1, Foreword
When a user registers, if the password is not encrypted, it is directly stored in plaintext in the database. Once the database is leaked, it is a very serious problem for the user and the company.
2, Use js-md5 package to encrypt
1. md5 introduction
MD5 message digest algorithm (English: MD5 message digest algorithm), a widely used cryptographic hash function, can generate a 128 bit (16 byte) hash value to ensure complete and consistent information transmission. A 128 bit MD5 hash is typically represented as a 32-bit hexadecimal value.
2. Use js-md5
- Installation:
$ npm install js-md5
- Introduction and use
const md5 = require('js-md5') md5('123456') // e10adc3949ba59abbe56e057f20f883e
3. Disadvantages of MD5 encryption
Theoretically, it cannot be cracked because md5 uses an irreversible algorithm.
Some websites provide MD5 decryption because there is a lot of storage space to save the source code and encrypted password. When decrypting, it is a query process, and a slightly more complex query cannot be completed.
This decryption method is called dictionary attack
3, bcryptjs
The solution to the dictionary attack is to add salt.
bcryptjs is an excellent package dealing with salt encryption in nodejs.
1. What is Salt
The so-called adding salt is to add some "seasoning" on the basis of encryption. This "seasoning" is a random value randomly generated by the system and mixed in the encrypted password in a random way.
Since "seasoning" is randomly generated by the system, different strings will be generated for the same original password after adding "seasoning".
This greatly increases the difficulty of cracking.
If you can't add salt, you can also have some monosodium glutamate, chicken essence, ginger and pepper
2. Use bcryptjs
1. Installation
$ npm install bcryptjs
2. Use:
// Introducing bcryptjs const bcryptjs = require('bcryptjs') // Original password const password = '123456' /** * Encryption processing synchronization method * bcryptjs.hashSync(data, salt) * - data Data to encrypt * - slat The salt used to hash the password. If specified as a number, the salt is generated and used with the specified number of rounds. Recommendation 10 */ const hashPassword = bcryptjs.hashSync(password, 10) /** * output * Note: the output will be different for each call */ console.log(hashPassword) // $2a$10$P8x85FYSpm8xYTLKL/52R.6MhKtCwmiICN2A7tqLDh6rDEsrHtV1W /** * Verification - use synchronization method * bcryptjs.compareSync(data, encrypted) * - data To compare the data, use the password passed during login * - encrypted To compare the data, use the encrypted password queried from the database */ const isOk = bcryptjs.compareSync(password, '$2a$10$P8x85FYSpm8xYTLKL/52R.6MhKtCwmiICN2A7tqLDh6rDEsrHtV1W') console.log(isOk)
There is a problem: in bcrypt Comparesync, why is there no salt parameter? Since the hash is generated from salt, why does the comparison of plaintext passwords not involve the original salt used in the hash?
Although the hash generated each time is different for the same password, However, salt is included in the hash (hash generation process: generate salt randomly first, and hash salt and password); in the next verification, take salt from the hash, and hash salt and password; compare the results with the hash saved in DB. This process has been implemented in compareSync: bcrypt.compareSync(password, hashFromDB);
Look at the code:
const bcryptjs = require('bcryptjs') // Original password const password = '123456' /** * Encryption processing synchronization method * bcryptjs.hashSync(data, salt) * - data Data to encrypt * - slat The salt used to hash the password. If specified as a number, the salt is generated and used with the specified number of rounds. Recommendation 10 */ const hashPassword = ()=>bcryptjs.hashSync(password, 8); console.log(hashPassword()) console.log(hashPassword()) console.log(hashPassword()) console.log(hashPassword()) console.log(hashPassword()) console.log(hashPassword()) console.log(hashPassword())
In the code, we added 8 salt to generate the password for many times. Let's see the print results:
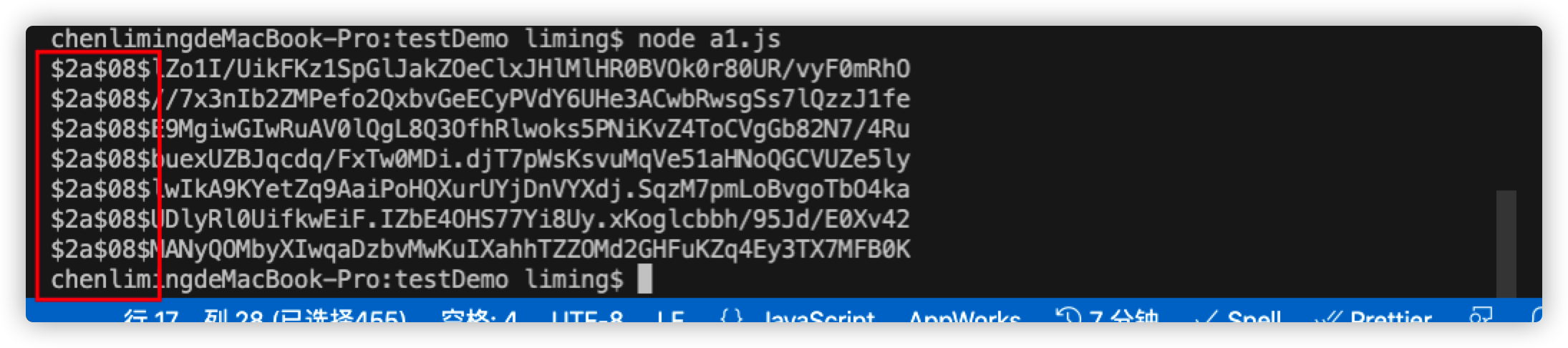
We see that salt is saved in the generated password, and the salt will be taken out every time we verify.
The bcrypt standard makes storing salts easy - everything it needs to check a password is stored in the output string.
The prefix "$2a$" or "2y" in a hash string in a shadow password file indicates that hash string is a bcrypt hash in modular crypt format. The rest of the hash string includes the cost parameter, a 128-bit salt (base-64 encoded as 22 characters), and the 192-bit[dubious – discuss] hash value (base-64 encoded as 31 characters).
The above is the method of using bcryptjs encryption. I hope it will help you.