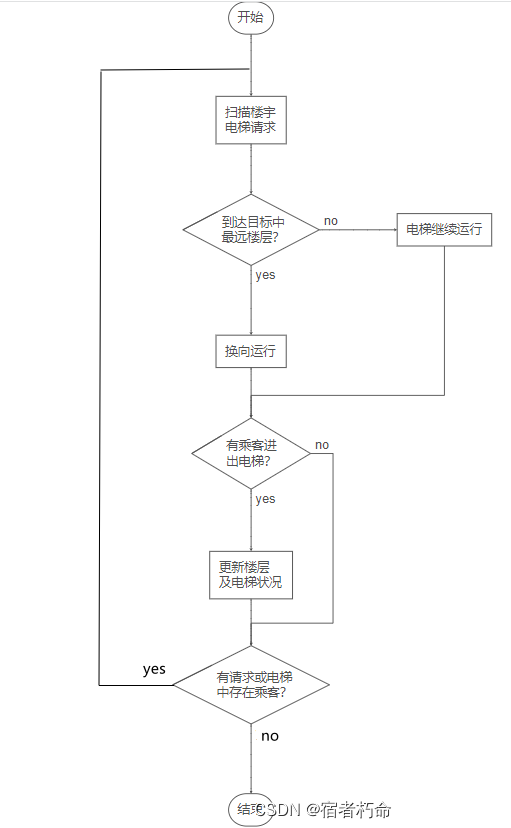
Through the program, scan the upstairs and downstairs requests of all floors of the building, drive the elevator to arrive, and complete the needs of passengers from the request floor to the target floor. Main code maps:
def take_elevator(self, elevator_data): """Enter the elevator""" if not elevator_data: return elevator_data elevator_data = pd.DataFrame(elevator_data, dtype='int') data = elevator_data[elevator_data['up'] == self.up].copy() data2 = elevator_data[elevator_data['up'] != self.up].copy() while data['weight'].sum() > self.weight and not data.empty: # overweight weight_max = data[data['weight'] == data['weight'].max()].index data2 = data2.append(data.loc[weight_max, :]) data.drop(weight_max, inplace=True) while len(data) > self.persons and not data.empty: # overload weight_min = data[data['weight'] == data['weight'].min()].index data2 = data2.append(data.loc[weight_min, :]) data.drop(weight_min, inplace=True) self.person_data = self.person_data.append(data) self.update_person_data(data, 'sub') return data2.to_dict(orient='records')
In order to better simulate the request acceptance state of real elevator, building class, character model class, elevator class and randomly generated character class are constructed. When the number of passengers in the elevator is greater than the specified number or the load is greater than the limited number, the elevator will not carry passengers on the current floor.
Character model class
Enter the floor, go to the floor range, and return the attribute of the person taking the elevator.
class PeopleRandom: """ Constructing random character model Return the attribute value of each person, including: floor, weight, whether to go upstairs and to floor """ def __init__(self, floor: int, floors: tuple = (1, 30), people: int = 1): self.floor = floor self.floor_min, self.floor_max = floors self.people = people self.weight = self.set_weight() self.up = random.randint(0, 1) self.floor_go = self.go()
Random floor generation class
The incoming floor range and the maximum generated number of people on this floor return the passengers on all floors of the building.
class FloorsRandom: """ Random floor generation, floor random number generation. """ def __init__(self, floor_min: int, floor_max: int, people: int = 6): """ The entered floor does not exist in 0 floor, so it is in floor_min Add 1 and then take random data. If it is less than or equal to 0, subtract 1 to restore the lowest floor. :param floor_min: Lowest floor :param floor_max: Highest floor """
Elevator class
Range of elevator to and from the floor, weight and load limit, operation direction and passenger status in the elevator.
class Elevator: """ Elevator model: The lowest floor, the highest floor and the current floor that can be run """ def __init__(self, floor_min, floor_max, floor: int = 1): self.floor = floor self.go_max = self.floor self.floor_min = floor_min self.floor_max = floor_max self.up = 1 # 1: Elevator up, 0: elevator down self.weight = 1000 # Elevator weight limit self.persons = 12 # Elevator limit number of personnel self.person_data = pd.DataFrame(columns=['floor', 'weight', 'up', 'floor_go'])
Buildings
Floor range of elevator operation between buildings and waiting passengers.
class BuildingList: """ Building model: Lowest floor """ def __init__(self, floor_min: int = -1, floor_max: int = 30): self.floor_min = floor_min self.floor_max = floor_max self.data = pd.DataFrame(columns=['floor', 'weight', 'up', 'floor_go']) self.set_data_all('simple')
In order to simplify the operation and simplify the design of some models, the elevator can send all waiting passengers to each designated floor by randomly generating the character model from the highest floor to the lowest floor of the building.
Design model code is open source:
https://github.com/lk20200413/FunnyCodeRepository/tree/main/ Reservation elevator task system
Design of group control elevator system
The design of group control elevator system can effectively alleviate the waiting time of passengers on each floor and improve the comfort of passengers in the elevator.
Taking myself as an example, the elevator in the community is an independent control elevator. In order to take the elevator earlier, I will press the request button of each elevator, resulting in varying degrees of congestion in the elevator or prolonging the waiting time of other passengers.
I didn't consult relevant data before this design. The overall design is certainly not as good as the existing mature algorithm design. I call this system task system, which should have a more professional algorithm corresponding to it. In short, take the inter floor request as a task, and the system arranges the task and distributes it to each elevator. After a period of time, the system scans the requests in the building again until there is no request, converts each elevator to an independent elevator mode, and completes the destination floor request of passengers in the elevator.
Task system design flow chart:
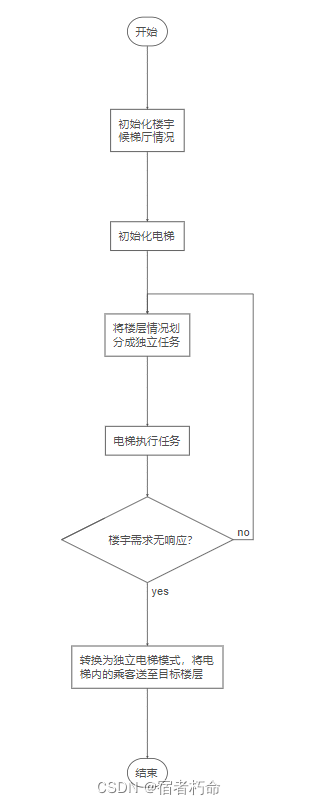
Execution flow chart of each elevator:

The process drawing is rough. Please make do with it. The task system adopts distance first code design, which is limited to the length of the article. It is inconvenient to display the relevant code parts, so you can move to
https://github.com/lk20200413/FunnyCodeRepository/tree/main/ Reservation elevator task system
Here or contact the author for the code, tasksystem Py is the task system design module, and the calls of related classes follow the independent elevator design.
summary
Hello, I'm[ 🌑 This is the back of the moon. By designing a task-based reservation elevator system with Python, this paper has a deeper understanding that the conversion from independent elevator to group control elevator is not only a simple combination of elevators. The design factors of the whole elevator system should focus on time operation, and each elevator should be divided into multi-threaded operation. In this design, the time factor is simplified, and the operation difference between elevators is replaced by skipping floors.
Any good thing in the world is worth remembering. We should not give up the right to pursue our dreams because of the unbearable situation at present!
Made on 29 December 2021