In website development, we often encounter the situation of generating two-dimensional codes, such as using Wechat payment, web page login, etc. This paper shares an example of generating two-dimensional codes by Spring Boot, which uses the zxing tool class of google.
Contents of this article
Introduction of Two-Dimensional Code2. Writing Code to Generate Two-Dimensional Code1. Introducing jar packages2. Writing Tool Classes3. Writing Control Layer Code4. Run and see the results
Introduction of Two-Dimensional Code
Two-dimensional codes, also known as QR Code, QR full name Quick Response, is a super popular coding method on mobile devices in recent years.
Two-dimensional codes record data symbolic information with black-and-white graphics which are distributed in the plane (two-dimensional direction) according to certain rules by a specific geometric figure.
The main application scenarios are as follows:
- Information Acquisition (Business Card, Map, WIFI Password, Information)
- Web site jump (jump to microblog, mobile website, website)
- Advertising push (user scan code, browse video and audio advertisements pushed by merchants directly)
- Mobile E-Commerce (User Scan Code, Mobile Direct Shopping Order)
- Anti-counterfeiting traceability (users can scan the code to see the place of production; at the same time, the background can obtain the final place of consumption)
- Preferential promotions (user scanning, downloading electronic coupons, lottery draw)
- Membership management (access to electronic membership information, VIP services on users'mobile phones)
- Mobile Payment (Scanning 2-D Code of Commodity and Completing Payment through Mobile Channel Provided by Bank or Third Party Payment)
- Account Logon (Scanning two-dimensional code for each website or software login)
2. Writing Code to Generate Two-Dimensional Code
1. Introducing jar packages
The jar package of zxing is introduced into pom.xml.
<!-- Two-Dimensional Code Support Packet -->
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.2.0</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.2.0</version>
</dependency>
2. Writing Tool Classes
The QRCodeUtil.java code is as follows:
/**
* QRCodeUtil Generating Two-Dimensional Code Tool Class
*/
public class QRCodeUtil {
private static final String CHARSET = "utf-8";
private static final String FORMAT_NAME = "JPG";
//Two-dimensional code size
private static final int QRCODE_SIZE = 300;
//LOGO Width
private static final int WIDTH = 60;
//LOGO Height
private static final int HEIGHT = 60;
private static BufferedImage createImage(String content, String imgPath, boolean needCompress) throws Exception {
Hashtable hints = new Hashtable();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET, CHARSET);
hints.put(EncodeHintType.MARGIN, 1);
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, QRCODE_SIZE, QRCODE_SIZE,
hints);
int width = bitMatrix.getWidth();
int height = bitMatrix.getHeight();
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, bitMatrix.get(x, y) ? 0xFF000000 : 0xFFFFFFFF);
}
}
if (imgPath == null || "".equals(imgPath)) {
return image;
}
//Insert pictures
QRCodeUtil.insertImage(image, imgPath, needCompress);
return image;
}
private static void insertImage(BufferedImage source, String imgPath, boolean needCompress) throws Exception {
File file = new File(imgPath);
if (!file.exists()) {
System.err.println("" + imgPath + " This file does not exist!");
return;
}
Image src = ImageIO.read(new File(imgPath));
int width = src.getWidth(null);
int height = src.getHeight(null);
if (needCompress) { //Compression LOGO
if (width > WIDTH) {
width = WIDTH;
}
if (height > HEIGHT) {
height = HEIGHT;
}
Image image = src.getScaledInstance(width, height, Image.SCALE_SMOOTH);
BufferedImage tag = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(image, 0, 0, null); //Draw a reduced map
g.dispose();
src = image;
}
//Insert LOGO
Graphics2D graph = source.createGraphics();
int x = (QRCODE_SIZE - width) / 2;
int y = (QRCODE_SIZE - height) / 2;
graph.drawImage(src, x, y, width, height, null);
Shape shape = new RoundRectangle2D.Float(x, y, width, width, 6, 6);
graph.setStroke(new BasicStroke(3f));
graph.draw(shape);
graph.dispose();
}
public static void encode(String content, String imgPath, String destPath, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
mkdirs(destPath);
ImageIO.write(image, FORMAT_NAME, new File(destPath));
}
public static BufferedImage encode(String content, String imgPath, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
return image;
}
public static void mkdirs(String destPath) {
File file = new File(destPath);
//When a folder does not exist, mkdirs automatically creates a multi-tier directory, unlike mkdir. (mkdir throws an exception if the parent directory does not exist.)
if (!file.exists() && !file.isDirectory()) {
file.mkdirs();
}
}
public static void encode(String content, String imgPath, OutputStream output, boolean needCompress)
throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
ImageIO.write(image, FORMAT_NAME, output);
}
public static void encode(String content, OutputStream output) throws Exception {
QRCodeUtil.encode(content, null, output, false);
}
}
3. Writing Control Layer Code
The QrCodeController.java code is as follows:
/**
* Generation of Ordinary Two-Dimensional Codes Based on url
*/
@RequestMapping(value = "/createCommonQRCode")
public void createCommonQRCode(HttpServletResponse response, String url) throws Exception {
ServletOutputStream stream = null;
try {
stream = response.getOutputStream();
//Using Tool Class to Generate Two-Dimensional Codes
QRCodeUtil.encode(url, stream);
} catch (Exception e) {
e.getStackTrace();
} finally {
if (stream != null) {
stream.flush();
stream.close();
}
}
}
/**
* Generation of two-dimensional codes with logo based on url
*/
@RequestMapping(value = "/createLogoQRCode")
public void createLogoQRCode(HttpServletResponse response, String url) throws Exception {
ServletOutputStream stream = null;
try {
stream = response.getOutputStream();
String logoPath = Thread.currentThread().getContextClassLoader().getResource("").getPath()
+ "templates" + File.separator + "logo.jpg";
//Using Tool Class to Generate Two-Dimensional Codes
QRCodeUtil.encode(url, logoPath, stream, true);
} catch (Exception e) {
e.getStackTrace();
} finally {
if (stream != null) {
stream.flush();
stream.close();
}
}
}
4. Run and see the results
In this project, two interfaces are provided to generate ordinary two-dimensional codes and two-dimensional codes with logo. Start the project. Let's demonstrate how to generate the two-dimensional codes of http://www.baidu.com url.
- Generating Ordinary Two-Dimensional Codes
The local browser opens http://localhost:8080/createCommonQRCode?url=http://www.baidu.com. The generated two-dimensional code screenshots are as follows:
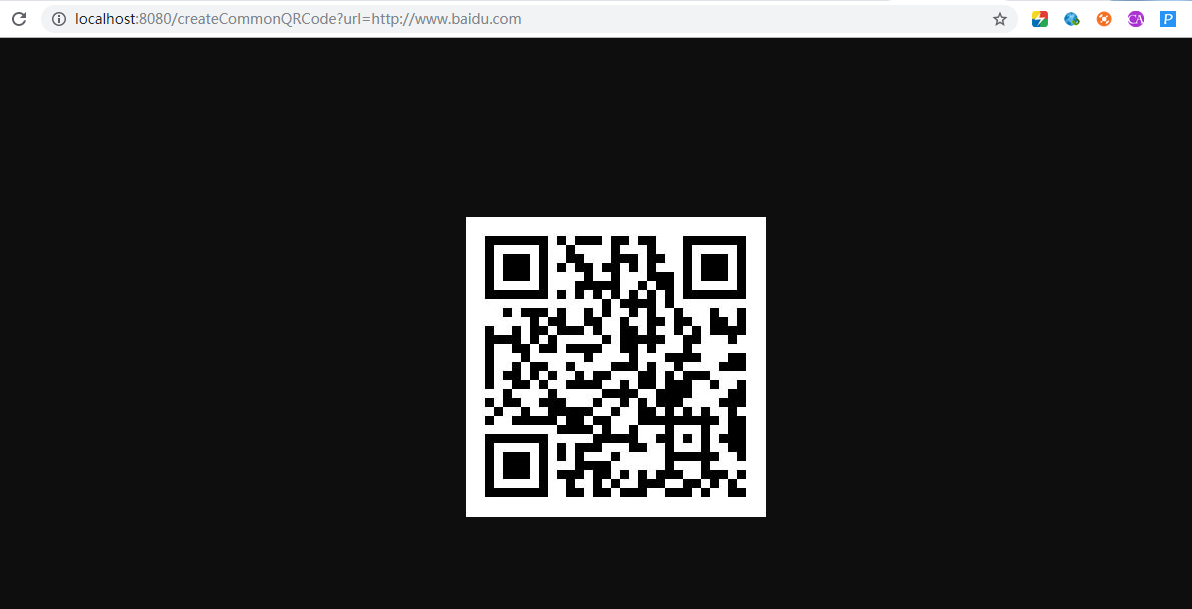
Generating Baidu's Normal Two-Dimensional Code
- Generating two-dimensional codes with logo
The local browser opens http://localhost:8080/createLogoQRCode?url=http://www.baidu.com. The generated two-dimensional code screenshots are as follows:
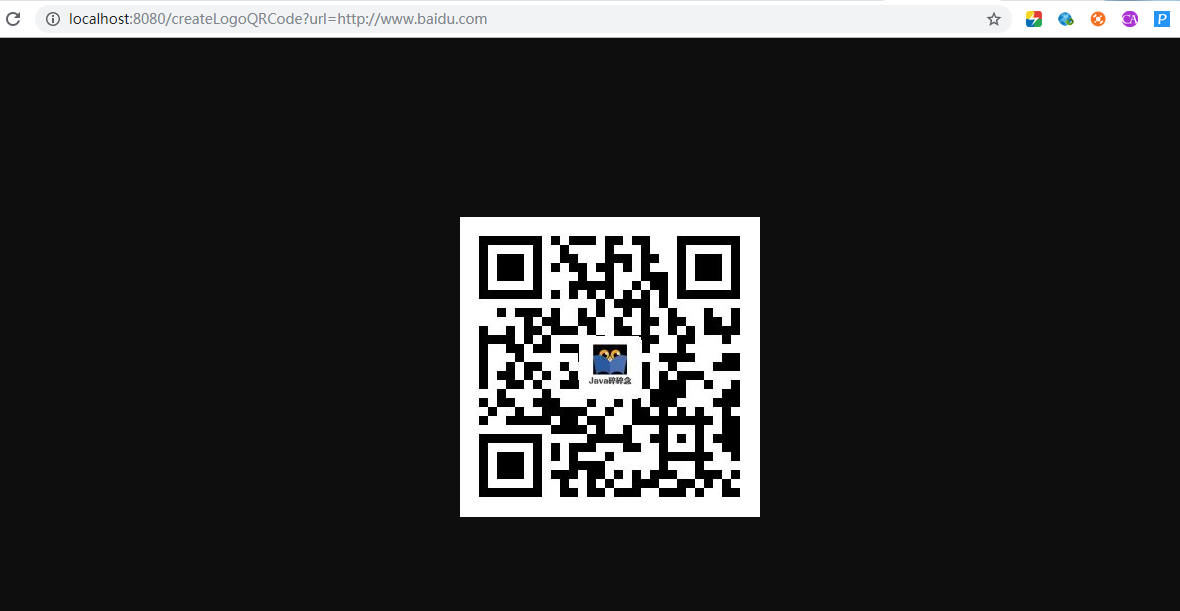
Generating two-dimensional code with logo for Baidu
Spring Boot 2.X to use ZXing to generate two-dimensional code functions are fully realized, if you have any questions, please leave a message to communicate oh!
Full source address: https://github.com/suisui2019/spring boot-study
Recommended reading
1. From a technical point of view, why not send the original map online?
2. How does Spring Boot 2.X integrate jpa quickly?
3. Profile of Spring Boot--Quickly Solve Multi-environment Use and Switching
4.Spring Boot 2.X integrates Spring-cache to speed up your website
5. Using Spring Boot+WxJava to Realize Web Site Integrated Weichat Logon Function
Timely access to free Java-related information covers Java, Redis, MongoDB, MySQL, Zookeeper, Spring Cloud, Dubbo/Kafka, Hadoop, Hbase, Flink and other high-concurrent distributed, large data, machine learning technology.
Pay attention to the following public numbers for free:
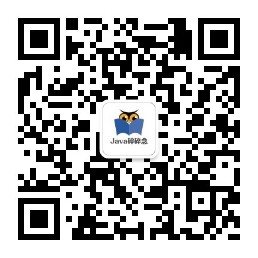