Value exchange
I. Basic data type value exchange
class Demo { public static void change(int a, int b) { int temp = a; a = b; b = temp; } public static void main(String[] args) { int a = 10; int b = 20; System.out.println("Before exchange:"+"a= "+a+" b= "+b); //Before exchange: a= 10 b= 20 change(a,b); System.out.println("After exchange:"+"a= "+a+" b= "+b); //After exchange: a= 10 b= 20 } }
There is no change in the value of the variable before and after the change.
Reason:
When the main function runs, it will open up its own space in the stack memory. When the main function calls the change function, it will also open up its own space in the stack memory, with its own a and b variables. When the change method ends, the A and b variables in the change method will also disappear.
A key:
1. The formal parameter is the local variable of the function to which the data belongs.
2. The local variables and local variables of different functions are independent of each other without any relationship.
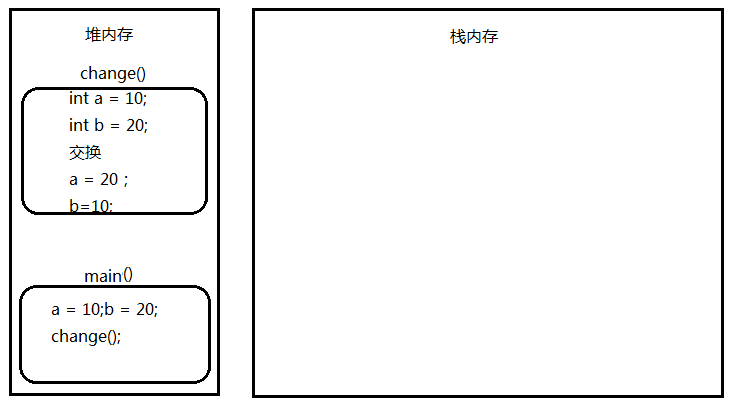
2. Array type value exchange
class Demo { public static void change(int[] arr, int index1,int index2) { int temp = arr[index1]; arr[index1] = arr[index2]; arr[index2] = temp; } public static void main(String[] args) { int[] arr = {1,2,3,4,5,6}; System.out.println("Before exchange:"+Arrays.toString(arr)); //Before exchange: [1, 2, 3, 4, 5, 6] change(arr,1,2); System.out.println("After exchange:"+Arrays.toString(arr)); //After exchange: [1, 3, 2, 4, 5, 6] } }
Exchange succeeded. Reason: the main method and the change method operate on the same object.
Note: 1. The ARR of the two functions is different. 2. The two different variables of arr operate on the same object, so the exchange is successful.
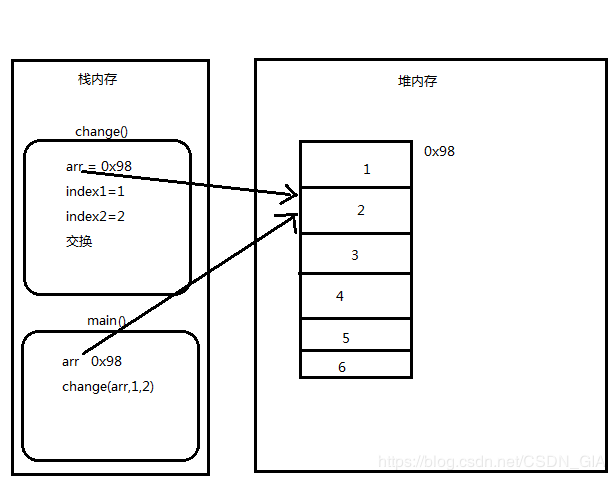
III. value exchange of objects
class MyTest{ int x = 10; public MyTest(int x) { this.x = x; } } class Demo { public static void change(MyTest myTest,int x) { myTest.x = x; } public static void main(String[] args) { MyTest myTest = new MyTest(1); System.out.println("Before modification:"+myTest.x); //Before modification: 1 change(myTest,2); System.out.println("After modification:"+myTest.x); //After modification: 2 } }
Successful exchange: different reference type variables operate on the same object, which will definitely affect the result.
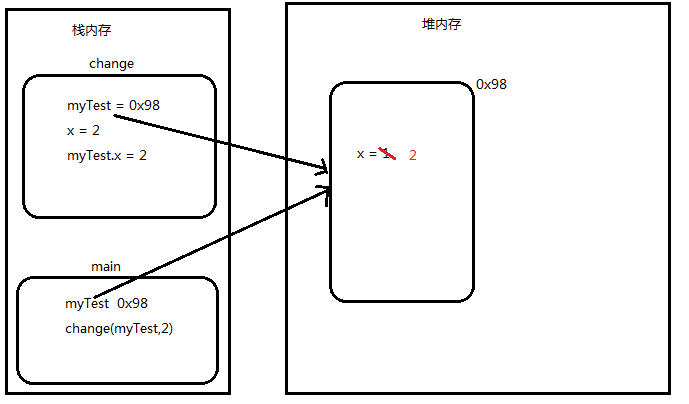
IV. string value exchange
class Demo { public static void change(String source,String target) { source = target; } public static void main(String[] args) { String str = "CSDN_HCX"; System.out.println("Before modification:"+str); //Before modification: CSDN_HCX change(str,"JS_HCX"); System.out.println("After modification:"+str); //After modification: CSDN_HCX } }
Exchange failure
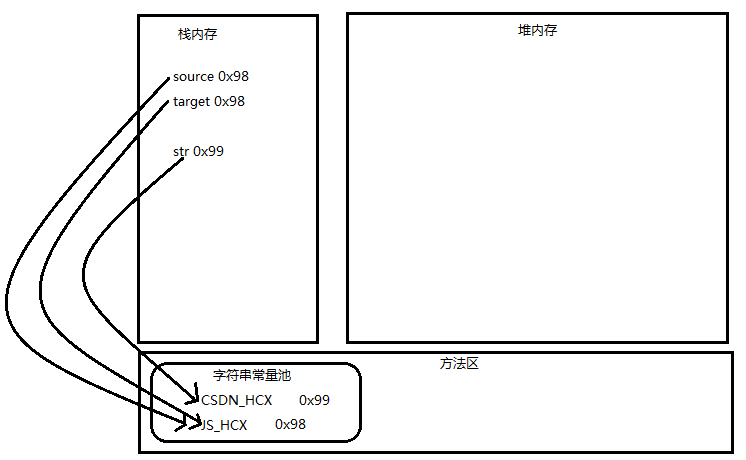