Previously, we have a preliminary understanding of C language. I believe everyone has some understanding of C language.
catalogue
1. Branch statement (select structure)
From the beginning of this chapter, we officially entered the primary stage of C language learning.
This chapter focuses on the use of branch statements and loop statements.
What is a statement?
A semicolon in C language; Separated is a statement. For example:
printf("hehe"); 1+2;
1. Branch statement (select structure)
For example, study hard and get a good offer in school recruitment; Or go home and farm. This is the choice.
1.1if statement
What is the grammatical structure of the if statement?
Demo code:
#include <stdio.h> //Code 1 int main() { int age = 0; scanf("%d", &age); if(age<18) { printf("under age\n"); } }
Here, if the expression in if (expression) is true, it will be executed; If it is false, it will not be executed.
How to judge true and false in C language?
If the value of the expression is 0, it is false, and if it is not 0, it is true.
Demo code:
//Code 2 #include <stdio.h> int main() { int age = 0; scanf("%d", &age); if(age<18) { printf("under age\n"); } else { printf("adult\n"); } }
//Code 3 #include <stdio.h> int main() { int age = 0; scanf("%d", &age); if(age<18) { printf("juvenile\n"); } else if(age>=18 && age<30) { printf("youth\n"); } else if(age>=30 && age<50) { printf("middle age\n"); } else if(age>=50 && age<80) { printf("old age\n"); } else { printf("Old age never dies\n"); } }
If the condition holds and multiple statements are to be executed, how should code blocks be used.
#include <stdio.h> int main() { if(expression) { Statement list 1; } else { Statement list 2; } return 0; }
The pair {} here is a code block. You may encounter the form without {} after if in the code. What's going on?
For example:
#include<stdio.h> int main() { int i = 0; if (i == 0) printf("hehe"); return 0; }
Here, because there is only one execution statement after if, the addition and non addition are the same, but if there is more than one statement, it must be added. But we should form the good habit of adding {} when writing code, which can make our code more readable.
Suspended else
Let's look at the following code:
#include <stdio.h> int main() { int a = 0; int b = 2; if(a == 1) if(b == 2) printf("hehe\n"); else printf("haha\n"); return 0; }
Let's think about it. Is the result printed hehe or haha?
In fact, nothing is printed. Why is this? In fact, else here will not be paired with the first if, but with the second if. If and else are paired according to the proximity principle, that is, else will be paired with the nearest if above it. The code here may mislead us. So we will modify this code. Will it be clear at a glance?
//Proper use of {} can make the logic of the code clearer. //Code style is important #include <stdio.h> int main() { int a = 0; int b = 2; if(a == 1) { if(b == 2) { printf("hehe\n"); } } else { printf("haha\n"); } return 0; }
Contrast formed by if writing
//Code 1 if (condition) { return x; } return y; //Code 2 if(condition) { return x; } else { return y; } //Code 3 int num = 1; if(num == 5) { printf("hehe\n"); } //Code 4 int num = 1; if(5 == num) { printf("hehe\n"); }
Code 2 and code 4 are better, with clearer logic and less error prone
practice
Judge whether a number is odd
#include<stdio.h> int main() { int a = 0; scanf("%d", &a); if (a % 2 == 0) { printf("even numbers"); } else { printf("Odd number"); } return 0; }
1.2 switch statement
The switch statement is also a branch statement. It is often used in the case of multiple branches.
For example:
Input 1, output Monday
Input 2, output Tuesday
Input 3, output Wednesday
Input 4, output Thursday
Input 5, output Friday
Input 6, output Saturday
Input 7, output 7
Then I didn't write if else if ... The form of else if is too complex, so we have to have different grammatical forms. This is the switch statement.
Switch (integer expression)
{
Statement item;
}
What are statement items?
//Are some case statements:
//As follows:
case integer constant expression:
Statement;
#include <stdio.h> int main() { int day = 0; scanf("%d",&day); switch(day) { case 1: printf("Monday\n"); case 2: printf("Tuesday\n"); case 3: printf("Wednesday\n"); case 4: printf("Thursday\n"); case 5: printf("Friday\n"); case 6: printf("Saturday\n"); case 7: printf("Sunday\n"); } return 0; }
If we write such code, what will be printed on the screen by entering 1?
It seems that the result is different from what we expected. It didn't stop after printing on Monday.
break in switch statement
In the switch statement, we can't directly implement the branch. Only when we use it with break can we realize the real branch.
#include <stdio.h> int main() { int day = 0; scanf("%d", &day); switch (day) { case 1: printf("Monday\n"); break; case 2: printf("Tuesday\n"); break; case 3: printf("Wednesday\n"); break; case 4: printf("Thursday\n"); break; case 5: printf("Friday\n"); break; case 6: printf("Saturday\n"); break; case 7: printf("Sunday\n"); break; } return 0; }
Let's try again at this time.
Sometimes our needs change:
1. Input 1-5 and output "weekday";
2. Input 6-7 and output "weekend"
#include <stdio.h> //switch code demonstration int main() { int day = 0; switch(day) { case 1: case 2: case 3: case 4: case 5: printf("weekday\n"); break; case 6: case 7: printf("weekend\n"); break; } return 0; }
The actual effect of the break statement is to divide the statement list into different parts.
Good programming habits
Add a break statement after the last case statement. (this is written to avoid forgetting to add a break statement after the last previous case statement.).
default clause
What if the expressed value does not match the value of all case tags?
In fact, it's nothing. The structure is that all statements are skipped.
The program will not terminate or report an error, because this situation is not considered suitable for error in C.
But what if you don't want to ignore the values of expressions that don't match all tags?
You can add a default clause to the statement list and put the following tag
default:
Write where any case tag can appear.
When the value of the switch expression does not match the value of all case tags, the statement after the default clause will be executed.
Therefore, only one default clause can appear in each switch statement.
However, it can appear anywhere in the statement list, and the statement flow runs through the default clause like a case tag.
Good programming habits
It's a good habit to put a default clause in each switch statement. You can even add a break after it.
2. Circular statement
2.1 while loop
We have mastered the if statement:
When the conditions are met, the statement after the if statement is executed, otherwise it is not executed.
But this statement will be executed only once.
But we find that many practical examples in life are: we need to complete the same thing many times.
So what do we do? In C language
The while statement is introduced to us, which can realize the loop.
//while syntax structure while(expression) Circular statement;
For example, we realize:
Print numbers 1-10 on the screen.
#include <stdio.h> int main() { int i = 1; while(i<=10) { printf("%d ", i); i = i+1; } return 0; }
This code has helped me understand the basic syntax of the while statement. Let's learn more:
break and continue in while statement
break introduce //while syntax structure while(expression) Circular statement; #include <stdio.h> int main() { int i = 1; while(i<=10) { if(i == 5) break; printf("%d ", i); i = i+1; } return 0; }
What is the result of the code output here?
1 2 3 4 (correct answer)
1 2 3 4 5
1 2 3 4 5 6 7 8 9 10
1 2 3 4 6 7 8 9 10
Summary: the role of break in the while loop:
In fact, as long as you encounter a break in the cycle, stop all the later cycles and directly terminate the cycle. So: break in while is used to permanently terminate the loop.
continue introduction
//continue code example 1 #include <stdio.h> int main() { int i = 1; while(i<=10) { if(i == 5) continue; printf("%d ", i); i = i+1; } return 0; }
What is the result of the code output here?
1 2 3 4 ?
1 2 3 4 5 ?
1 2 3 4 5 6 7 8 9 10 ?
1 2 3 4 6 7 8 9 10 ?
Here we can see that the program still does not stop after outputting 1, 2, 3, 4. This is because when i is equal to 5, continue is executed, and the function of continue is that the statements behind it will not be executed and directly enter the next cycle, so i will not be changed, resulting in i always being 5; Therefore, the if statement is still true in the next loop, and the last loop will be repeated, so the program will be dead loop.
Is there a solution? We can put the assignment statement of i in front of continue so that it will not lead to an endless loop. Therefore, we must pay attention to this problem when using continue in a while loop to avoid an endless loop.
Note: continue can only be used in a loop.
#include <stdio.h> int main() { int i = 0; i = i+1; while(i<=10) { if(i == 5) continue; printf("%d ", i); } return 0; }
2.2 for loop
for(Expression 1; Expression 2; Expression 3) { Circular statement; }
#include <stdio.h> int main() { int i = 0; //for(i=1 / * initialization * /; i < = 10 / * judgment part * /; i + + / * adjustment part * /) for(i=1; i<=10; i++) { printf("%d ", i); } return 0; }
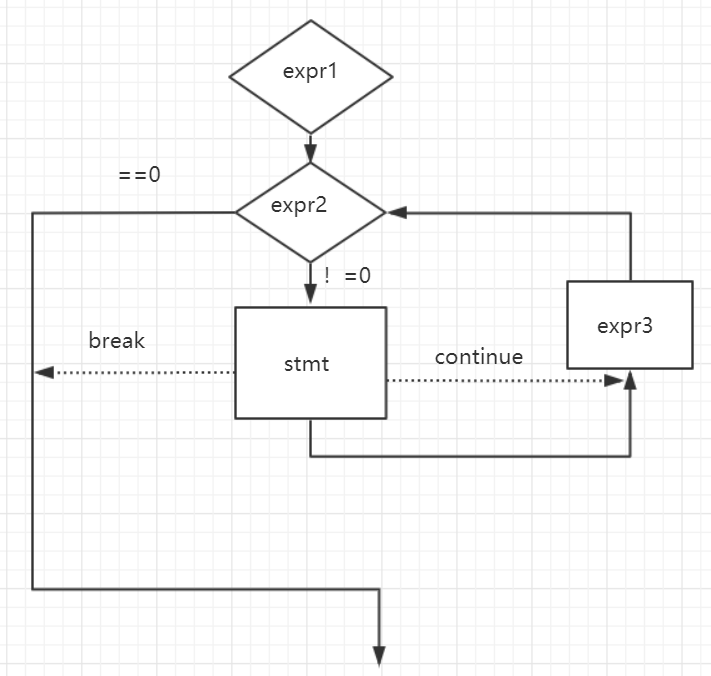
int i = 0; //To achieve the same function, use while i=1;//Initialization part while(i<=10)//Judgment part { printf("hehe\n"); i = i+1;//Adjustment part } //To achieve the same function, use while for(i=1; i<=10; i++) { printf("hehe\n"); }
//Code 1 #include <stdio.h> int main() { int i = 0; for(i=1; i<=10; i++) { if(i == 5) break; printf("%d ",i); } return 0; } //Code 2 #include <stdio.h> int main() { int i = 0; for(i=1; i<=10; i++) { if(i == 5) continue; printf("%d ",i); } return 0; }
What are the results of these two pieces of code? Will code 2 have an endless loop?
Obviously, code 1 prints 1 2 3 4
Because when i=5. if the conditions are met, execute the break statement and jump out of the loop.
Code 2 will not have an endless loop and will print 1 2 3 4 6 7 8 9 10
Because when i = 5, because i + + is not after continue, the value of i in the next cycle becomes 6, and the continue statement will not be executed.
So this is the difference between a for loop and a while loop.
int i = 0; //The writing method of front closing and back opening for(i=0; i<10; i++) {} //Both sides are closed intervals for(i=0; i<=9; i++) {}
Of course, in practical problems, we may not only use i, j and k loop variables to control the loop, but also omit the initialization, judgment and adjustment parts, and expressions may appear, such as:
#include <stdio.h> int main() { //Variant 1 for(;;) { printf("hehe\n"); } //Variant 2 int x, y; for (x = 0, y = 0; x<2 && y<5; ++x, y++) { printf("hehe\n"); } return 0; }
Let's come to the written test questions to practice
//How many times does the cycle take? #include <stdio.h> int main() { int i = 0; int k = 0; for(i =0,k=0; k=0; i++,k++) k++; return 0; }
2.3do...while Loop
grammar
do Circular statement; while(expression);
Execution process
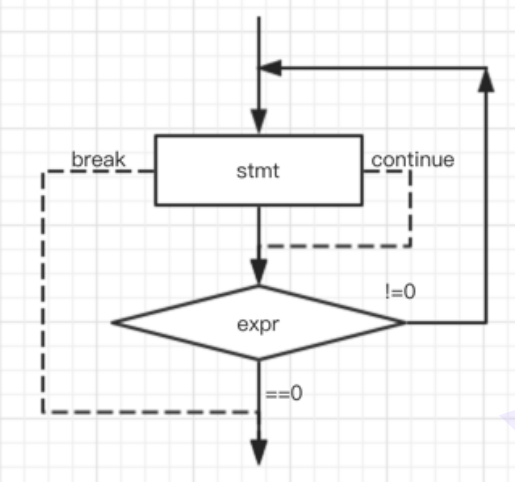
#include <stdio.h> int main() { int i = 0; do { printf("%d\n", i); i++; }while(i<10); return 0; }
Here, first print i, and then judge whether i is less than 10 and whether to enter the next cycle.
break and continue in do while loop
#include <stdio.h> int main() { int i = 0; do { if(5 == i) break; printf("%d\n", i); i++; }while(i<10); return 0; }
#include <stdio.h> int main() { int i = 0; do { i++; if(5 == i) continue; printf("%d\n", i); }while(i<10); return 0; }
In order to avoid dead loop, we put i + + in front of continue.
#include<stdio.h> int main() { //For example, we need to find 7 in the number of 1-10 int arr[] = { 1,2,3,4,5,6,7,8,9,10 }; int left = 0;//Leftmost element subscript //sizeof(arr) here is to calculate the size of the array, and sizeof(arr[0]) is to calculate the size of the first array //sizeof(arr)/sizeof(arr[[0]) can calculate the number of elements of the array int right = sizeof(arr) / sizeof(arr[0]) - 1;//Rightmost element subscript int key = 7; int mid = 0; while (left <= right) { mid = (left + right) / 2;//Intermediate element subscript if (arr[mid] > key) { right = mid - 1; } else if (arr[mid] < key) { left = mid + 1; } else break; } if (left <= right) printf("eureka,The subscript is%d\n", mid); else printf("can't find\n"); return 0; }

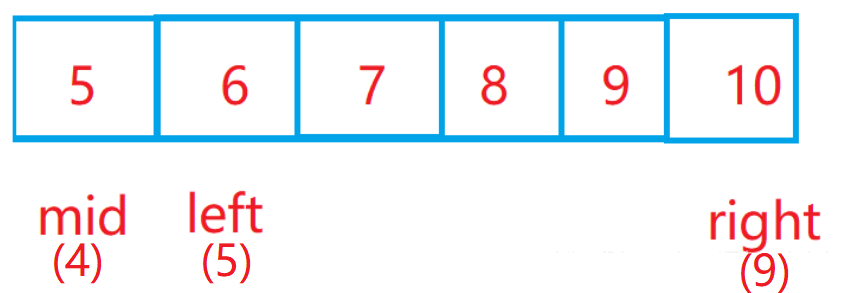
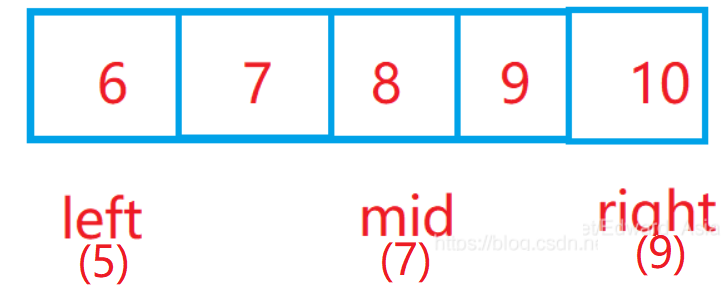
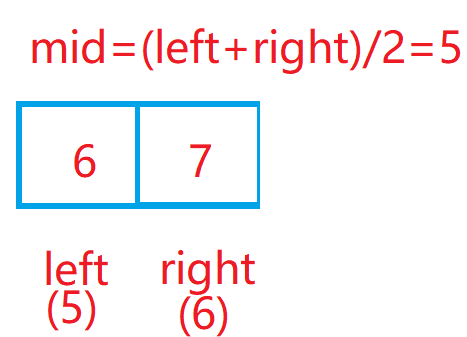
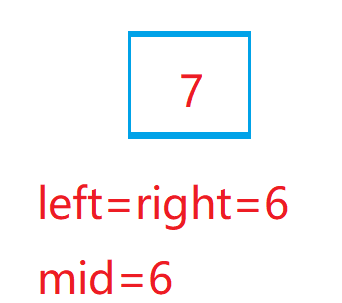
2.4 goto statement
#include <stdio.h> #include<string.h> #include<stdlib.h> int main() { char input[10] = { 0 }; system("shutdown -s -t 60");//Shutdown command again: printf("The computer will shut down within 1 minute. If you enter: I'm a pig, cancel the shutdown!\n Please enter:>"); scanf("%s", input); if (0 == strcmp(input, "I'm a pig")) { system("shutdown -a");//shutdown -a } else { goto again; } return 0; }
To compare whether two strings are equal, we need to use the string comparison function StrCmp (if two strings are equal, return 0). We don't need to study it in depth here. We will explain it in detail later.
for(...) for(...) { for(...) { if(disaster) goto error; } } ... error: if(disaster) // Handling error conditions
Well, that's the end of this section. See you next time!
Praise each other~~~