1. Introduction
There is a timer requirement for the project, as shown in the figure below. It was supposed to be quite simple, but it actually involves the time zone.
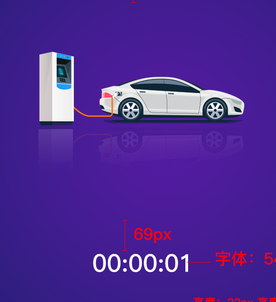
The renderings are as follows
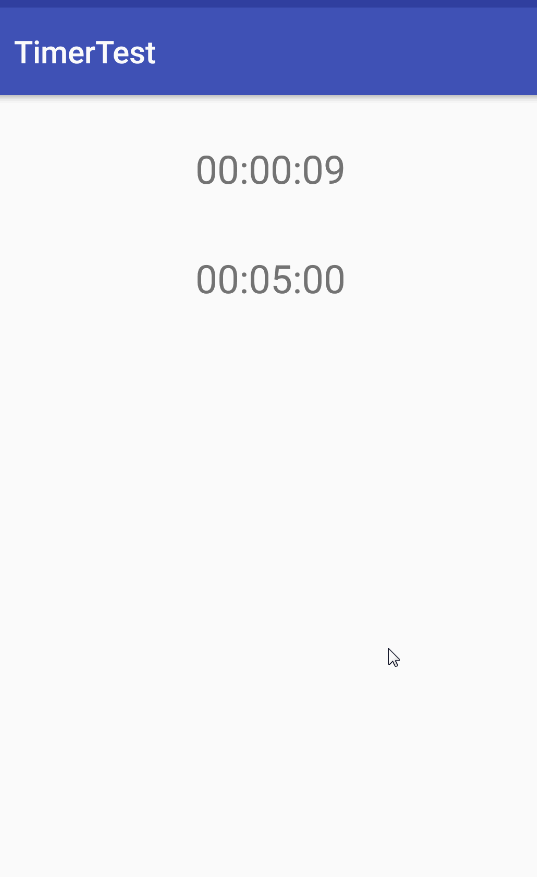
2. Solution
The key code is relatively simple
- Milliseconds to HH:ss:mm
- Refresh and change the time on the control regularly
The timing change time here is refreshed to the control at the same time. I use the timer, timerTask and Handler implementation, which belongs to the knowledge of timing to do something, not the key point here, directly to the code
timerTask = new TimerTask() {
@Override
public void run() {
i++;
Message message = handler.obtainMessage();
message.what = mwhat;
message.obj = i;
handler.sendMessage(message);
}
};
timer = new Timer();
timer.schedule(timerTask,1000,1000);
private Handler handler = new Handler(){
@Override
public void handleMessage(Message msg) {
switch(msg.what){
case mwhat:
long second = (int) msg.obj*1000;
tv.setText(getStringByFormat(second,dateFormatHMS));
break;
}
}
};
Next, we will focus on how to convert the above MS value into the time, minute and second string. Of course, here we have the tool class
/**
* Description: gets the string of date and time represented by milliseconds
* @param format Format the string, such as "yyyy MM DD HH: mm: SS"
* @return String Date time string
*/
public static String getStringByFormat(long milliseconds, String format) {
String thisDateTime = null;
try {
SimpleDateFormat mSimpleDateFormat = new SimpleDateFormat(format);
thisDateTime = mSimpleDateFormat.format(milliseconds);
} catch (Exception e) {
e.printStackTrace();
}
return thisDateTime;
}
/**
* Hour minute second
*/
public static String dateFormatHMS = "HH:mm:ss";
Then run it to get the following results
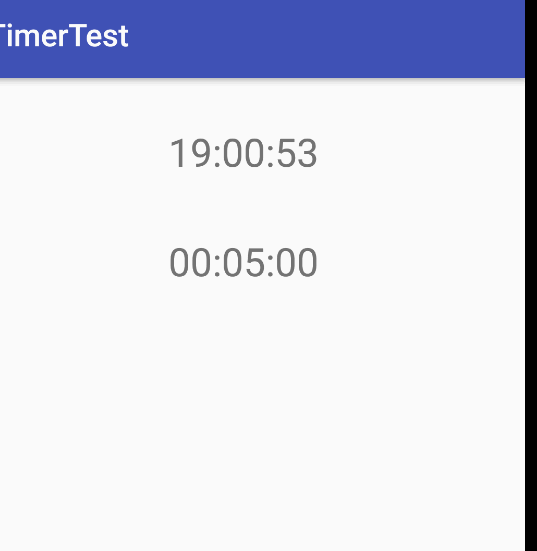
Did you find out? It's 19 in an hour. It should be 00 in principle. This is the effect of running on the simulator. Then I run it on my mobile phone. The result is
08: 00: 55
It's so strange. Youmuyou found later that the time zone of the operating system on my mobile phone is the U.S. zone, while the real time on my mobile phone is the Chinese standard time, Beijing time.
We want to set the time zone for the above SimpleDateFormat because we want to ask for the GMT time and the real machine gives us GMT+8
Modify the code as follows
/**
* Description: gets the string of date and time represented by milliseconds
* @param format Format the string, such as "yyyy MM DD HH: mm: SS"
* @return String Date time string
*/
public static String getStringByFormat(long milliseconds, String format) {
String thisDateTime = null;
try {
SimpleDateFormat mSimpleDateFormat = new SimpleDateFormat(format);
mSimpleDateFormat.setTimeZone(TimeZone.getTimeZone("GMT"));
thisDateTime = mSimpleDateFormat.format(milliseconds);
} catch (Exception e) {
e.printStackTrace();
}
return thisDateTime;
}
Problem solving, thank you
Notes on java processing time zone