This section introduces the basic usage of rollup and esbuild. We all know that the rollup package used by vite itself is consistent with the plug-in mechanism of rollup; Esbuild is used to process files in the development environment. It also has its own declaration cycle hook function. Because it is not friendly to file segmentation and css support, it has not been applied to packaging.
rollup
rollup is a mature construction tool. Open source class library is preferred. It is a construction tool aiming at esm standard, package JSON provides the module field, which is the main field when referencing, not main. commonjs is not supported by default. You need to use the plug-in resolvenode to realize the conversion. webpack is suitable for enterprise projects. Written loader s, plugins and packaging optimization
- tree shaking
rollup's favorite feature is the treeshaking function, which eliminates unused code and reduces the packaging volume
The following code
function test() { var name = 'test'; console.log(123); } const name = 'Test test'; function fn() { console.log(name); } fn();
There is no code for the test function after packaging
'use strict'; const name = 'Test test'; function fn() { console.log(name); } fn();
The implementation principle still depends on ast parsing, which is why we need to use the esm standard. When parsing, the code will be unified to the top layer of a file, and the fields of import and export will be marked, where they are defined and used. Naturally, unused will not be introduced.
Common commands
- npm i -g rollup global installation
- rollup -i index.js package index JS file to view the content on the console
- rollup -i index.js --file dist.js package file output to dist.js
- rollup -i index.js --file dist.js --format umd is packaged in umd mode,
And Iife CJS UMD mode
- rollup -i index.js a.js --dir dist is packaged into the dist directory, and the index.js is automatically generated js
- rollup -i index.js --file dist.js --format umd --name Index (--watch) can be named global ly
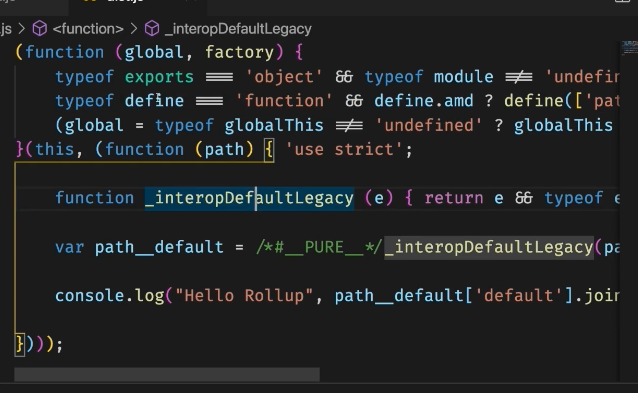
- rollup -h (--help) view command
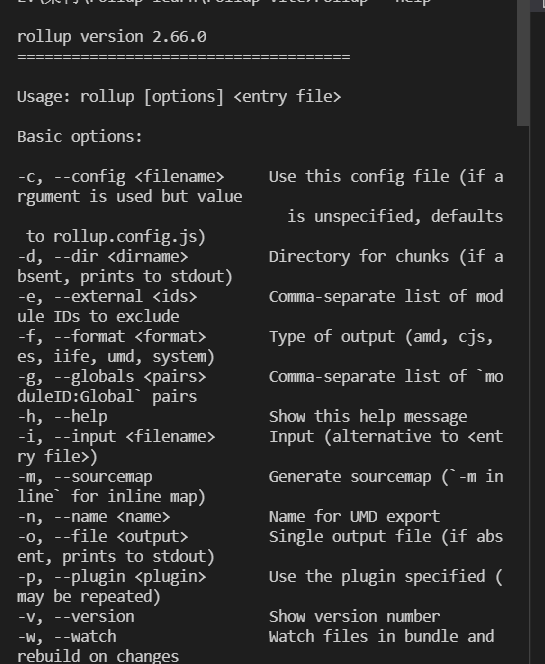
rollup.config.js
Execute the command rollup - C rollup config. js --environment TEST:123
// The command line defines environment variables, which can be obtained directly in the script console.log(process.env.TEST) export default { input: 'index.js', output: { file: 'dist.js', // The packing mode can be judged by conditions format: isLocal ? 'es' : 'umd', name: 'Index' } }
If we install rollup globally, the command line will use global rollup. If we want to use the in the project, execute node\_ Under modules:
./node_modules/.bin/rollup -c rollup.config.js --plugin json
configuration file
rollup can package not only one file, but also many kinds. Let's see the types of vue3 packaging as follows. Just export the array form in the configuration file
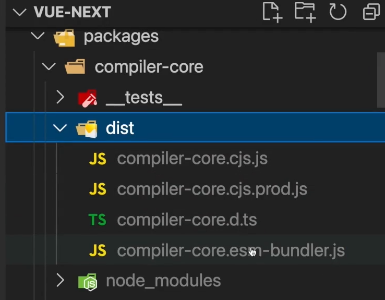
Default root directory // The module can be imported without suffix or index in the directory JS can be abbreviated improt resolve from '@rollup/plugin-node-resolve' // Convert commonjs to esm import commonjs from '@rollup/commonjs' // compress import {terser} from 'rollup-plugin-terser' export default [ { input:'index.js', // If we don't want to package them, we use cdn to import them external: ['react', { // Object requires global name 'react': 'React' }], output: { file: 'dist/index.umd.js', format: 'umd', // There are also plugin s, which can only be used after compilation and compressed plugins: [ terser() ], // Conflict with terser, because this is the content of the annotation banner: '/\*\* hellp this is banner' }, // By default, it is placed first and executed first plugins: [ resolve(), commonjs(), json() ] }, { input:'index.js', output: { file: 'dist/index.es.js', format: 'es' } } ]
The command line command defaults to global, package Default local node in JSON\_ Modules
rollup plug-in
Implementation mechanism
input -> rollup main code -> plugin1 -> plugin2 ... -> Emit file output to file - > finish
The plug-in we write is the file of the type of object to be operated. We execute different methods at different nodes according to different hook s to achieve the purpose we want
Configure which files to perform plug-in operations and which files not to perform operations. Keywords: include, exclude
- @Rollup / plugin alias setting alias
import a from '../a' // ... is cumbersome and wants to be abbreviated as follows import a from 'a' // Plug in configuration plugins: [ alias({ entries: { a: "../a",// Page/ A replace with a }, }), ],
// Finally, export a plug-in function ... export default function alias(options: RollupAliasOptions = {}) ... // Function return return { name: 'alias',// Plug in name, error report, view // inputOptions corresponds to rollup config. JS write configuration export value // Pre build processing async buildStart(inputOptions) { await Promise.all( [...(Array.isArray(options.entries) ? options.entries : []), options].map( ({ customResolver }) => customResolver && typeof customResolver === 'object' && typeof // Path mapping a/ a resolveId(importee, importer) { // Return to the real path }
Other relatively important hook functions, such as:
- transform
- Type: Function
- Form: (source, ID) = > (code, map, dependencies}, promise)
Can be used to change the code of each module. In -- watch mode, it will listen for changes to all files or directories in the dependencies array.
For example, we use replace plug-in , replace the contents of the file
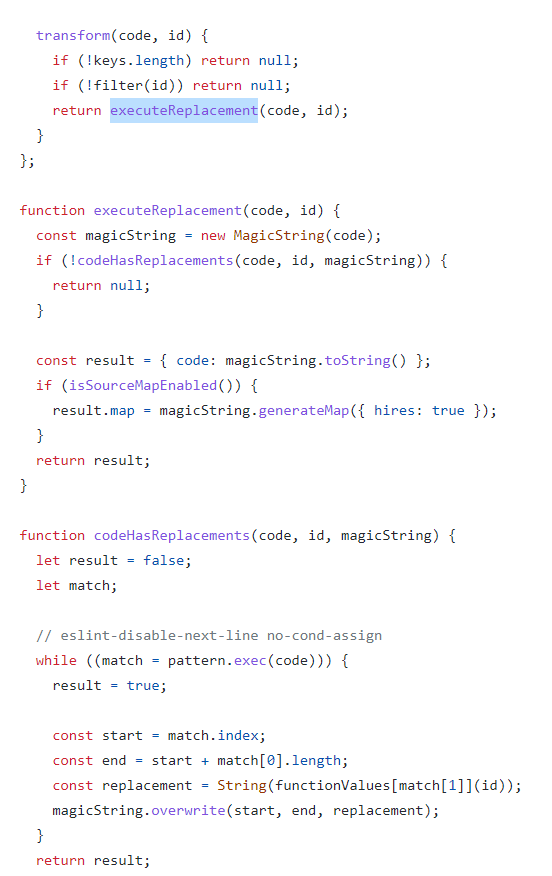
Specifically, you can go Official website learning
esbuild
Because the vite internal file compilation uses esbuild, which is written in go language and used entirely from the command line. It can parse js, but it can't run js. The code in the node tool is also executed indirectly through the command line. We need to understand the plug-in execution mechanism of esbuild. When writing vite plug-ins, if it is necessary to write our own plug-ins, we should also be compatible with rollup and esbuild
use
npx esbuild index.js
npx esbuild index.js --ourfile=dist.js output
If you want to package (all code is packaged together), add -- bundle, and there is treeshaking inside
--target=esnext specifies the compilation target. Note that the compilation of es5 by esbuild is incomplete, and const will still be va
--platform=node, browser node environment or browser environment
--format=esm cjs
--watch
--define:TEST=12 environment variable
If pictures are used in the project, you need to use load
import a from './logo.png'
--loader:.png=dataurl / / converted to base64
esbuild plug-in
In esbuild, the plug-in is designed as a function, which needs to return an Object, which contains two attributes such as name and setup
The build object will expose two very important functions in the whole construction process: onResolve and onLoad. Both of them need to pass in two parameters, such as Options and CallBack.
// build.js let exampleOnLoadPlugin = { name: "example", // Name for error warning prompt setup(build) { let fs = require("fs"); // Entered options console.log(build.initialOptions); // We can modify the configuration build initialOptions. outdir = 'lib' /\*\* build.onStart({}, () => { // You can no longer modify the configuration in onstart }) \*/ // What kind of files are processed build.onResolve( { filter: /\.txt$/, }, async (args) => ({ path: args.path, namespace: "txt", // Distinguish file loading and identification }) ); build.onLoad( { // Execute regular in go filter: /\.\*$/, //What type of file execution namespace: "txt", }, async (args) => { // File path of the module let text = await fs.promises.readFile(args.path, "utf-8"); return { // File content preprocessing contents: `export default ${JSON.stringify([ ...text.split(/\s+/), "----------", ])}`, // loader: "json", }; } ); }, }; require("esbuild") .build({ // Command line parameters entryPoints: ["index.js"], bundle: true, outdir: "dist", loader: { ".png": "dataurl", }, plugins: [exampleOnLoadPlugin], }) .catch(() => process.exit(1));
Callback function of onResolve
The callback function of onResolve function will be executed when esbuild builds the import path (matching) of each module.
The callback function of onResolve function needs to return an object, which will contain attributes such as path, namespace and external.
Usually, this callback function will be used to customize the way esbuild handles path, for example:
Rewrite the original path, such as redirecting to another path
Mark the module corresponding to the path as external, that is, the modified file will not be built (output as is)
Callback function of onLoad
The callback function of the onLoad function will be called before the esbuild parsing module, mainly for processing and returning the contents of the module, and telling esbuild how to parse them. Also, it should be noted that the onload callback function does not handle modules marked external.
The callback function of onLoad function needs to return an object with a total of 9 properties. Here, let's take a look at two common attributes:
Contents processed module contents
The default is to tell the loader how to build the content. For example, if the returned module content is CSS, declare the loader as CSS
This section introduces the common usage of rollup and esbuild, including the use of common commands and plug-ins. The next section will introduce the learning and use of vite plug-in. If you have any questions, please leave a message. Thank you for reading!