When using Vue for project, Baidu Map is useful, using vue-baidu-map plug-in, including picking up location coordinates, searching for locations, etc.
1. Ways of introduction
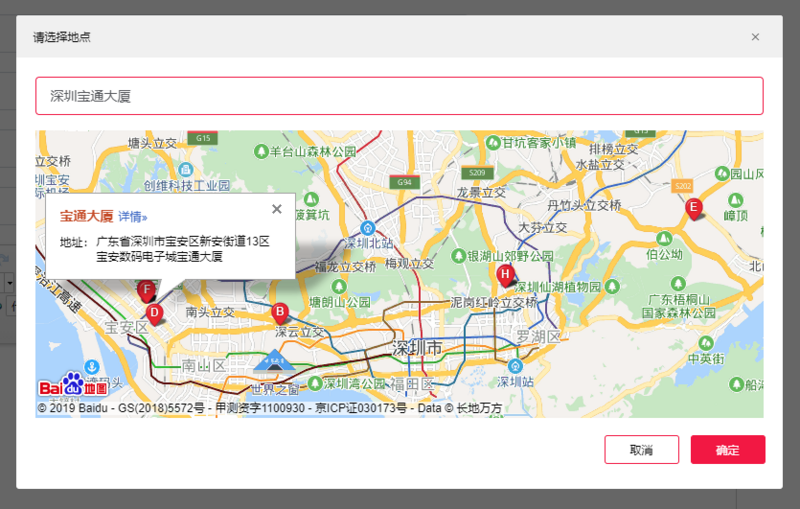
- Can be introduced globally in app.js
import BaiduMap from 'vue-baidu-map' Vue.use(BaiduMap, { /* You need to register Baidu Map Developer to get your ak */ ak: 'YOUR_APP_KEY' })
- Local introductions can also be done, where components are found in node_modules/vue-baidu-map/components and where AK attributes are declared in <baidu-map ak='></baidu-map>
import BaiduMap from 'vue-baidu-map/components/map/Map.vue' import BmView from 'vue-baidu-map/components/map/MapView.vue' import BmLocalSearch from 'vue-baidu-map/components/search/LocalSearch.vue' export default { components: { BaiduMap, BmView, BmLocalSearch }, }
- Use (local reference requires an ak attribute)
<div class="map"> <el-input v-model="addressKeyword" placeholder="Please enter an address to find the location directly"></el-input> <baidu-map class="bmView" :scroll-wheel-zoom="true" :center="location" :zoom="zoom" @click="getLocationPoint" ak="YOUR_APP_KEY"> <bm-view style="width: 100%; height:100px; flex: 1"></bm-view> <bm-local-search :keyword="addressKeyword" :auto-viewport="true" style="display: none"></bm-local-search> </baidu-map> </div>
Note: (Defined height that the map needs to display)
BmView is a container used to render the visualization area of Baidu Map Instances. It is often used in conjunction with components such as LocalSearch that output other views.
The BaiduMap component container itself is an empty block-level element. If the container does not define a height, the Baidu map will be rendered in a container that is not visible at a height of 0
Map components without center and zoom properties are not rendered.The exception is when the center attribute is a legal place name string, because Baidu Map automatically adjusts the zoom value based on the place name
Since the Baidu Map JS API only has JSONP as a loading method, rendering of BaiduMap components and all their subcomponents can only be asynchronous.Therefore, use the read event of the component to execute code that cannot be executed until the map API has been loaded, do not attempt to invoke the BMap class in the vue's own life cycle, and do not modify the model layer at these times.
The BaiduMap component container itself is an empty block-level element. If the container does not define a height, the Baidu map will be rendered in a container that is not visible at a height of 0
Map components without center and zoom properties are not rendered.The exception is when the center attribute is a legal place name string, because Baidu Map automatically adjusts the zoom value based on the place name
Since the Baidu Map JS API only has JSONP as a loading method, rendering of BaiduMap components and all their subcomponents can only be asynchronous.Therefore, use the read event of the component to execute code that cannot be executed until the map API has been loaded, do not attempt to invoke the BMap class in the vue's own life cycle, and do not modify the model layer at these times.
- Baidu Map Development Platform jsAPI: Baidu Map jsAPI
- vue-baidu-map document: vue-baidu-map document
- Golden Map vue-amap document: Gourde Map Document
2. Search
- Search uses bm-local-search to search. I don't know if it's because I use fixed positioning on the outside, so when I enter a location in the input box, I overwrite the map, not the official document, and then I use display: none to hide the container.
<div class="map"> <el-input v-model="addressKeyword" placeholder="Please enter an address to find the location directly"></el-input> <!-- Add a click event to the map getLocationPoint,Click on the map to get location-related information, latitude and longitude --> <!-- scroll-wheel-zoom: Whether you can use the mouse wheel to control map zooming, zoom Is View Scale --> <baidu-map class="bmView" :scroll-wheel-zoom="true" :center="location" :zoom="zoom" @click="getLocationPoint" ak="YOUR_APP_KEY" > <bm-view style="width: 100%; height:100px; flex: 1"></bm-view> <bm-local-search :keyword="addressKeyword" :auto-viewport="true" style="display: none"></bm-local-search> </baidu-map> </div>
data() { return { location: { lng: 116.404, lat: 39.915 }, zoom: 12.8, addressKeyword: "", }; }, methods: { getLocationPoint(e) { this.lng = e.point.lng; this.lat = e.point.lat; /* Create an instance of an address resolver */ let geoCoder = new BMap.Geocoder(); /* Get the coordinates of the location */ geoCoder.getPoint(this.addressKeyword, point => { this.selectedLng = point.lng; this.selectedLat = point.lat; }); /* Getting address details using coordinates */ geocoder.getLocation(e.point, res=>{ console.log(res); }, }