Published in 2019-03-192020-03-03 author Ryan
At present, in the programmer market environment, the Java Party's lamda syntax is everywhere, such as the familiar Spring 5.x framework. lambda is flying all over the world!
Here's why we play with lambda and what's the difference between lambda and object-oriented.
1. The object-oriented programming method is that programmers hit the keyboard to tell the computer what to do, what to do first and then what to do; 2. lambda is a program to tell the programmer what to do first and what to do later; 3. The writing method of lambda can reduce the number of keystrokes and give you more time to pick up coffe e;
I won't explain the syntax and methods of lamda here. If you are interested, you can directly read the Book Java 8 practice, have a detailed description, or read the official documents;
Explain:
- windows 7
- oracle hotspot jdk 1.8
- InteliJ IDEA 2018.1.5
- Apache Maven 3.3.3
Let's start with an example. To use the lambda syntax in Java 8, we first need to ensure that our new project language level is above 8. As shown below
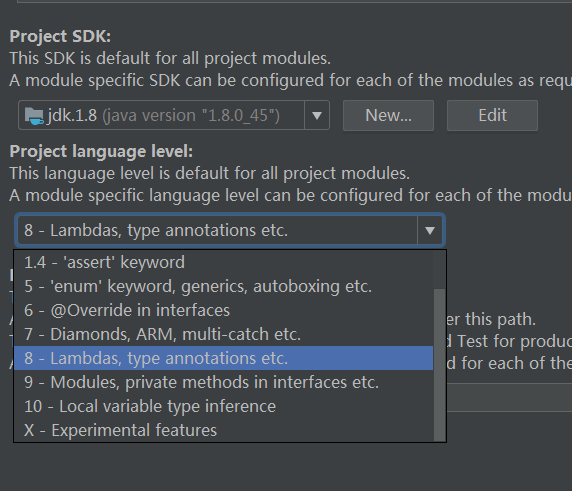
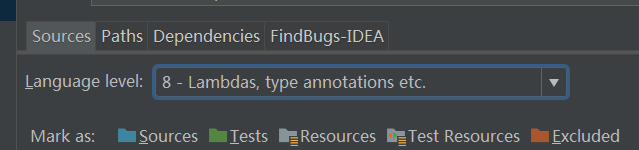
The above two items must be 8 +, otherwise the expected function and effect will not be achieved.
Next, create a Student sample class with the following code:
private static class Student{ private String id; private String name; private int age; private boolean graduation; public Student(String id, String name, int age, boolean graduation) { this.id = id; this.name = name; this.age = age; this.graduation = graduation; } public String getId() { return id; } public void setId(String id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public boolean isGraduation() { return graduation; } public void setGraduation(boolean graduation) { this.graduation = graduation; } @Override public String toString() { return "Student{" + "id='" + id + '\'' + ", name='" + name + '\'' + ", age=" + age + ", graduation=" + graduation + '}'; } }
Next, write our program main class, as shown in the following figure:
public static void main(String[] args) { List<String> data = new ArrayList<String>(); data.add("1,Ryan,25,true"); data.add("2,zhangsan,22,false"); data.add("3,lisi,18,false"); data.add("4,wangwu,35,true"); data.add("5,liliu,20,false"); Set<String> collect = data.stream().map(d -> { String[] split = d.split(","); return new Student(split[0], split[1], Integer.parseInt(split[2]), Boolean.parseBoolean(split[3])); }).filter(Student::isGraduation).map(Student::getName).collect(Collectors.toSet()); System.out.println(collect); }
To explain, this is mainly to analyze the data of students, filter out the graduated students, obtain their names, and finally output;
Here, we first need to debug Lambda and hit the breakpoint. An F8 may pass, and we can't see the result of. map(Student::getName). What should we do at this time? It is also possible to encounter an embarrassing thing like the author (not a sentence, so embarrassing!)
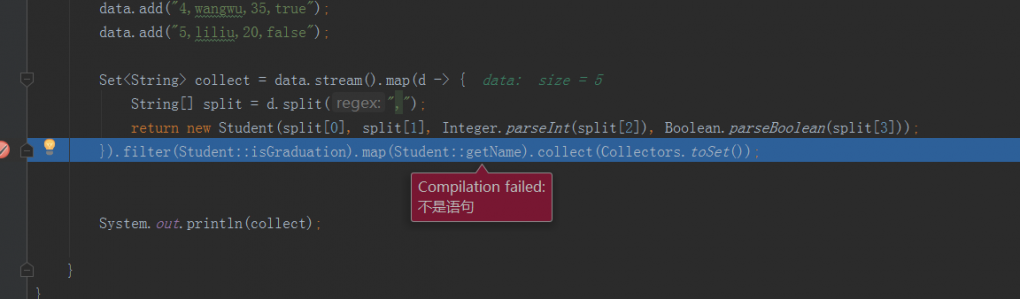
We need to debug lambda. IDEA provides us with a friendly way, that is, "Trace Current Stream Chain". This function is in the debug Tab, as shown in the following figure:
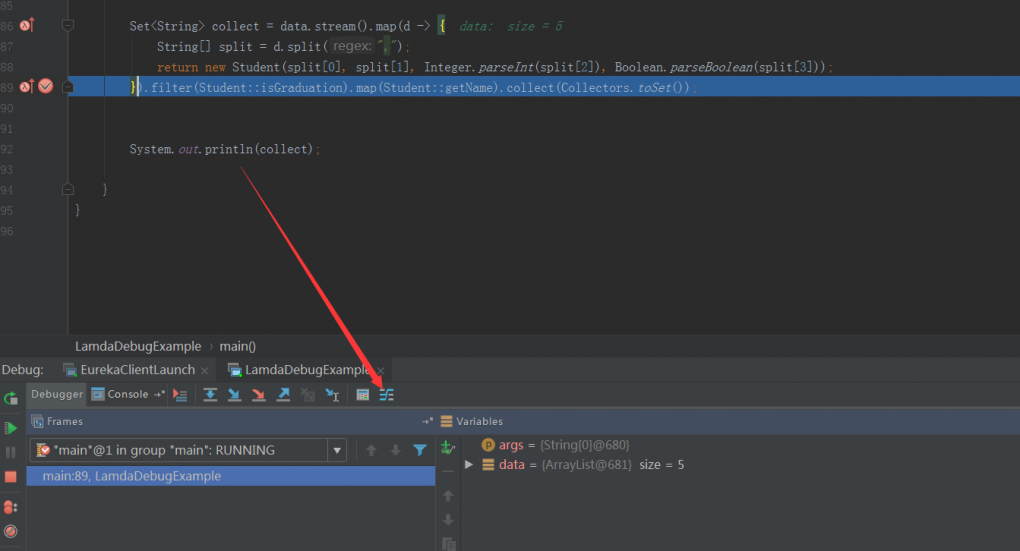
We can click it directly. If there is no such function under your IDEA, be sure to check your IDEA version. After clicking, you need to wait a little while, and a picture similar to the author will appear:
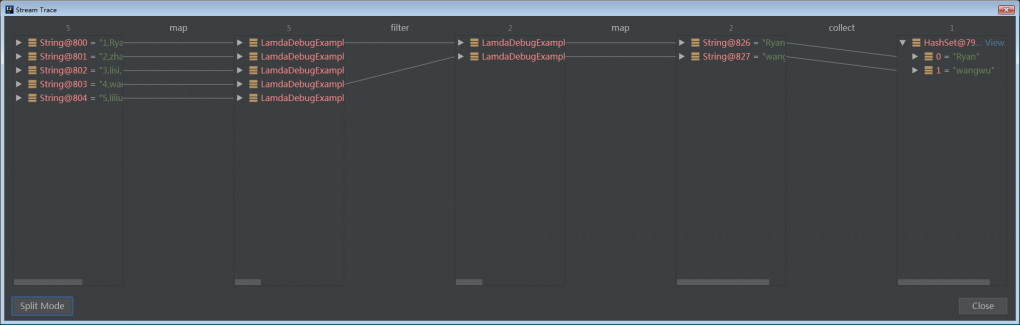
If you pay attention, you can see the switch display of "Split Mode" in the lower left corner. Every step of lambda operation and results can be displayed here. It is clear at a glance. Is it very humanized!
lambda debugging can be so simple.