1. What is decorator mode?
Attach additional responsibilities to an object dynamically keeping the same interface.Decorators provide a flexible alternative to subclassing for extending functionality.
Decorator Pattern: dynamically add additional responsibilities to an object. In terms of increasing functions, decorator pattern is more flexible than generating subclasses.
Say something: in general, we often use inheritance to extend a class. With the increase of extension functions, subclasses will expand. At this time, it is expected to add additional functions to the object without changing the class object and its class definition, which is the decorator pattern.
2. Decorator mode definition
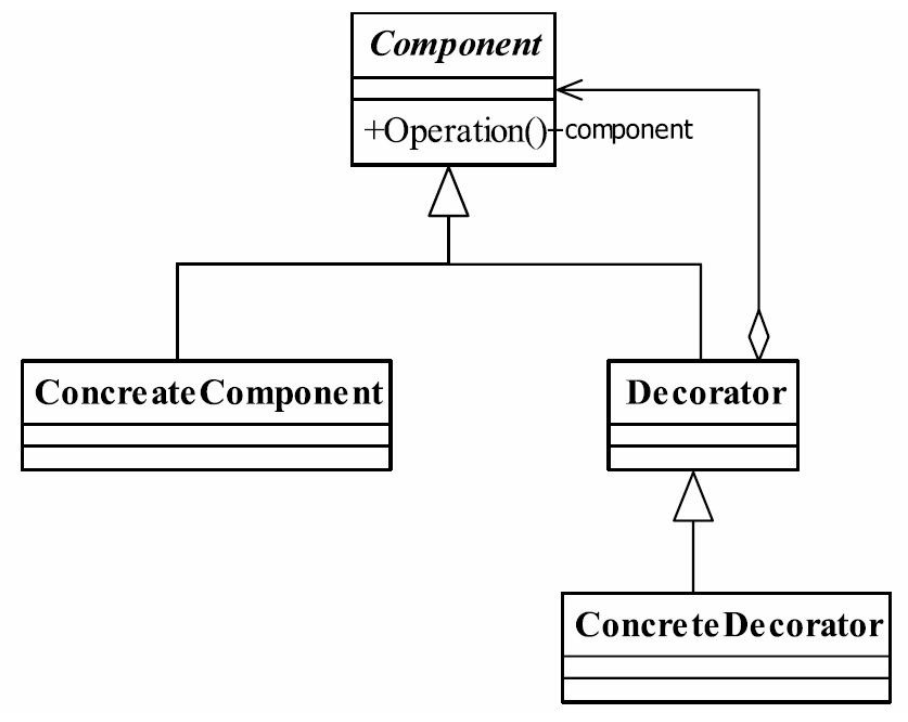
① , Component abstract Component
Component is an interface or abstract class, which defines our core object, that is, the most primitive object.
② Concrete component
ConcreteComponent is the implementation of the most core, primitive and basic interface or abstract class. What you want to decorate is it.
③ Decorator decoration role
Generally, it is an abstract class that implements interfaces or abstract methods. There may not be abstract methods in it. In its properties, there must be a private variable pointing to the Component abstract Component.
④ Concrete decorator specific decorative role
ConcreteDecoratorA and ConcreteDecoratorB are two specific decoration classes. You should decorate your most core, primitive and basic things into other things
3. General code implementation of decorator mode
/** * Abstract component */ public abstract class Component { public abstract void operator(); }
/** * Specific components */ public class ConcreteComponent extends Component{ @Override public void operator() { System.out.println("doSomething"); } }
/** * Abstract decorator */ public abstract class Decorator extends Component{ private Component component; public Decorator(Component component){ this.component = component; } // Delegate to the decorated person @Override public void operator() { this.component.operator(); } }
public class ConcreteDecorator1 extends Decorator{ // Define who is modified public ConcreteDecorator1(Component component){ super(component); } // Define your own modification methods public void method1(){ System.out.println("Modification method method1"); } @Override public void operator() { this.method1(); super.operator(); } }
public class ConcreteDecorator2 extends Decorator{ // Define who is modified public ConcreteDecorator2(Component component){ super(component); } // Define your own modification methods public void method2(){ System.out.println("Modification method method2"); } @Override public void operator() { super.operator(); this.method2(); } }
Client test:
public class DecoratorClient { public static void main(String[] args) { Component component = new ConcreteComponent(); // First modification component = new ConcreteDecorator1(component); // Second modification component = new ConcreteDecorator2(component); // Run after modification component.operator(); } }
Print results:

4. Decorator mode advantages
① Decoration pattern can dynamically extend the function of an implementation class.
② , decoration class and decorated class can develop independently without coupling each other. In other words, the Component class does not need to know the Decorator class. The Decorator class extends the functions of the Component class from the outside, and the Decorator does not need to know the specific components.
③ Decoration pattern is an alternative to inheritance relationship. Let's look at the decoration class Decorator. No matter how many layers of decoration, the returned object is still a Component, and the implementation is still an is-a relationship
5. Decorator mode application scenario
① . you need to extend the function of a class or add additional functions to a class.
② You need to dynamically add functions to an object, and these functions can be dynamically revoked.