preface
During web development, we often encounter the needs of file upload and analysis. For example, we upload and analyze Excel files, and finally insert the analyzed excel content into the database. Today, we will practice file upload and excel analysis. This blog mainly practices the file upload function, corresponding to Analysis of excel files , will practice in the next blog.
preparation:
This article uses the Commons - file upload jar,commons-io.jar
If it is a maven project, it needs to be in POM The XML configuration depends on
<dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>${commons.fileupload.version}</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.2</version> </dependency>
If it is not a maven project, download the of the jar package and import it into the project. The download address is as follows:
Link: https://pan.baidu.com/s/12FHorV5ImIn4aiCqFcBUkg Password: yxgj
Function realization:
Using the above two jar packages to realize the upload function is relatively simple. Here you can directly upload the code
Front end code: mainly a form
<form name="frm_test" action="${pageContext.request.contextPath }/uploadExcelFile" method="post" enctype="multipart/form-data"> user name:<input type="text" name="userName"> <br/> File: <input type="file" name="file"> <br/> <input name="upload" type="submit" value="upload"> </form>
Note: enctype = "multipart / form data", and < input type = "file" name = "file" >
If you don't pay attention to anything else, remember not to write the interface wrong
Background code:
@RequestMapping(value="/uploadExcelFile",method=RequestMethod.POST,produces = "text/html;charset=UTF-8") @ResponseBody public String uploadExcelFile(@RequestParam("file") CommonsMultipartFile file,HttpServletRequest request) { try { String path = request.getSession().getServletContext().getRealPath("/"); String fileName = "display.xlsx"; File excelFile = new File(path+fileName); LOGGER.info("uploadExcelFile file = "+excelFile.toString()); if(excelFile.exists()) { excelFile.delete(); } file.transferTo(excelFile); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); return "fail"; } return "success"; }
Here is a small point. Note that the file in @ RequestParam("file") should be consistent with the name = "file" in < input type = "file" name = "file" > in the front end.
This part of the code is a simple function implementation, which is not particularly in line with the design of spring mvc, because a good mvc design can not write too much logic in the controller layer, and the business logic should be written in the model layer. In addition, if returned, it simply returns success and fail. In addition to the above code, you also need to create an application context in the configuration file Configure a key bean in XML, otherwise an error will be reported when uploading.
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver" p:maxUploadSize="5242880" p:maxInMemorySize="4096" p:defaultEncoding="UTF-8"> </bean>
One thing to note here is that the bean id must be set to "multipartResolver", otherwise an error will also be reported.
Well, according to the above operations, you can basically upload files. Let's see the operation effect
Front end display:
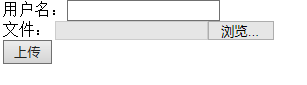
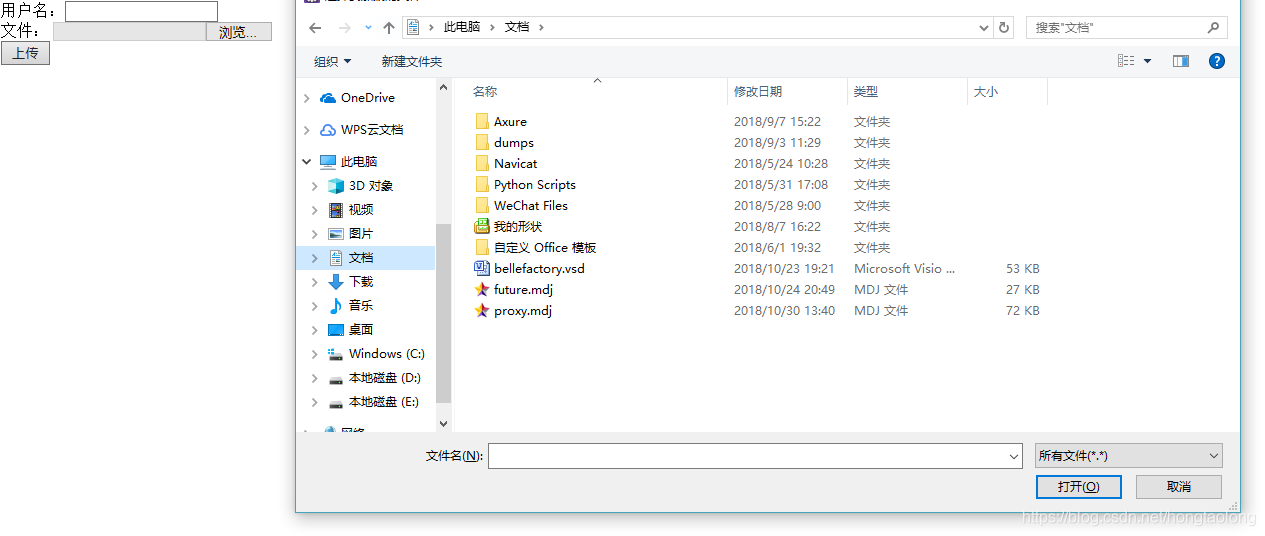
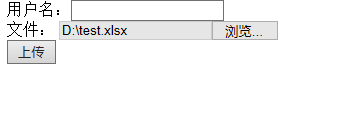
My side is the root directory of the project uploaded to the corresponding project in tomcat
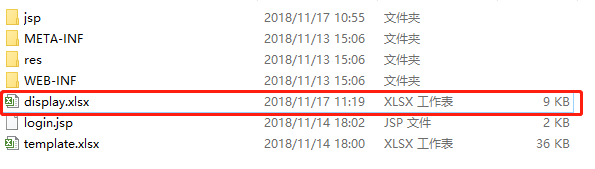
Here is just a file upload function. Next, let's see how to realize excel file parsing, excel file analysis