1. Introduction to filter
The concept of filter should be familiar to everyone, especially to those who start with Servlet and learn Java background. So what can we do with this thing? Filter filter is mainly used to filter user requests. It allows us to pre process and post process user requests, such as realizing URL level permission control, filtering illegal requests and so on. Filter filter is a specific implementation of AOP (AOP aspect programming is just a programming idea).
In addition, Filter depends on the Servlet container. The Filter interface is under the Servlet package and belongs to a part of the Servlet specification. Therefore, we often call it "enhanced Servlet".
If we need to customize the filter, it is very simple. We only need to implement javax Servlet. Filter interface, and then rewrite the three methods inside!
Filter.java
public interface Filter { //Action after initializing the filter default void init(FilterConfig filterConfig) throws ServletException { } // Filter requests void doFilter(ServletRequest var1, ServletResponse var2, FilterChain var3) throws IOException, ServletException; // The operation performed after the filter is destroyed is mainly the user's recycling of some resources default void destroy() { } }
2. How does filter intercept?
There is a method called doFilter in the Filter interface, which implements the filtering of user requests. The specific process is generally as follows:
- The user sends a request to the web server, and the request will arrive at the filter first;
- The filter will process the request, such as filtering the parameters of the request, modifying the content of the response returned to the client, determining whether to let the user access the interface, and so on.
- User request response completed.
- Do something else you want.
3. How to customize a Filter
3.1 internship javax Servlet. Filter interface
@Component public class MyFilter1 implements Filter { @Override public void init(FilterConfig filterConfig) throws ServletException { System.out.println("Initialization method: execute only once when the server starts initialization"); } @Override public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException { System.out.println("Perform filter operation"); /*Release operation*/ filterChain.doFilter(servletRequest,servletResponse); /*After executing the corresponding method, I passed through the filter*/ System.out.println("It's done,Echo to client"); } /*Execute when the server shuts down normally*/ @Override public void destroy() { System.out.println("The destruction method is executed only once when the server is shut down"); } }
3.2 register custom filters in configuration
@Configuration public class MyFilterConfig { @Autowired MyFilter1 myFilter1; @Bean public FilterRegistrationBean<MyFilter1> Filter1(){ FilterRegistrationBean<MyFilter1> myFilter1FilterRegistrationBean = new FilterRegistrationBean<>(); /*Set Filter*/ myFilter1FilterRegistrationBean.setFilter(myFilter1); /*Set access path*/ myFilter1FilterRegistrationBean.setUrlPatterns(new ArrayList<>(Arrays.asList("/filter/*"))); return myFilter1FilterRegistrationBean; } }
3.3 configuration through annotation
be careful:
**
To use WebFilter, you need to add @ ServletComponentScan} annotation on the startup class.
@WebFilter(filterName = "MyFilter1",urlPatterns = "/filter") public class MyFilter1 implements Filter { @Override public void init(FilterConfig filterConfig) throws ServletException { System.out.println("Initialization method: execute only once when the server starts initialization"); } @Override public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException { System.out.println("Perform filter operation"); /*Release operation*/ filterChain.doFilter(servletRequest,servletResponse); /*After executing the corresponding method, I passed through the filter*/ System.out.println("It's done,Echo to client"); } /*Execute when the server shuts down normally*/ @Override public void destroy() { System.out.println("The destruction method is executed only once when the server is shut down"); } }
4. Customize multiple filters to determine the execution order of filters
Operate by setting the filter level, and call the setOrder method of FilterRegistrationBean
package com.pjh.Config; import com.pjh.Filter.MyFilter1; import com.pjh.Filter.MyFilter2; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.web.servlet.FilterRegistrationBean; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import java.util.ArrayList; import java.util.Arrays; /** * @ClassName: MyFilterConfig * @Author: 86151 * @Date: 2021/4/14 14:29 * @Description: TODO */ @Configuration public class MyFilterConfig { @Autowired MyFilter1 myFilter1; @Autowired MyFilter2 myFilter2; @Bean public FilterRegistrationBean<MyFilter1> Filter1(){ FilterRegistrationBean<MyFilter1> myFilter1FilterRegistrationBean = new FilterRegistrationBean<>(); /*Set Filter*/ myFilter1FilterRegistrationBean.setFilter(myFilter1); /*Sets the level of the filter*/ myFilter1FilterRegistrationBean.setOrder(1); /*Set access path*/ myFilter1FilterRegistrationBean.setUrlPatterns(new ArrayList<>(Arrays.asList("/filter/*"))); return myFilter1FilterRegistrationBean; } @Bean public FilterRegistrationBean<MyFilter2> Filter2(){ FilterRegistrationBean<MyFilter2> myFilter1FilterRegistrationBean = new FilterRegistrationBean<>(); /*Set Filter*/ myFilter1FilterRegistrationBean.setFilter(myFilter2); /*Sets the level of the filter*/ myFilter1FilterRegistrationBean.setOrder(2); /*Set access path*/ myFilter1FilterRegistrationBean.setUrlPatterns(new ArrayList<>(Arrays.asList("/filter/*"))); return myFilter1FilterRegistrationBean; } }
5. Introduction of relevant notes
@WebFilter
summary
@WebFilter is used to declare a class as a filter. The annotation will be processed by the container during deployment. The container will deploy the corresponding class as a filter according to the specific attribute configuration. The annotation has some common attributes given in the following table (all the following attributes are optional, but value, urlPatterns and servletNames must contain at least one, and value and urlPatterns cannot coexist. If they are specified at the same time, the value of value is usually ignored)
@Common properties of WebFilter
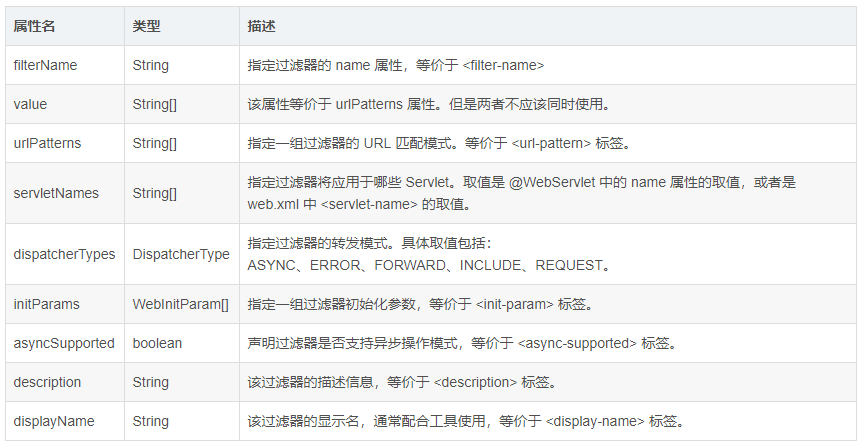
matters needing attention
filterName The first letter of must be lowercase!!! a lowercase letter!!! a lowercase letter!!!
Application Start class addition@ServletComponentScan annotation
@Order
summary
The annotation @ Order or Ordered interface is used to define the priority of the execution Order of beans in the Spring IOC container, rather than the loading Order of beans. The loading Order of beans is not affected by the @ Order or Ordered interface;
code implementation
@Retention(RetentionPolicy.RUNTIME) @Target({ElementType.TYPE, ElementType.METHOD, ElementType.FIELD}) @Documented public @interface Order { /** * The default is the lowest priority. The smaller the value, the higher the priority */ int value() default Ordered.LOWEST_PRECEDENCE; }