demand
The previous chapter introduced the new functions of data in list operation. This chapter looks at the function of deleting data.
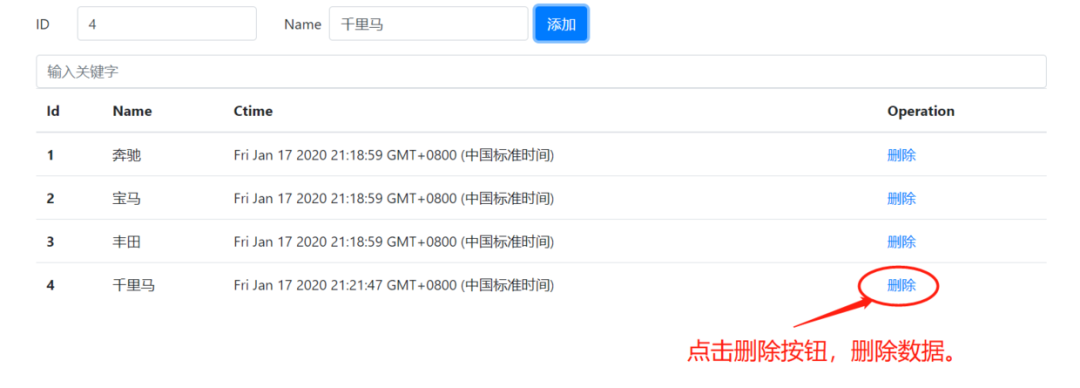
thinking
If you want to delete the data in the list, how do you delete it?
- Deleting data needs to be based on the id number of the data, and the id of the data needs to be passed to the deletion method
- According to the id, find the array index to delete
- If the index index is found, directly call the slice (index, 1) method of the array to delete the data
Example code
Before writing the deletion method, provide sample code for readers to read, as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <!-- 2.Import bootstrap library --> <link rel="stylesheet" type="text/css" href="lib/bootstrap4/bootstrap.min.css"> <script type="text/javascript" src="lib/bootstrap4/popper.min.js"></script> <script type="text/javascript" src="lib/bootstrap4/bootstrap.min.js"></script> </head> <body> <div id="app"> <div class="container"> <!-- Search condition start --> <div class="row mt-2"> <!-- Enter to add data id --> <div class="form-group row"> <label for="input_id" class="col-sm-2 col-form-label">ID</label> <div class="col-sm-10"> <input type="text" class="form-control" id="input_id" placeholder="ID" v-model="id"> </div> </div> <!-- Enter to add data name --> <div class="form-group row ml-3"> <label for="input_name" class="col-sm-2 col-form-label">Name</label> <div class="col-sm-10"> <input type="text" class="form-control" id="input_name" placeholder="Name" v-model="name"> </div> </div> <!-- add button --> <input type="button" value="add to" class="btn btn-primary ml-2 mb-3" @click="add"> <!-- Search element keyword --> <input type="text" class="form-control" id="input_keywords" placeholder="Enter keywords"> </div> <!-- Search condition end --> <!-- table list start--> <div class="row"> <table class="table"> <thead> <tr> <th scope="col">Id</th> <th scope="col">Name</th> <th scope="col">Ctime</th> <th scope="col">Operation</th> </tr> </thead> <tbody> <!-- use v-for Traverse the data and set key Ensure component uniqueness --> <tr v-for="item in list" :key="item.id"> <th scope="row">{{ item.id }}</th> <td>{{ item.name }}</td> <td>{{ item.ctime }}</td> <td><a href="#"> delete</a></td> </tr> </tbody> </table> </div> <!-- table list end--> </div> </div> <script> // 2. Create an instance of Vue var vm = new Vue({ el: '#app', data: { id: '', name: '', // Set the data list of the list list: [ { id: 1, name: 'Benz', ctime: new Date() }, { id: 2, name: 'bmw', ctime: new Date() }, { id: 3, name: 'Toyota', ctime: new Date() }, ], }, methods:{ add(){ // Add data to list array this.list.push({ id: this.id, name: this.name, ctime: new Date() }); } } }) </script> </body> </html>
Method for implementing deletion
1. Deleting data needs to be based on the id number of the data, and the id of the data needs to be passed to the deletion method
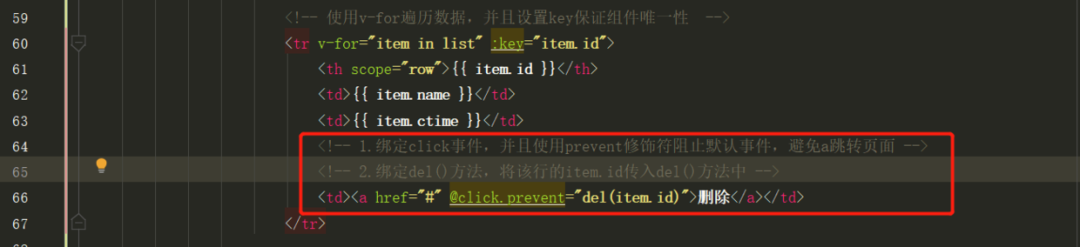
2. Find the array index to delete according to the id
If you already have the id number in the array list, you can query the index index of the array according to this id number.
Based on ES6, several new methods are provided so that the array can define the index according to the value, such as some findIndex. Let's start with a simple completion example.
2.1 use the some method to traverse the array. When return true, the loop will be terminated
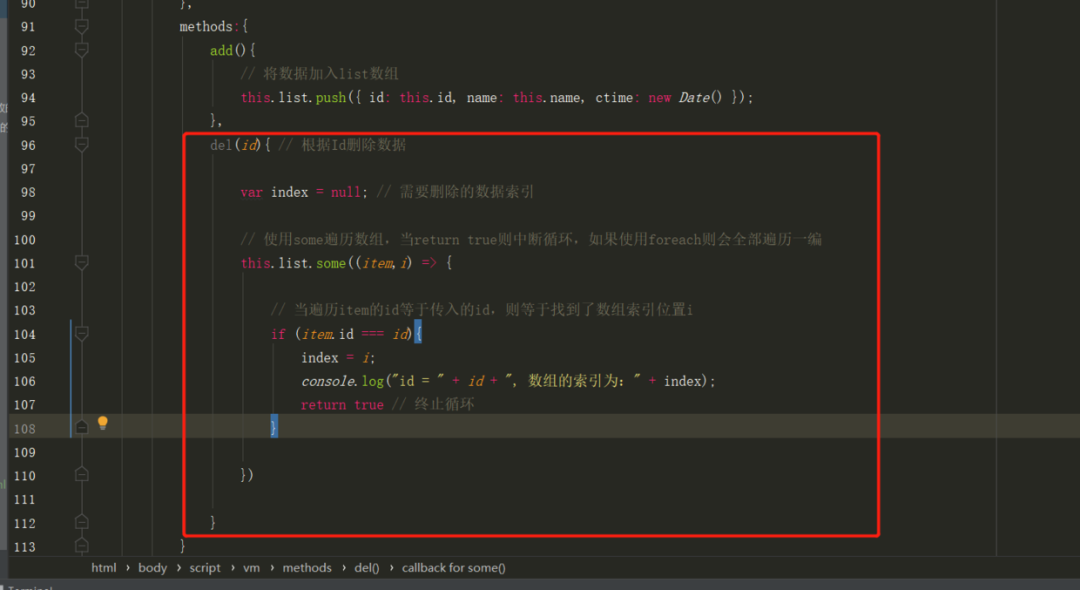
Click "delete" in the browser to view the printed array index, as follows:
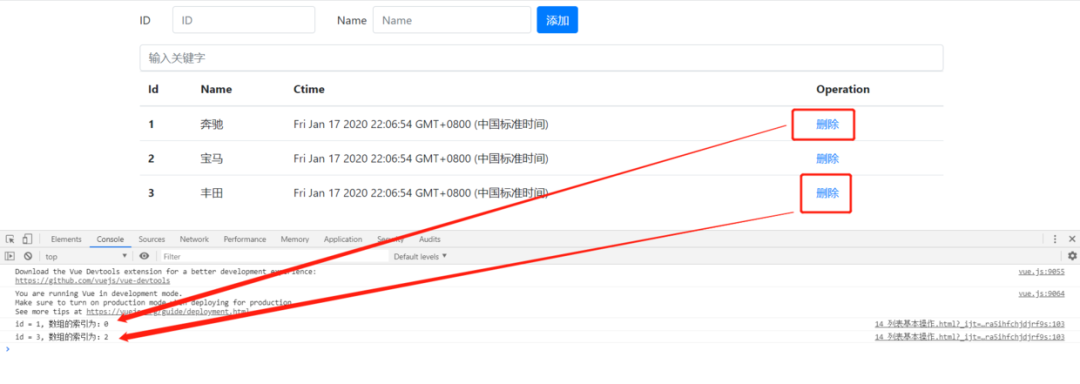
Let's look at the findIndex method to locate the index of the array.
2.2 locate the index of the array using the findIndex method
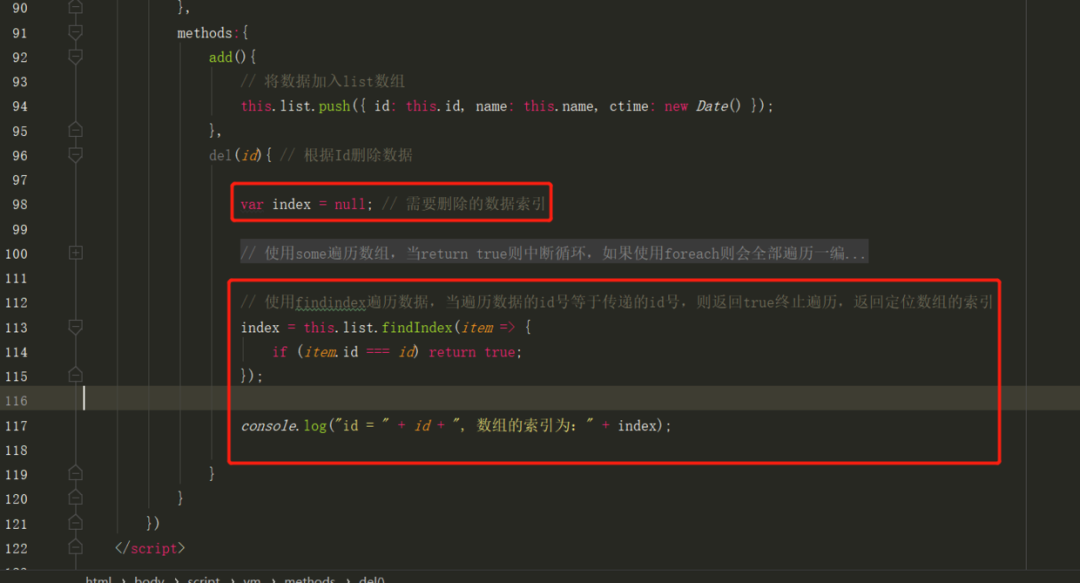
Click "delete" in the browser to view the printed array index, as follows:
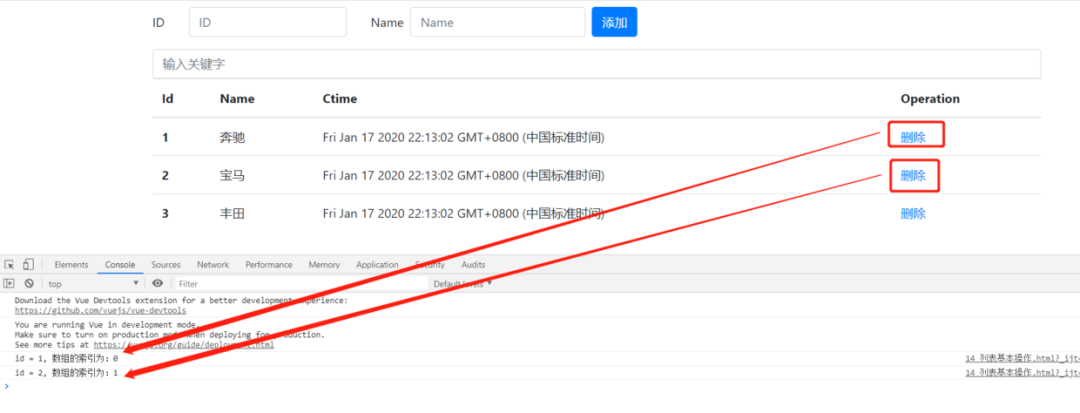
It can be seen that the findIndex method directly returns the index of the termination location.
3. Directly call the slice (index, 1) method of the array using the index index to delete the number
Delete the data according to the index found above, as follows:
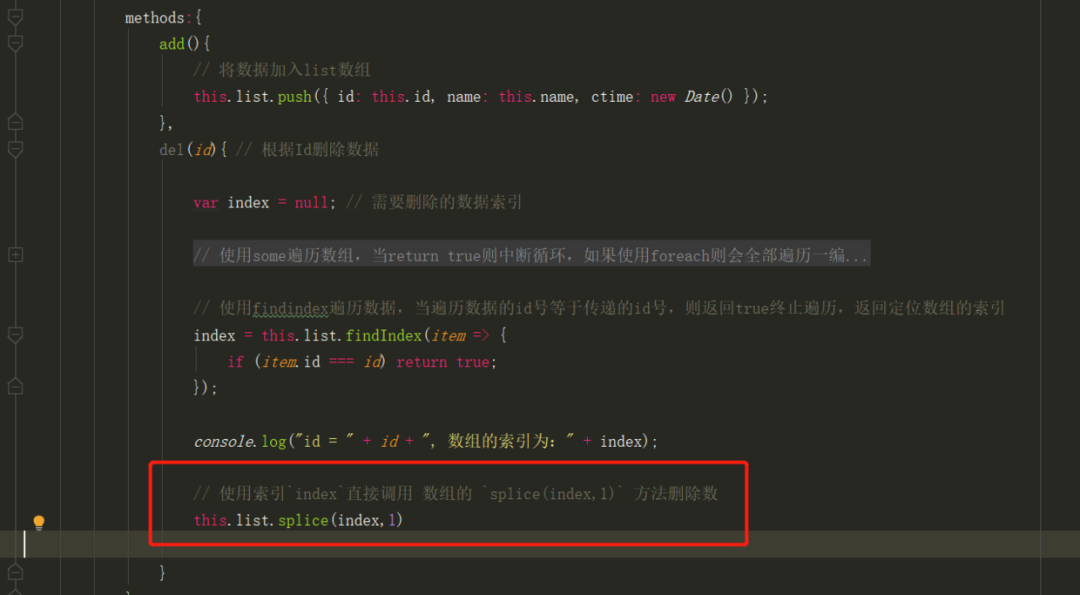
The browser performs the deletion as follows:
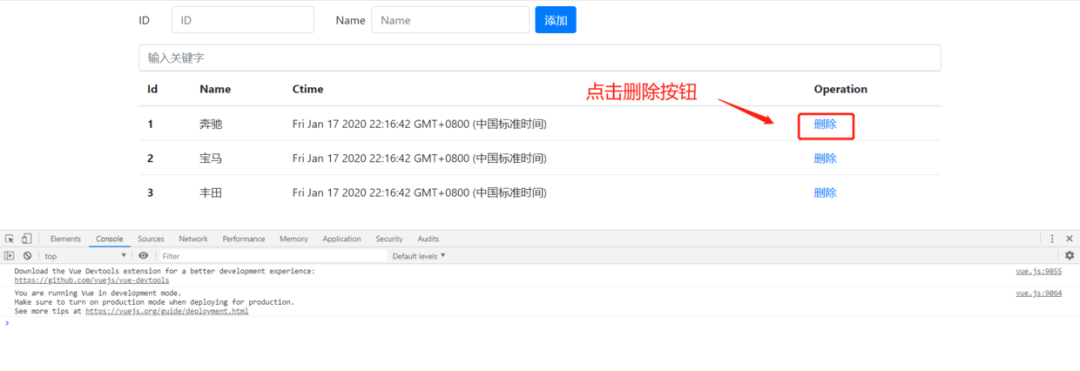
