1. Monitor, filter and interceptor pairs
(1) Servlet: Processing Request requests and Response responses
(2) Filter: Filter requests before Servlet if configured to /* access all resources (servlet, js/css static resources, etc.) filter processing
(3) Monitor (
Listener
): Implemented
javax.servlet.ServletContextListener
Connected server-side components that follow
Web
Should be started Start, only initialize times, then direct transport monitor, follow
Web
Should be destroyed
Muscle making:
Do some initialization work,
web
Ying Xin Zhong
spring
Container Start
ContextLoaderListener
Making muscles:
Monitor
web
Specific events in, such as
HttpSession,ServletRequest
Creation and destruction; Variable creation,
Destroy, modify, etc. Processing can be added before and after certain actions to achieve monitoring, e.g. counting the number of online mussels, profit mussels
HttpSessionLisener
Wait.
(4) Interceptors (
Interceptor
): Yes
SpringMVC
,
Struts
Equal performance layer framework, will not intercept
jsp/html/css/image
Access to, etc., will only block access to the controller_method (
Handler
).
It can also be concluded from the configured pitch:
serlvet
,
fifilter
,
listener
Is configured in
web.xml
Of,
interceptor
yes
Configured in the Configuration Part of the Representation Layer Framework
- Intercept times before Handler business logic enforcement
- Intercept times before Handler Logical Execution is completed but not jumped
- Intercept after jump
2. Interceptor implementation process
When running a program, the interceptor's holds are in a fixed order, which is related to the order in which interceptors are defined in the configuration file. A single interceptor, the execution process in the program is as follows:
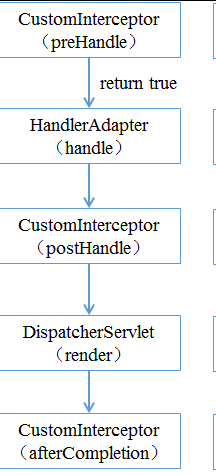
1
) Procedural preemption_
preHandle()
Method if the return value of the method is
true
, the program will continue to hold down_method in the processor, otherwise it will not hold down_again.
2
) On the business processor (that is, the controller)
Controller
Class) After processing the request, will hold_
postHandle()
_, and then passes
DispatcherServlet
Return a response to the client.
3
) in
DispatcherServlet
You will not hold until you have processed the request
afterCompletion()
_method.
3. Multiple Interceptor Enforcement Process
Multiple interceptors (assume you have two interceptors)
Interceptor1
and
Interceptor2
And in the configuration,
Interceptor1
The interceptor is configured first, and the execution process in the program is as follows:
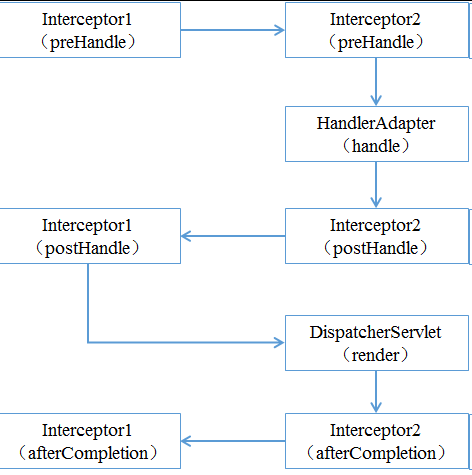
As you can see from the diagram, when multiple interceptors are working at the same time, their
preHandle()
_Method will execute_, _their interceptors in the order they are configured in the configuration file
postHandle()
_Method and
afterCompletion()
The rule will be inverted in the order of configuration.
4. Code Samples
_Define the Spring MVC interceptor
package com.lagou.edu.interceptor; import org.springframework.web.servlet.HandlerInterceptor; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Custom springmvc interceptor */ public class MyIntercepter01 implements HandlerInterceptor { /** * Executes before handler method business logic executes * Permission checking is often done here * @param request * @param response * @param handler * @return The return value boolean denotes release, true denotes release, and false denotes abort * @throws Exception */ @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { System.out.println("MyIntercepter01 preHandle......"); return true; } /** * Executes when the handler method business logic has not been executed before jumping to the page * @param request * @param response * @param handler * @param modelAndView The view and data are encapsulated and the page has not been jumped yet. Here you can modify the returned data and view information * @throws Exception */ @Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { System.out.println("MyIntercepter01 postHandle......"); } /** * Execute after page has skipped rendering * @param request * @param response * @param handler * @param ex Exceptions can be caught here * @throws Exception */ @Override public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception { System.out.println("MyIntercepter01 afterCompletion......"); } }
SpringMVC. Register SpringMVC interceptor in XML
<mvc:interceptors> <!--Intercept All handler--> <!--<bean class="com.lagou.edu.interceptor.MyIntercepter01"/>--> <mvc:interceptor> <!--Configuring the current interceptor's url Interception rules,**Represents all under the current directory and its subdirectories url--> <mvc:mapping path="/**"/> <!--exclude-mapping Can be used in mapping Exclude some on the basis of url Intercept, if you want to exclude some URL Must have first<mvc:mapping path="/**"/>--> <!--<mvc:exclude-mapping path="/demo/**"/>--> <bean class="com.lagou.edu.interceptor.MyIntercepter01"/> </mvc:interceptor> <mvc:interceptor> <mvc:mapping path="/**"/> <bean class="com.lagou.edu.interceptor.MyIntercepter02"/> </mvc:interceptor> </mvc:interceptors>