When using the database, you need to view the list of drivers supported in the installed QT. Generally, you can view all the driver plug-in files in the plugins/sqldrivers folder in the QT installation directory.
This is not the focus of this article, so I will not elaborate.
The following should be added to the xxx.pro project file:
QT += sql
Connect to the SQLite database:
After compilation, a my.db database file is generated in the directory. There is a table named user in the database.#include <QMessageBox> #include <QSqlDatabase> #include <QSqlQuery> #include <QDebug> static bool createConnection() { QSqlDatabase db = QSqlDatabase::addDatabase("QSQLITE"); db.setDatabaseName("my.db"); if (!db.open()) { QMessageBox::critical(0, "Cannot open database1", "Unable to establish a database connection.", QMessageBox::Cancel); return false; } QSqlQuery query; // Create a login user table query.exec("create table user (username varchar primary key, passwd varchar)"); query.exec("insert into user values('admin', 'root')"); }
#include <QMessageBox> #include <QSqlDatabase> #include <QSqlQuery> static bool createConnection() { // Create a database connection using "Connection 1" as the connection name QSqlDatabase db1 = QSqlDatabase::addDatabase("QSQLITE", "connection1"); db1.setDatabaseName("my1.db"); if (!db1.open()) { QMessageBox::critical(0, "Cannot open database1", "Unable to establish a database connection.", QMessageBox::Cancel); return false; } // Here you specify the connection QSqlQuery query1(db1); query1.exec("create table student (id int primary key, " "name varchar(20))"); query1.exec("insert into student values(0, 'LiMing')"); query1.exec("insert into student values(1, 'LiuTao')"); query1.exec("insert into student values(2, 'WangHong')"); // To create another database connection, use a different connection name, here is "Connection 2" QSqlDatabase db2 = QSqlDatabase::addDatabase("QSQLITE", "connection2"); db2.setDatabaseName("my2.db"); if (!db2.open()) { QMessageBox::critical(0, "Cannot open database1", "Unable to establish a database connection.", QMessageBox::Cancel); return false; } // Here you specify the connection QSqlQuery query2(db2); query2.exec("create table student (id int primary key, " "name varchar(20))"); query2.exec("insert into student values(10, 'LiQiang')"); query2.exec("insert into student values(11, 'MaLiang')"); query2.exec("insert into student values(12, 'ZhangBin')"); return true; }
Connect to the SQL Server database:
Prerequisites:
Installed SQL Server 2008 and created database MyDB in SQL Server
Overview of Qt Connecting SQL Server 2008 through ODBC:
When Qt connects to database through ODBC, the database name used is not written directly to the database name, but DSN name.
There are two ways to use DSN names:
1. Configure DSN in operating system;
2. Using DSN connection string to connect ODBC database directly in Qt program code.
Here is a detailed description of how to connect SQL Server 2008 with DSN configuration in the operating system
Configuring DSN in Operating System
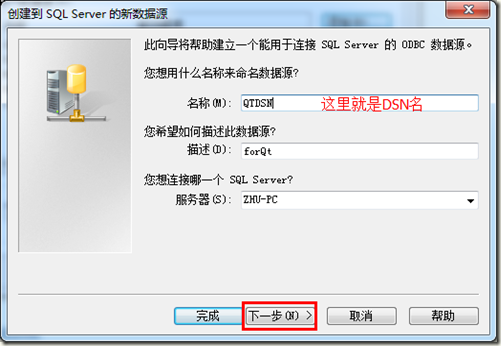
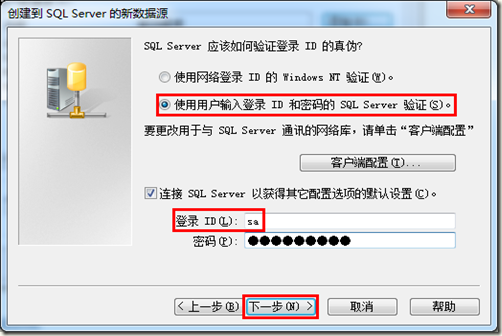
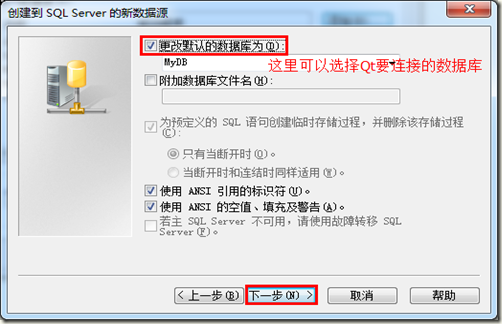
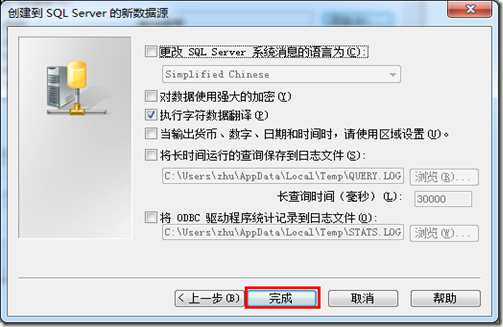
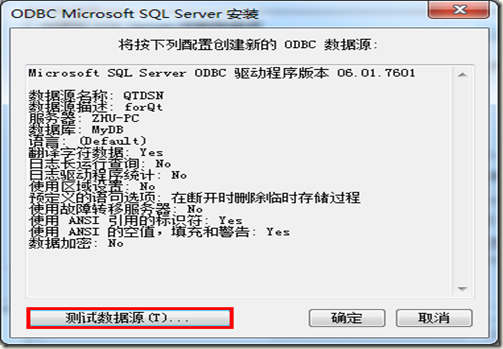
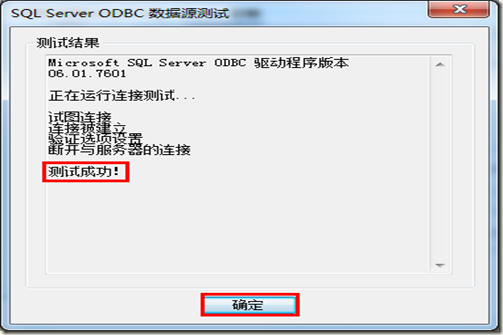
So far, the link is complete.QSqlDatabase db = QSqlDatabase::addDatabase("QODBC"); QString dsn = QString::fromLocal8Bit("QTDSN"); db.setHostName("127.0.0.1"); db.setDatabaseName(dsn); db.setUserName("sa"); db.setPassword("123456");
#include <QMessageBox> #include <QSqlDatabase> #include <QSqlQuery> #include <QDebug> static bool createConnection() { QSqlDatabase db = QSqlDatabase::addDatabase("QODBC"); QString dsn = QString::fromLocal8Bit("QTDSN"); db.setHostName("127.0.0.1"); db.setDatabaseName(dsn); db.setUserName("sa"); db.setPassword("123456"); if (!db.open()) { QMessageBox::critical(0, "Cannot open database1", "Unable to establish a database connection.", QMessageBox::Cancel); return false; } QSqlQuery query; // Create a login user table query.exec("select * from user"); while(query.next()){ qDebug()<<query.value(0).toString(); qDebug()<<query.value(1).toString(); } }