1, Variable
Variable: open up a space in memory to store the corresponding value. The value stored in this space can be changed
Variable declaration: tell the compiler that there is a variable. The compiler opens up a space in memory. If there is no assignment, the stored value is a random value
Variable declaration syntax: type variable name; such as int i; That is, one variable is I, and I is of type int
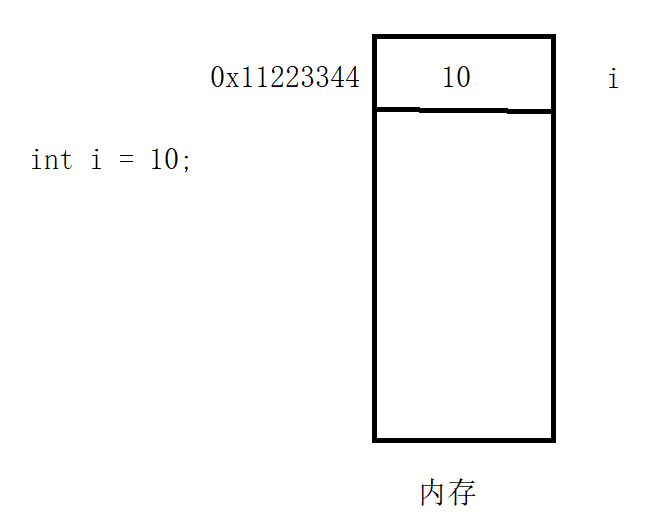
1.1 global and local variables
Global variables: variables defined outside the code block {}
Local variables: variables defined within the code block {}
#include <stdio.h> int s = 20; //Global variable s void test() { int j = 30; //Local variable j printf("Local variables: j = %d\n", j); } int main() { int i = 10; //Local variable i { int z = 40; //Local variable z printf("Local variables: z = %d\n", z); } printf("Global variables: s = %d\n", s); printf("Local variables: i = %d\n", i); test(); return 0; }
output
Local variables: z = 40 Global variables: s = 20 Local variables: i = 10 Local variables: j = 30
When the global variable has the same name as the local variable, the local variable takes precedence, so it is recommended that the name should not be the same to avoid misunderstanding
#include <stdio.h> int i = 20; //Global variable i int main() { int i = 10; //Local variable i printf("i = %d\n", i); return 0; }
output
i = 10
1.2 scope of variable
Scope: the valid range of the variable, that is, within which part of the code the variable can be used
The global variable scope is the entire project
The scope of a local variable is within the code block {} where the variable is defined
#include <stdio.h> int main() { { int i = 10; //The local variable i takes effect only within the definition code block {} } printf("%d\n", i); //The compiler will report an error. i is an undeclared variable return 0; }
a.c Documents
int num = 20;
test.c Documents
#include <stdio.h> int j = 20; extern int num; //Global variables that reference other files should be declared with the extern keyword, telling the compiler to look for num variables in other source files int main() { printf("global variable j = %d\n", j); printf("a.c Global variables for files num = %d\n", num); return 0; }
output
global variable j = 20 a.c Global variables for files num = 20
1.3 life cycle of variables
Life cycle: the life cycle of a variable refers to the time period between the creation of a variable and the destruction of a variable
The life cycle of a local variable is the beginning of the scope life cycle and the end of the scope life cycle.
The life cycle of a global variable is the life cycle of the entire program.
2, static keyword
2.1 modify global variables
Static modifies the global variable: it changes the scope of the variable, so that the static global variable can only be used inside its own source file, and cannot be used outside the source file
a.c Documents
static int num = 20; //Modify the global variable with static to make it a static global variable
test.c Documents
#include <stdio.h> extern int num; int main() { printf("a.c Global variables for files num = %d\n", num); //Error, the scope of num variable can only be in the a.c source file return 0; }
2.2 modifying local variables
static modifies local variables: it changes the life cycle of variables. The life cycle of local variables becomes longer, which is equivalent to the program life cycle
, but the local variable scope does not change
#include <stdio.h> int test() { int n = 0; //N is a local variable, which is created in function N and destroyed in function n. therefore, it is called multiple times and the return value is also 1 n++; return n; } int main() { int i = 0; for (i = 1; i < 10; i++) { printf("The first%d Call times n = %d\n", i, test()); } return 0; }
output
First call n = 1 Second call n = 1 3rd call n = 1 4th call n = 1 5th call n = 1 The 6th call n = 1 7th call n = 1 8th call n = 1 The 9th call n = 1
Use static keyword
#include <stdio.h> int test() { static int n = 0; n++; return n; } int main() { int i = 0; for (i = 1; i < 10; i++) { printf("The first%d Call times n = %d\n", i, test()); } return 0; }
output
First call n = 1 Second call n = 2 3rd call n = 3 4th call n = 4 5th call n = 5 6th call n = 6 7th call n = 7 8th call n = 8 The 9th call n = 9
2.3 modification function
Static modification function: the scope of the function is changed, so that the static function can only be used inside its own source file, and cannot be used outside the source file
a.c Documents
static int add(int x, int y){ // Modify the function with static to make it a static function return x + y; }
test.c Documents
#include <stdio.h> extern int add(int,int); int main() { int result = add(3, 5); //Error, the scope of the add function can only be used within the a.c source file printf("%d\n", result); return 0; }