Using vuejs and mockjs to simulate shopping cart
1. If you don't know mockjs, it's recommended to go to the official website to learn about it: Mockjs
2. The first step is to make the simulation data by yourself (of course, you can also use the MVC mode of nodejs to introduce mockjs to obtain the simulation data, and use ajax cross domain request to obtain the back-end interface data in the front-end);
- First of all, we need to use the nodejs instruction to build the environment, which requires the nodejs foundation.
- The following is the code block of mockModel simulation data layer, including the introduction of mockjs module:
const Mock = require('mockjs');
var arr = [];
for(var i = 0 ; i < 20 ; i++){
var data = Mock.mock({
'id': i+1,
'isSelected':false,
'productPic':Mock.Random.image('100×100','#fb0a2a'),
'productName':Mock.Random.cparagraph(1),
'productInfo':Mock.Random.cparagraph(1),
'price':Mock.Random.float(0,100),
'number':1
})
arr.push(data);
}
module.exports = {
arr
}
- The following is the shopController.js module of the Controllers layer, which provides the api interface data format
const mockData = require('../mockModel/mockModel');
function shopController (req, res) {
shopCar = {
error_code:0,
reason:'Data returned successfully',
shopcar:mockData.arr
}
res.json(shopCar);
};
module.exports = {
shopController,
}
- Here is the routing shop.js module:
var express = require('express');
var router = express.Router();
var shopCatCon = require('../controllers/shopController');
/* GET users listing. */
router.get('/list', shopCatCon.shopController);
module.exports = router;
- Here is the app.js section:
var express = require('express');
var path = require('path');
var cors = require('cors');
var shopsRouter = require('./routes/shops');
var app = express();
app.use(cors());
app.use('/shop', shopsRouter);
var port = 3000;
app.listen(port,()=>{
console.log('this server is running at '+port)
})
module.exports = app;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Bootstrap Shopping Cart</title>
</head>
<link rel="stylesheet" type="text/css" href="css/bootstrap.css">
<link type="text/css" rel="stylesheet" href="css/style.css" />
<body>
<div class="shopping-car-container" id="cartBox">
<div class="car-headers-menu">
<div class="row">
<div class="col-md-1 car-menu">
<label><input type="checkbox" id="check-goods-all" v-model="isSelectedAll"/><span id="checkAll">All election</span></label>
</div>
<div class="col-md-3 car-menu">Commodity information</div>
<div class="col-md-3 car-menu">Commodity parameter</div>
<div class="col-md-1 car-menu">Unit Price</div>
<div class="col-md-1 car-menu">Number</div>
<div class="col-md-1 car-menu">Amount of money</div>
<div class="col-md-2 car-menu">operation</div>
</div>
</div>
<div class="goods-content" v-for="item in shopCar">
<div class="goods-item">
<div class="panel panel-default">
<div class="panel-body">
<div class="col-md-1 car-goods-info">
<label><input type="checkbox" class="goods-list-item" v-model="item.isSelected" /></label>
</div>
<div class="col-md-3 car-goods-info goods-image-column">
<img class="goods-image" :src=" item.productPic " style="width: 100px; height: 100px;" />
<span id="goods-info">
{{ item.productInfo }}
</span>
</div>
<div class="col-md-3 car-goods-info goods-params">{{ item.productName }} </div>
<div class="col-md-1 car-goods-info goods-price"><span>¥</span><span class="single-price">
{{ item.price | toFixed(2) }} </span></div>
<div class="col-md-1 car-goods-info goods-counts">
<div class="input-group">
<div class="input-group-btn">
<button type="button" class="btn btn-default car-decrease">-</button>
</div>
<input type="text" class="form-control goods-count" value="" v-model=" item.number ">
<div class="input-group-btn">
<button type="button" class="btn btn-default car-add">+</button>
</div>
</div>
</div>
<div class="col-md-1 car-goods-info goods-money-count"><span>¥</span><span class="single-total">
{{ priceSum(item.price,item.number) | toFixed(2) }}</span></div>
<div class="col-md-2 car-goods-info goods-operate">
<button type="button" class="btn btn-danger item-delete" @click="delProduct(item)">delete</button>
</div>
</div>
</div>
</div>
</div>
<div class="panel panel-default">
<div class="panel-body bottom-menu-include">
<div class="col-md-1 check-all-bottom bottom-menu">
<label>
<input type="checkbox" id="checked-all-bottom" />
<span id="checkAllBottom">All election</span>
</label>
</div>
<div class="col-md-1 bottom-menu">
<span id="deleteMulty">
delete
</span>
</div>
<div class="col-md-6 bottom-menu">
</div>
<div class="col-md-2 bottom-menu">
<span>Selected commodities <span id="selectGoodsCount">0</span> piece</span>
</div>
<div class="col-md-1 bottom-menu">
<span>Total:<span id="selectGoodsMoney">{{ total | toFixed(2) }}</span></span>
</div>
<div class="col-md-1 bottom-menu submitData" :style="style">
Settlement
</div>
</div>
</div>
</div>
</body>
</html>
<script type="text/javascript" src="js/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script type="text/javascript" src="js/renderVue.js"></script>
- Here's the JavaScript section
$.ajax({
url:'http://localhost:3000/shop/list',
type:'GET',
dataType:'json',
success:(data)=>{
shiLiVue(data)
}
});
function shiLiVue(data){
var shopCar = data.shopcar;
var vm = new Vue({
el:'#cartBox',
data:{
shopCar,
number:0,
style:'',
isSelected:false,
},
computed:{
priceSum(){
var lPriceSum = 0;
return function(pr,num){
lPriceSum = pr * num;
return lPriceSum;
};
},
isSelectedAll:{
get:function(){
return this.shopCar.every((ele,index)=>{
return ele.isSelected;
});
},
set:function(val){
console.log(val);
return this.shopCar.map((ele,index)=>{
return ele.isSelected = val;
});
}
},
total:function(){
var totalAll;
totalAll = this.shopCar.reduce((pre,nextItem)=>{
console.log(pre,nextItem);
if(nextItem.isSelected){
return pre + (nextItem.price * nextItem.number);
}
else{
return pre;
}
},0);
return totalAll;
}
},
filters:{
toFixed:function(input,params){
return input.toFixed(params);
}
},
methods:{
delProduct:function(item){
this.shopCar = this.shopCar.filter((ele,index)=>{
return ele !== item;
});
},
}
});
}
Well, that's about the whole thing. Let's see the effect! Hee hee, may write a little more, hope to have a passing small partner, what optimization please leave a message. Thank you
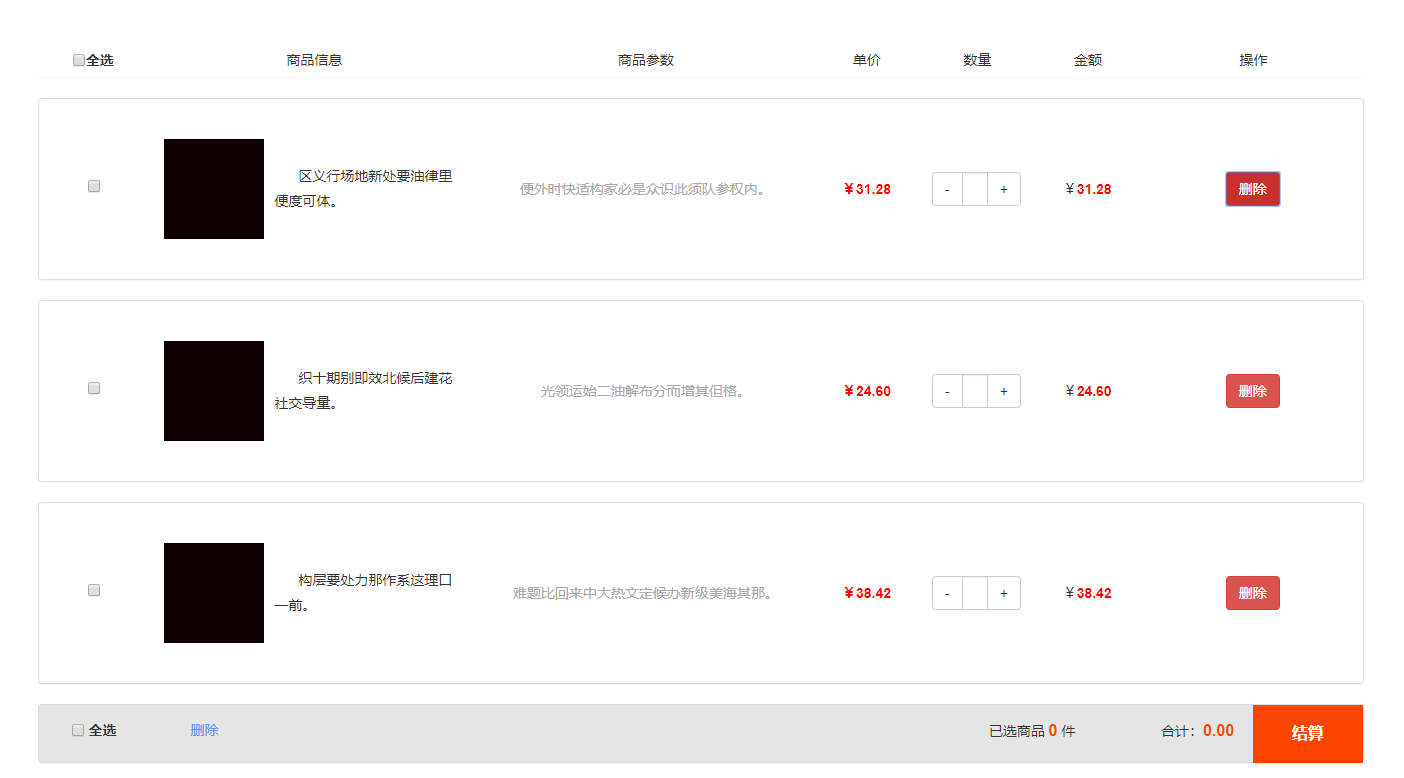