console.log('Hello Node.js')
Open command line terminal: Ctrl + Shift + y
Go to the directory where the program is located, enter
node 01.js
The browser's core consists of two parts:
-
DOM rendering engine;
-
JS parser (js engine)
-
js runs inside the js engine in the browser's kernel
Node.js is a JavaScript program that runs out of the browser environment, based on the V8 engine (the JavaScript engine for Chrome)
3. Server-side Application Development (Understanding)
Create 02-server-app.js
const http = require('http'); http.createServer(function (request, response) { // Send HTTP Header // HTTP status value: 200: OK // Content type: text/plain response.writeHead(200, {'Content-Type': 'text/plain'}); // Send response data "Hello World" response.end('Hello Server'); }).listen(8888); // The terminal prints the following information console.log('Server running at http://127.0.0.1:8888/');
Run Server Programs
node 02-server-app.js
After the server starts successfully, type in the browser: http://localhost:8888/ View webserver running successfully and output html page
Stop service: ctrl + c
NPM
=======
1. Introduction to npm
The full name of NPM, Node Package Manager, is Node. The JS package management tool is the largest module ecosystem in the world, where all modules are free from open source. It's also Node.js package management tool, equivalent to the front-end Maven.
1. Installation location of NPM tools
npm makes it easy to download js libraries and manage front-end projects.
Node. Location of npm packages and tools installed by default for js: Node.js directory\node_modules
- In this directory you can see the npm directory, which is itself a tool managed by the npm package manager, describing Node.js has integrated npm tools
#Enter npm-v at the command prompt to view the current NPM version npm -v
2. Use npm to manage projects
1. Create folder npm
2. Project Initialization
#Create an empty folder and enter it at the command prompt to perform command initialization npm init #Enter relevant information as prompted, or return directly if default value is used. #Name:Project name #Version: Project version number #description:Project description #keywords: {Array} keywords to make it easy for users to search for our items #The package is generated. JSON file, this is the package's configuration file, which is equivalent to maven's pom.xml #We can then make changes as needed.
#If you want to generate the package directly. JSON file, then you can use the command
npm init -y
### 3. Modify npm image NPM Officially managed packages are from [http://npmjs.com](https://gitee.com/vip204888/java-p7) Downloaded, but the website is very slow in China. Taobao is recommended here NPM image [http://npm.taobao.org/](https://gitee.com/vip204888/java-p7) The Taobao NPM image is a complete npmjs.com mirror, the synchronization frequency is currently 10 minutes to ensure maximum synchronization with official services. **Set mirror address:**
#After the following configuration, all NPM installs will be downloaded from Taobao's mirror address in the future
npm config set registry https://registry.npm.taobao.org
#View npm configuration information
npm config list
### 4. **Use of npm install command**
#Use npm install to install the latest version of dependent packages,
#Module Installation Location: Project Directory\node_modules
#Installation automatically adds package-lock to the project directory. JSON file, which helps lock the version of the installation package
#Simultaneous package. In the JSON file, dependent packages are added under the dependencies node, similar to those in maven
npm install jquery
#npm managed items generally do not carry node_when backing up and transferring Modules Folder
npm install #according to package. Configuration download dependency in json, initializing project
#If you want to specify a specific version at installation time
npm install jquery@2.1.x
#devDependencies node: Dependency packages for development, dependencies not included when a project is packaged into a production environment
#Add dependencies to the devDependencies node using the -D parameter
npm install --save-dev eslint
#or
npm install -D eslint
#Global Installation
#Node. Location of NPM packages and tools installed globally by js: User Directory\AppData\Roaming\npm\node_modules
#Some command-line tools often use a global installation
npm install -g webpack
### 5. Other Commands
#Update Package (Update to Latest Version)
npm update package name
#Global Update
NPM update-g package name
#Uninstall Package
npm uninstall package name
#Global Uninstall
NPM uninstall-g package name
* * * **Babel** ========= I. Babel brief introduction --------- Babel Is a widely used transcoder that allows ES6 Code to ES5 Code to execute in an existing environment. That means you can use it now ES6 Write a program without worrying about whether the existing environment supports it. 2. Installation ----
npm install --global babel-cli
#Check to see if the installation was successful
babel --version
3. Babel Use ---------- ### 1. Initialize Project
npm init -y
### 2. Create Files src/example.js Here is a paragraph ES6 Code:
//before transcoding
//Define data
let input = [1, 2, 3]
//Add each element of the array+1
input = input.map(item => item + 1)
console.log(input)
### 3. Configuration. babelrc Babel The configuration file is.babelrc,Stored in the project's root directory, this file is used to set transcoding rules and plug-ins in the following basic format.
{
"presets": [], "plugins": []
}
presets Fields set transcoding rules that will es2015 Rule join .babelrc:
{
"presets": ["es2015"], "plugins": []
}
### 4. Install Transcoder
npm install --save-dev babel-preset-es2015
### 5. Transcoding
Transcoding results are written to a file
mkdir dist1
- out-file or -o parameter specifies output file
babel src/example.js --out-file dist1/compiled.js
perhaps
babel src/example.js -o dist1/compiled.js
Entire Catalog Transcoding
mkdir dist2
- out-dir or-d parameter specifies output directory
babel src --out-dir dist2
perhaps
babel src -d dist2
* * * **Webpack** =========== I. Webpack brief introduction ----------- Webpack Is a Front End Resource Load/Packaging tool. It performs a static analysis based on the dependencies of the modules, then generates the corresponding static resources for the modules according to the specified rules. As we can see from the picture, Webpack Multiple static resources can be used js,css,less Converting to a static file reduces page requests. 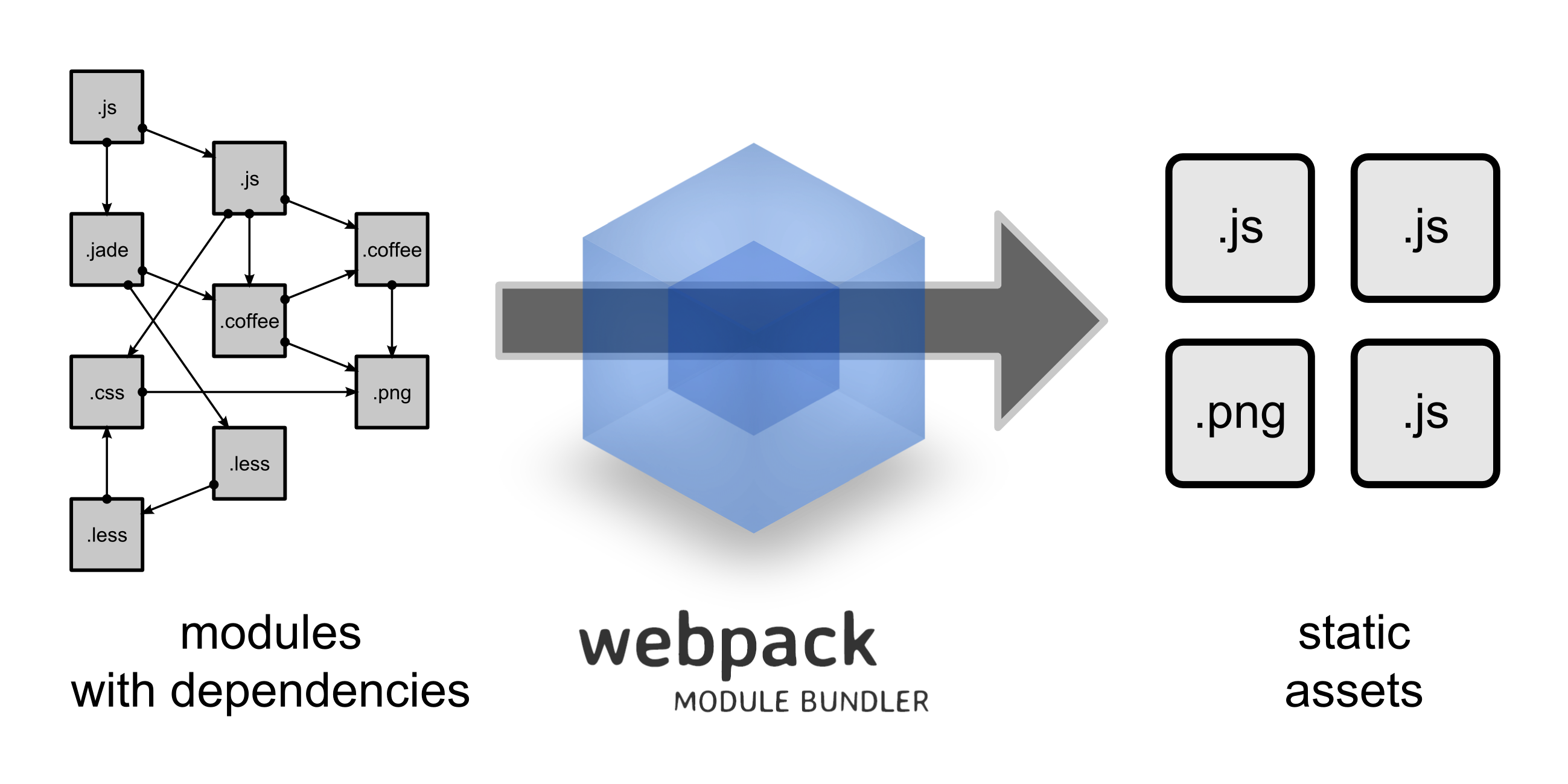 2. Webpack install ------------ ### 1. Global Installation
npm install -g webpack webpack-cli
### 2. View version number after installation
webpack -v
3. Initialization Project ------- ### 1. Create a webpack folder Get into webpack Directory, Execute Command
npm init -y
### 2. Create src folder ### 3. ** under **src **** create common.js**
exports.info = function (str) {
document.write(str);
}
### 4. ** Create utils under src. Js**
exports.add = function (a, b) {
return a + b;
}
### 5. ** Create main under src. Js**
const common = require('./common');
const utils = require('./utils');
common.info('Hello world!' + utils.add(100, 200));
IV. JS Pack ------ ### 1. Create a configuration file webpack in the webpack directory. Config. JS The following configuration means: Read the current project directory src In folder main.js(Entry File) Content, analyze resource dependencies, put relevant js Files are packaged and placed in the current directory dist Folder, packaged js File name bundle.js
const path = require("path"); // Node.js built-in module
module.exports = {
entry: './src/main.js', //Configuration Entry File output: { path: path.resolve(__dirname, './dist'), //Output Path, u dirname: The path to the current file filename: 'bundle.js' //output file }
}
### 2. ** Command line executes compile command**
webpack #has yellow warning
webpack --mode=development #No warnings
#View bundles after execution. js contains the contents of the above two js files and wakes up code compression
You can also configure the npm Run commands, modify package.json file
"scripts": {
//..., "dev": "webpack --mode=development"
}
Function npm Command Execution Packaging
npm run dev
### 3. Create an index in the webpack directory. HTML # Knowing what it is, the factory often asks how to review the interview technology? **1,Top interview questions and answers** Do your best before the interview to improve your interview success rate, here is a hot 350 front-line Internet frequently asked interview questions and answers to help you take offer > [**Haldane's Best Answers to Tough Interview Questions+book+Core knowledge acquisition: Stamp here for free download**](https://gitee.com/vip204888/java-p7)! With sincerity!!! 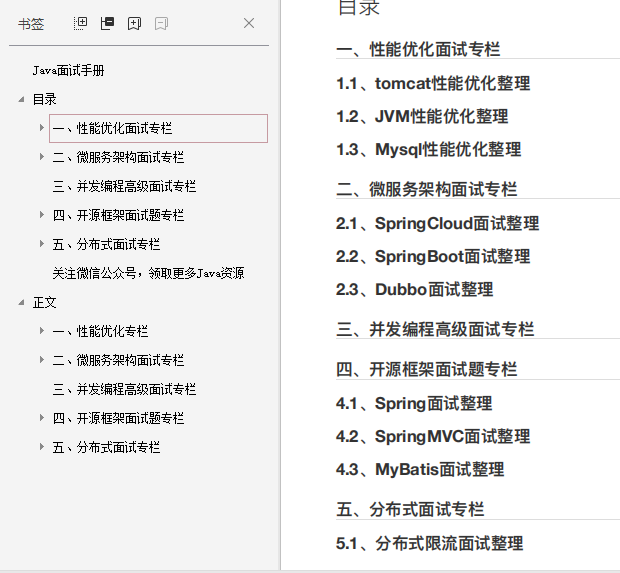 **2,Multi-threaded, high concurrency, caching entry to real-world projects pdf book** 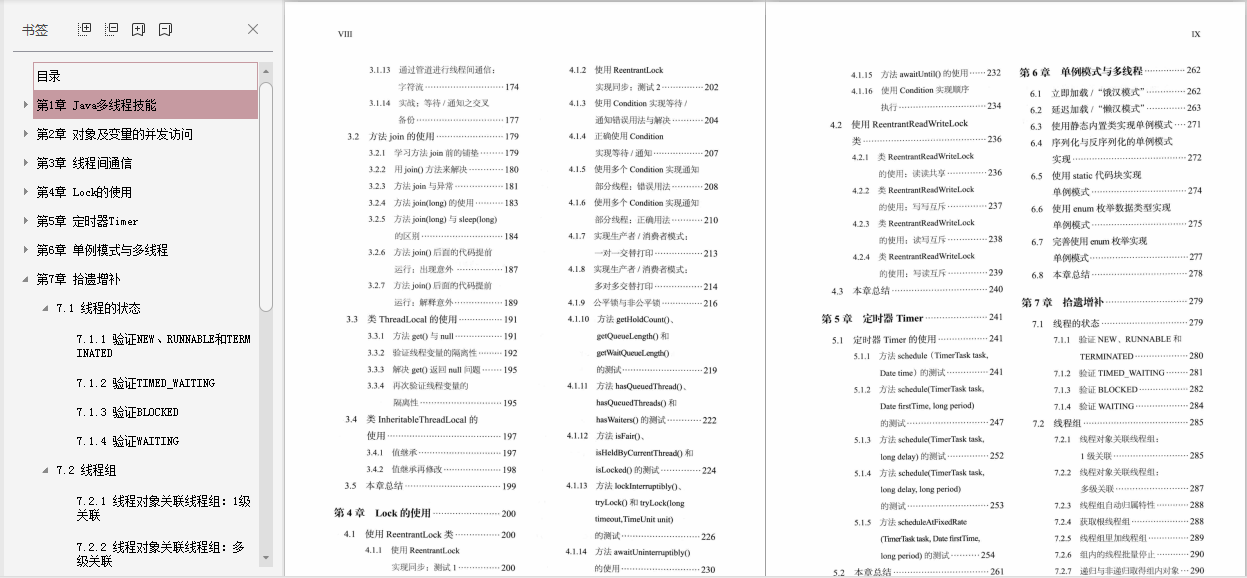 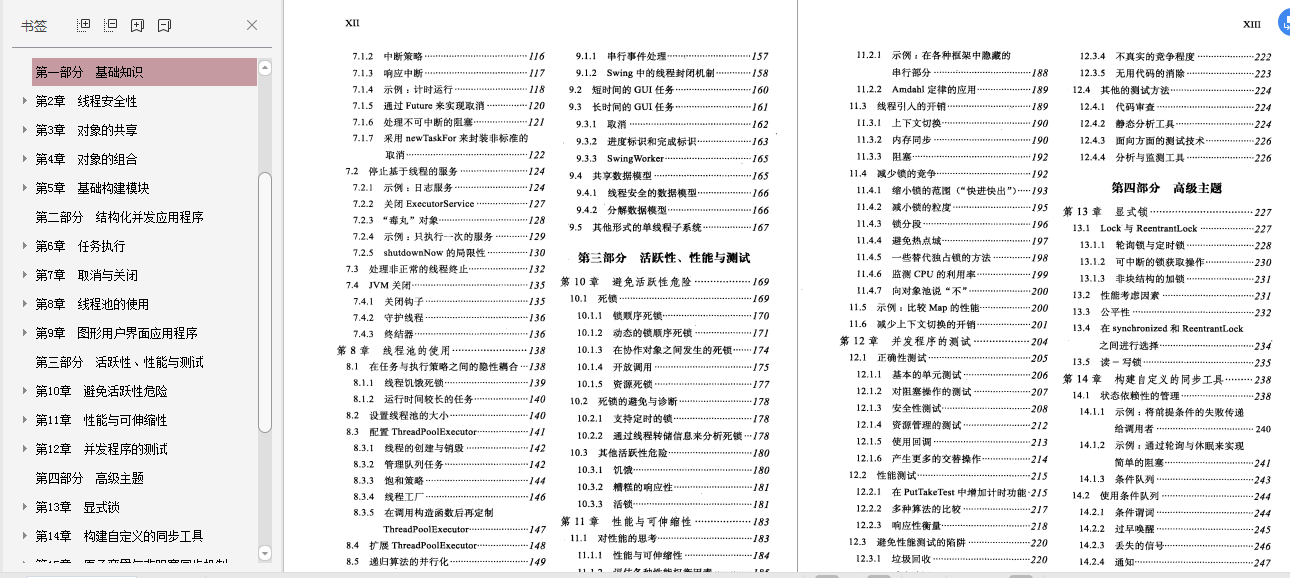 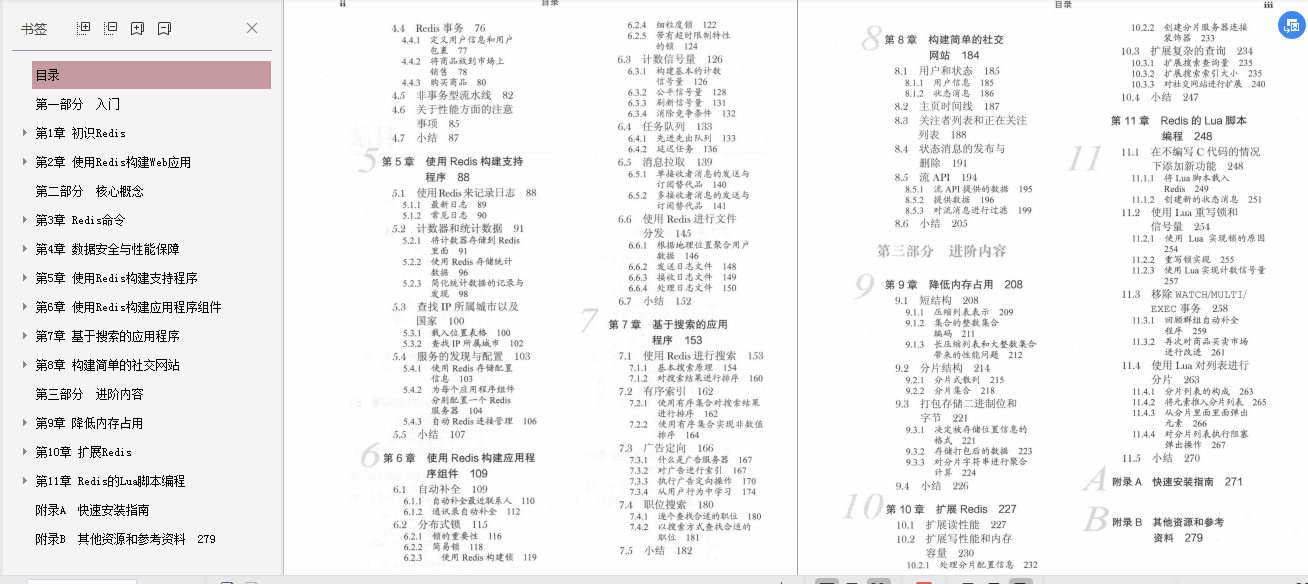 **3,Answer arrangement for interview questions mentioned in the article** 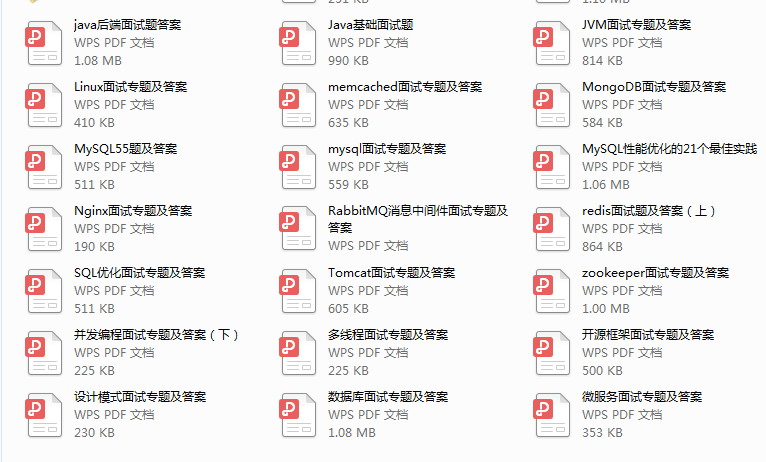 **4,Java Core Knowledge Interview Treasure** Covered**JVM ,JAVA Collection, JAVA Multithreaded concurrency, JAVA Base, Spring Principles, Micro Services, Netty and RPC,Network, Log, Zookeeper,Kafka,RabbitMQ,Hbase,MongoDB ,Cassandra,Design patterns, load balancing, databases, consistency algorithms, JAVA Algorithm, data structure, algorithm, distributed cache, Hadoop,Spark,Storm Numerous technical points and in-depth explanations** 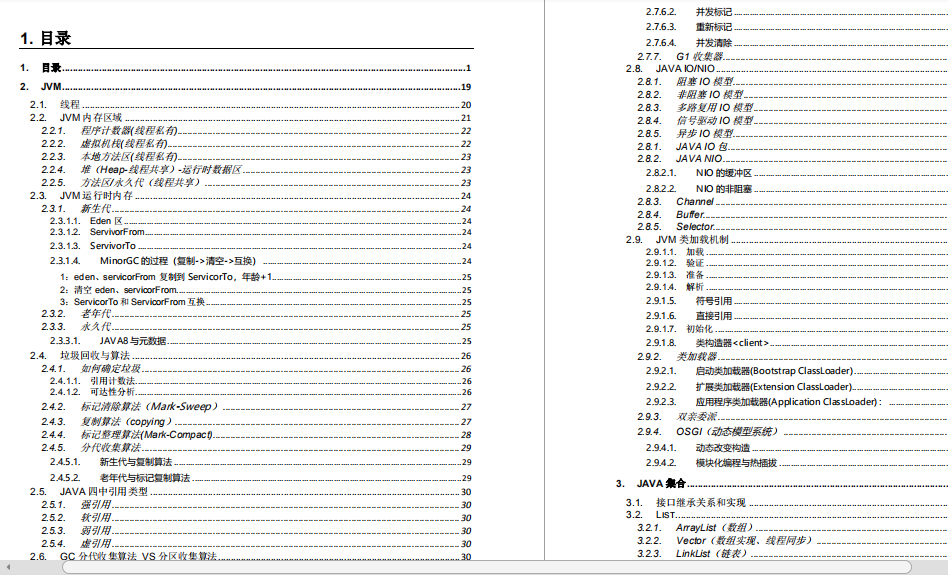 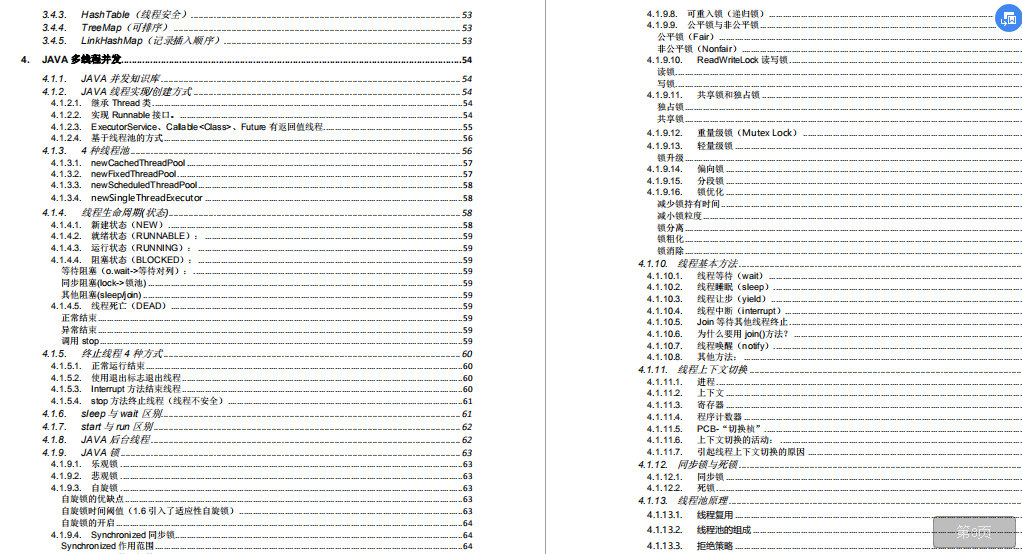 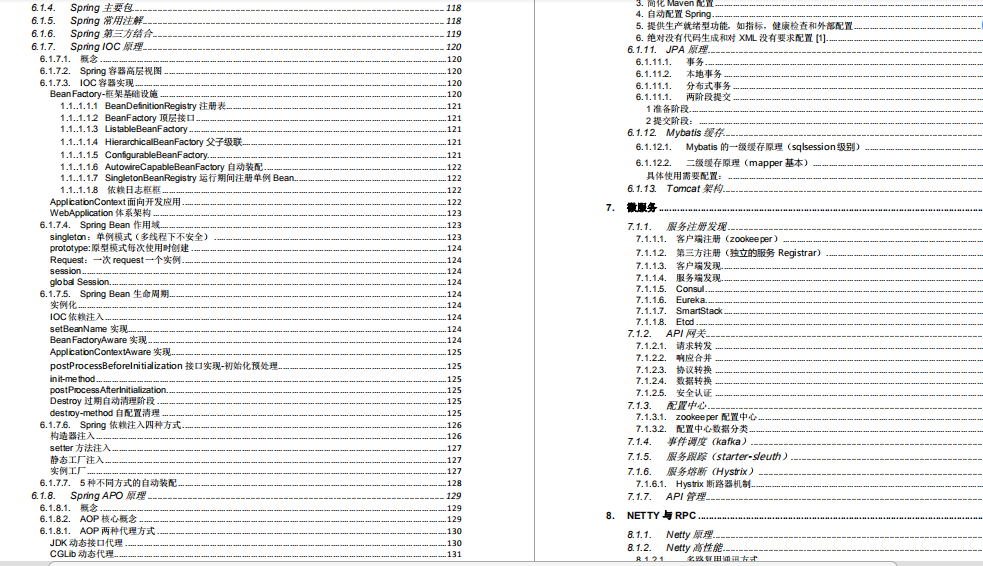 A Top 350 Front-line Internet Frequently Asked Questions and Answers Help You Get offer > [**Haldane's Best Answers to Tough Interview Questions+book+Core knowledge acquisition: Stamp here for free download**](https://gitee.com/vip204888/java-p7)! With sincerity!!! [Outer Chain Picture Transfer in Progress...(img-WxXuPzFJ-1628600974516)] **2,Multi-threaded, high concurrency, caching entry to real-world projects pdf book** [Outer Chain Picture Transfer in Progress...(img-6sjeo0uz-1628600974519)] [Outer Chain Picture Transfer in Progress...(img-pHGFI3DL-1628600974522)] [Outer Chain Picture Transfer in Progress...(img-QFwldkDV-1628600974524)] **3,Answer arrangement for interview questions mentioned in the article** [Outer Chain Picture Transfer in Progress...(img-I2lUVVb5-1628600974526)] **4,Java Core Knowledge Interview Treasure** Covered**JVM ,JAVA Collection, JAVA Multithreaded concurrency, JAVA Base, Spring Principles, Micro Services, Netty and RPC,Network, Log, Zookeeper,Kafka,RabbitMQ,Hbase,MongoDB ,Cassandra,Design patterns, load balancing, databases, consistency algorithms, JAVA Algorithm, data structure, algorithm, distributed cache, Hadoop,Spark,Storm Numerous technical points and in-depth explanations** [Outer Chain Picture Transfer in Progress...(img-xxvXv4aL-1628600974527)] [Outer Chain Picture Transfer in Progress...(img-AKeZJJtj-1628600974528)] [Outer Chain Picture Transfer in Progress...(img-znd3mW1X-1628600974529)]