Hello, I'm an advanced Python player.
preface
"Cheat the Golden Flower", also known as three cards, is a folk multiplayer card game widely spread throughout the country. For example, the cheating golden flower in JJ competition (winning three cards) has unique card comparison rules. The game needs to test the player's courage and wisdom-- A few days ago, in the python exchange group of Baidu Encyclopedia, a fan named [^ - ^] shared the topic of cheating gold flowers with playing cards, which is required to be implemented in Python. The topics are as follows:
Win and lose a program. #### Rules of the game: A set of playing cards, remove the king of size, and each player will deal 3 cards. Finally, compare the size to see who wins. There are the following cards: Leopard: three identical cards, such as three six. Shunjin: also known as flush, that is, three shunzi of the same design and color, such as hearts 5, 6 and 7 Shunzi: also known as tractor, it has different designs and colors, but shunzi, such as 5 hearts, 6 square pieces and 7 spades, is composed of shunzi Pair: two cards are the same Leaflet: what is the biggest single A The order of size of these cards is, **leopard>Shun Jin>Shunzi>Pair>Leaflet** #### Points to be realized by the program: 1. Sir, a complete set of playing cards 2. Deal cards to 5 players at random 3. Who is the winner of unified card opening, size comparison and output
1, Train of thought
To solve this problem, we first need to construct a pair of playing cards, disassemble them continuously according to the attribute characteristics of playing cards, and then construct player users. Dictionaries and lists are often used here to store information, which has been tried and tested repeatedly.
2, Solution
To solve this problem, fan [^ - ^] gave a solution. The direct code is as follows:
# -*- coding: utf-8 -*- import random puke = [] # Store playing cards num_list = ['2', '3', '4', '5', '6', '7', '8', '9', '10', 'J', 'Q', 'K', 'A'] hua_list = ['Plum blossom', 'heart', 'spade', 'block'] sort_dic = {'2': 0, '3': 1, '4': 2, '5': 3, '6': 4, '7': 5, '8': 6, '9': 7, '10': 8, 'J': 9, 'Q': 10, 'K': 11, 'A': 12, 'Pair': 15, 'Shunzi': 30, 'Shun Jin': 60, 'leopard': 100} count_new_list = [] # Store player scores and ranking after sorting count_dic = {} # Store player scores # Prepare 52 cards for hua in hua_list: for num in num_list: a = hua + num puke.append(a) player_dic = {'Player 1': [], 'Player 2': [], 'Player 3': [], 'Player 4': [], 'Player 5': []} # Deal cards to five players at random # print(len(puke)) for key, value in player_dic.items(): for i in range(3): plate = random.sample(puke, 3) player_dic[key] = plate for i in plate: puke.remove(i) print(player_dic) # Get the player's card type def paixing(list1): num = [] huase = [] for i in list1: a = i[2:] b = i[:2] num.append(a) huase.append(b) return num, huase # sort_dic = {'2': 0, '3': 1, '4': 2, '5': 3, '6': 4} # Sort the card types of numbers def sort(num): new_num = [] sort_list2 = [] list1 = [] for i in num: new_num.append(sort_dic[i]) new_num = sorted(new_num) # After sorting is [2, 4, 7] for new in new_num: sort_list2.append([k for k, v in sort_dic.items() if v == new]) for m in sort_list2: for n in m: list1.append(n) return list1 # Count the score of the player's card shape def count(num, huase): a = 0 base_count = sort_dic[num[0]] + sort_dic[num[1]] + sort_dic[num[2]] if num[0] == num[1] and num[1] == num[2]: paixing = 'leopard' a = base_count + sort_dic[paixing] elif (sort_dic[num[0]] + 1 == sort_dic[num[1]] and sort_dic[num[2]] - 1 == sort_dic[num[1]]) and (huase[0] == huase[ 1] and huase[1] == huase[2]): paixing = 'Shun Jin' a = base_count + sort_dic[paixing] elif (sort_dic[num[0]] + 1 == sort_dic[num[1]]) and (sort_dic[num[2]] - 1 == sort_dic[num[1]]) and ( huase[0] != huase[ 1] or huase[1] != huase[2]): paixing = 'Shunzi' a = base_count + sort_dic[paixing] elif (num[0] == num[1] and num[1] != num[2]) or (num[1] == num[2] and num[0] != num[1]) or ( num[0] == num[2] and num[1] != num[0]): paixing = 'Pair' a = base_count + sort_dic[paixing] else: a = base_count return a # Sort the dictionaries that store player scores def compare(count_dic): d = list(zip(count_dic.values(), count_dic.keys())) return sorted(d, reverse=True) for key, value in player_dic.items(): num, huase = paixing(value) num = sort(num) count1 = count(num, huase) count_dic[key] = count1 print(key + "The cards are:" + str(value)) count_new_list = compare(count_dic) # print(count_new_list) print('Final ranking:' + "\t" + count_new_list[0][1] + "the first" + "\t" + count_new_list[1][1] + "proxime accessit" + "\t" + count_new_list[2][ 1] + "third" + "\t" + count_new_list[3][1] + "Fourth place" + "\t" + count_new_list[4][1] + "Fifth place")
The code does look quite large, more than 100 lines, and it takes some time to read. However, the knowledge involved is not complex. It is basically a little Python based and understandable. After running the code, you can see the following results:
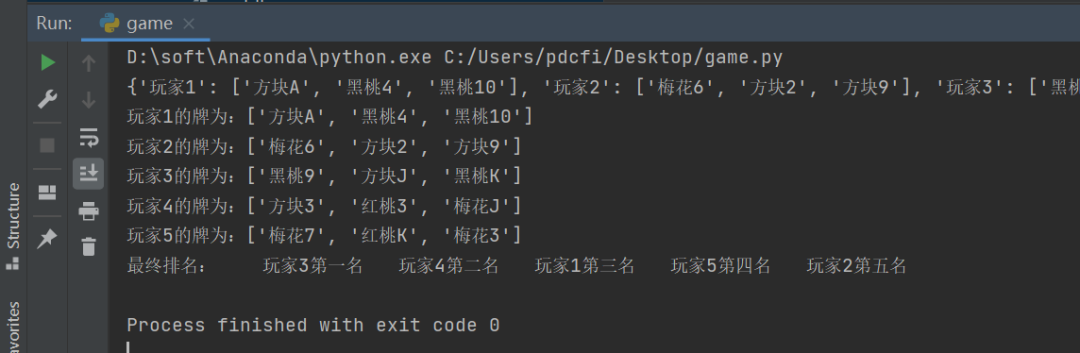
However, when I read the code later, I found that there was some redundancy in the middle. With a little modification, the code was simpler. Some functions and variables were named with some corresponding words of practical significance, which made it more readable. The code is as follows:
# -*- coding: utf-8 -*- import random puke = [] # Store playing cards num_list = ['2', '3', '4', '5', '6', '7', '8', '9', '10', 'J', 'Q', 'K', 'A'] hua_list = ['Plum blossom', 'heart', 'spade', 'block'] sort_dic = {'2': 0, '3': 1, '4': 2, '5': 3, '6': 4, '7': 5, '8': 6, '9': 7, '10': 8, 'J': 9, 'Q': 10, 'K': 11, 'A': 12, 'Pair': 15, 'Shunzi': 30, 'Shun Jin': 60, 'leopard': 100} count_new_list = [] # Store player scores and ranking after sorting count_dic = {} # Store player scores # Prepare 52 cards for hua in hua_list: for num in num_list: a = hua + num puke.append(a) player_dic = {'Player 1': [], 'Player 2': [], 'Player 3': [], 'Player 4': [], 'Player 5': []} # Deal cards to five players at random print(len(puke)) for key, value in player_dic.items(): for i in range(3): plate = random.sample(puke, 3) player_dic[key] = plate for i in plate: puke.remove(i) print(player_dic) # Get the player's card type def paixing(list1): num = [] huase = [] for data in list1: huase_type = data[:2] pai_number = data[2:] num.append(pai_number) huase.append(huase_type) return num, huase # Count the score of the player's card shape def get_score(num, huase): base_count = sort_dic[num[0]] + sort_dic[num[1]] + sort_dic[num[2]] if num[0] == num[1] and num[1] == num[2]: paixing = 'leopard' score = base_count + sort_dic[paixing] elif (sort_dic[num[0]] + 1 == sort_dic[num[1]] and sort_dic[num[2]] - 1 == sort_dic[num[1]]) and (huase[0] == huase[ 1] and huase[1] == huase[2]): paixing = 'Shun Jin' score = base_count + sort_dic[paixing] elif (sort_dic[num[0]] + 1 == sort_dic[num[1]]) and (sort_dic[num[2]] - 1 == sort_dic[num[1]]) and ( huase[0] != huase[ 1] or huase[1] != huase[2]): paixing = 'Shunzi' score = base_count + sort_dic[paixing] elif (num[0] == num[1] and num[1] != num[2]) or (num[1] == num[2] and num[0] != num[1]) or ( num[0] == num[2] and num[1] != num[0]): paixing = 'Pair' score = base_count + sort_dic[paixing] else: score = base_count return score if __name__ == '__main__': for key, value in player_dic.items(): num, huase = paixing(value) # Sort the card types of numbers num = sorted(num) score = get_score(num, huase) count_dic[key] = score print(key + "The cards are:" + str(value)) # Sort the dictionaries that store player scores count_new_list = sorted(zip(count_dic.values(), count_dic.keys()), reverse=True) print("Final ranking:") for i in range(len(count_new_list)): print(count_new_list[i][1] + '\t', end='')
3, Summary
I'm an advanced Python player. In this paper, the real-life gold fraud game is programmed based on python, and the process of online gold fraud is realized by using lists, dictionaries, functions and so on in the basic knowledge of Python.
Finally, thank fans [^ - ^] for sharing. Xiaobian believes that there must be other ways to solve this problem. You are also welcome to give advice in the comment area.
Guys, let's practice it!