Recently, I have summarized 9 good Python tools commonly used, which can greatly improve our work efficiency, install them, and then gradually use them skillfully. If useful, you can collect this article.
1 Faker generates false data
If you are still worried about generating names, addresses and IP addresses, try the Faker library.
It is a professional artifact to generate false data, but the generated data looks so "not false".
The basic usage is as follows:
from faker import Faker fake = Faker(locale="zh_CN") fake.name() # 'tan Liu ' fake.address() # '630814, block Y, Zhao street, Xincheng, Guanling County, Jiangxi Province' fake.text() 'How the author area.Development source of corporate content responsibility in Investment Report.Take a look at yourself and the whole community at once.\n Thank you very much today.Among them, everyone organizes games.\n Only the latest control plans the school at the same time.Analyze the reporter's price mode.\n Possible ways to feel successful include mobile phones.China is important. This comparison must center on mine.\n There are many security categories in the company, one of which is prestige.Title Department National Document Japanese post points are different.\n The sales history of this project can.For the feeling that the whole country takes place, it's not at home.Unit user news becomes.Leave a message on this site to explain that the report work continues.'
2 Pendulum management time
Using date and time formats is never fun.
Although the built-in datetime module does quite well, there is a more intuitive Pendulum, which can process quickly.
It supports time zone conversion, date, time operations, and formatting.
The following is a quick example to quickly create a time zone in Shanghai:
from datetime import datetime import pendulum sh = pendulum.timezone('Asia/Shanghai') shc = pendulum.now() print('Current Date Time in sh =', shc) # DateTime(2021, 12, 9, 16, 38, 52, 599942, tzinfo=Timezone('Asia/Shanghai')) shc.add(years=1) # DateTime(2022, 12, 9, 16, 38, 52, 599942, tzinfo=Timezone('Asia/Shanghai'))
3 Scrapy is a reptile
Scrapy is a powerful tool that allows you to quickly extract information from websites.
When a large amount of information needs to be extracted from multiple websites or web pages, manual extraction is inefficient.
scrapy provides easy-to-use methods and packages that can extract information using HTML tags or CSS classes. Install the script with the following command:
pip install scrapy
Then input the following line of code directly at the terminal,
scrapy fetch --nolog https://baidu.com
You can get the html content of Baidu's home page.
4 using Pandas data analysis
Pandas is a simple but powerful data analysis tool. It can be used for data cleaning and statistical analysis.
After analyzing the data, you can also visualize it using external libraries such as [Matplotlib]( https://github.com/matplotlib/matplotlib).
The best thing about Pandas is that it is built on NumPy. NumPy is a powerful data analysis tool. Because Pandas is based on it, it means that most NumPy methods are existing functions in Pandas.
5 click command line tool
click is a Python package that can be used to create a command line interface. It's a pretty command line and very smooth.
Let's take an example:
""" click Module demonstration """ @click.command() @click.option('--count', default=3) @click.option('--name', prompt='Enter your name:') def hello(count, name): for x in range(count): print(f"Hello {name}!") if __name__ == "__main__": hello()
The hello function exposes two parameters: count and name. Finally, on the command line, call the script directly as follows:
python click_hello.py --count=5
Last print:
Enter your name:: zhenguo Hello zhenguo! Hello zhenguo! Hello zhenguo! Hello zhenguo! Hello zhenguo!
6 micro web framework Flask
Do you need to set up a web server?
Do you have two seconds? Because this is the time required to start a simple web server in Python, go directly to the following line of code:
python -m http.server 8000
But for a basic web application, this may be too simple. Flask is a micro web framework built in Python. It is "micro" because it does not have any database abstraction layer, form validation, or mail support.
Fortunately, it has a lot of extensions that can be plug and play. If you only want to provide a simple API, it is perfect.
To create an API server using flash, use the following script:
from flask import Flask from flask import jsonify app = Flask(__name__) @app.route('/') def root(): return jsonify( app_name="zhenguo New gadgets", app_user="zhenguo" )
Start the service with the following line of code:
FLASK_APP=flask.py flask run
Finally, when you access the URL in the browser http://127.0.0.1:5000/ When,
You should see the following JSON:
{"app_name":"zhenguo New gadgets","app_user":"zhenguo"}
7 API Requests
Requests is a powerful HTTP library. With it, you can automate any operation related to HTTP requests, including API automation calls, so that you don't have to call them manually.
It comes with some useful features, such as authorization processing, JSON/XML parsing, and session processing.
Get the plaintext address as follows: No. 35, Qinghua East Road, Haidian District, Beijing. For the corresponding longitude and latitude, use Baidu map interface to register for free to get an apk. The returned longitude and latitude results are as follows:
import requests import re rep = requests.get( 'https://api.map.baidu.com/geocoding/v3/?address = 35 Qinghua East Road, Haidian District, Beijing & output = JSON & AK = your APK & callback = showlocation ') print(re.findall(r'"lng":(.*),"lat":(.*?)}', rep.text)) # The results show that [('116.35194130702107', '40.00664192889596')]
8 automated testing Selenium
Selenium is a testing framework for writing automated test cases.
Although it is written in Java, the Python package provides access to similar API s for almost all Selenium functions.
Selenium is usually used to automate the testing of application UI, but you can also use it to automate tasks on the machine, such as opening a browser, dragging and dropping files, and so on.
Take a quick example to demonstrate how to open the browser and visit Baidu's home page:
from selenium import webdriver import time browser = webdriver.Chrome(executable_path ="C:\Program Files (x86)\Google\Chrome\chromedriver.exe") website_URL ="https://baidu.com/" brower.get(website_URL) refreshrate = int(15) # Keep running while True: time.sleep(refreshrate) browser.refresh()
Now, the script refreshes the baidu home page in the browser every 15 seconds.
9 image processing pilot
Many times, you need to modify the image in some way to make it more suitable, such as blurring details, combining one or more images, or creating thumbnails.
Combining homemade pilot scripts with Click and then accessing them directly from the command line is very useful for speeding up repetitive image processing tasks.
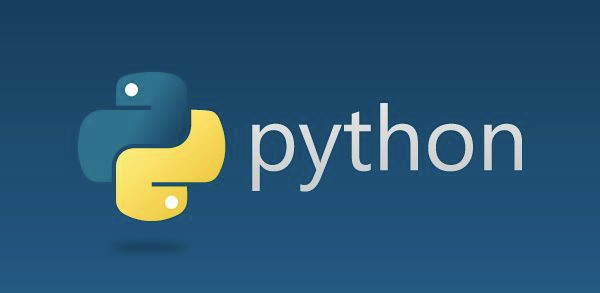
Look at a quick example of a blurred image:
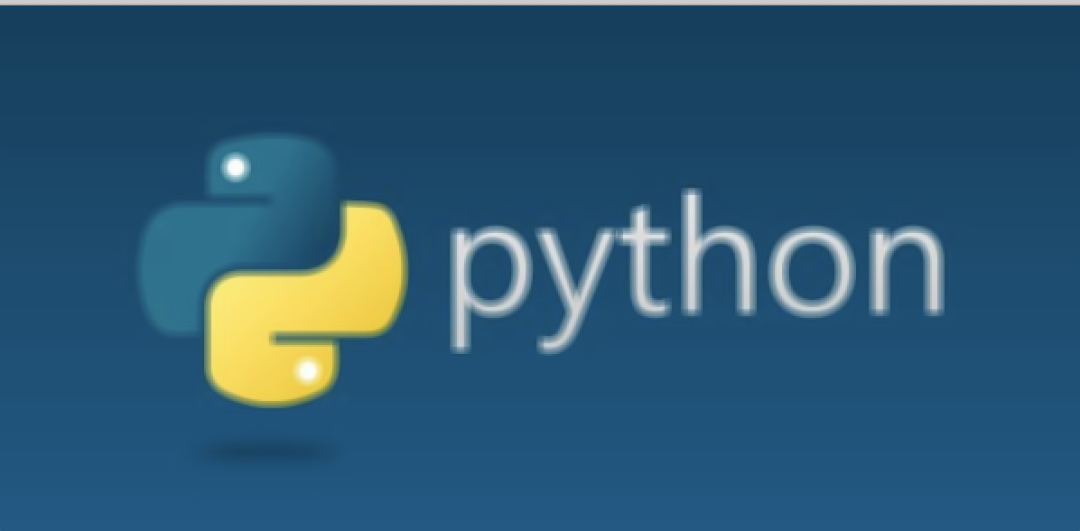
from PIL import Image, ImageFilter try: original = Image.open("python-logo.png") # Blur the image blurred = original.filter(ImageFilter.BLUR) # Display both images original.show() blurred.show() blurred.save("blurred.png") except: print('Failed to load image')