summary
Vue provides application transition effects in many different ways when inserting, updating or removing DOM. Includes the following tools:
- Automatically apply class in CSS transitions and animations
- You can use third-party CSS animation libraries, such as animate css
- Use JavaScript in the transition hook function to directly manipulate DOM
- You can use third-party JavaScript animation libraries, such as velocity js
The previous article explained the use of "transition class name" to achieve animation effect, but it is actually a troublesome thing if each animation has to be written by itself. This chapter explains the use of the third-party css animation library "Animate.css" to achieve animation effect.
Animate. Introduction to CSS Library
brief introduction
animate.css is a CSS3 animation library from abroad. It preset more than 60 kinds of animation effects, such as shake, flash, bounce, flip, rotateIn/rotateOut, fadeIn/fadeOut, and almost all common animation effects.
Although with the help of animate CSS can easily and quickly make CSS3 animation effects, but it is recommended to take a look at animation CSS code, maybe you can learn something from it.
Relevant website
animate.css Chinese website: http://www.animate.net.cn/
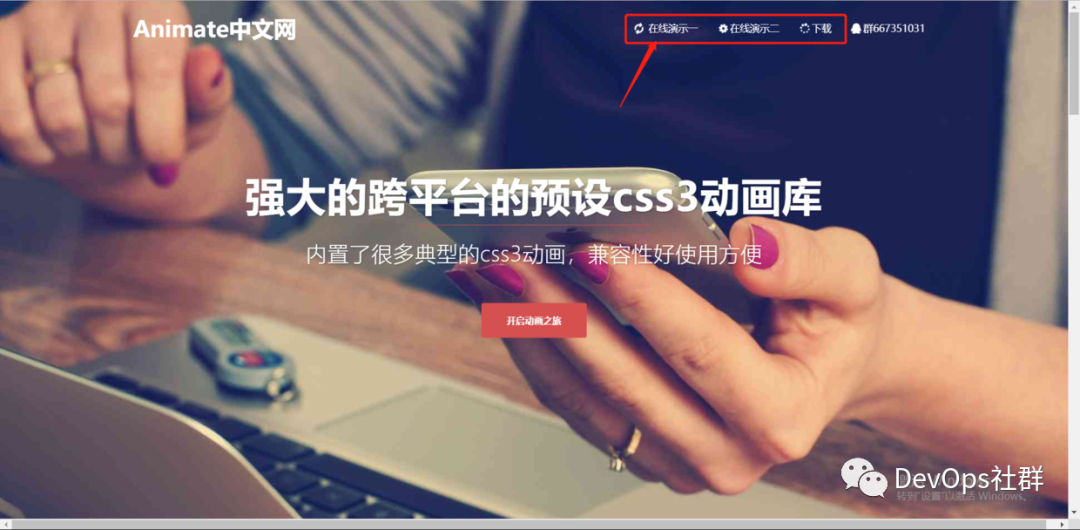
image-20200131234307872
After entering the Animate Chinese website, you can view some online demonstrations.
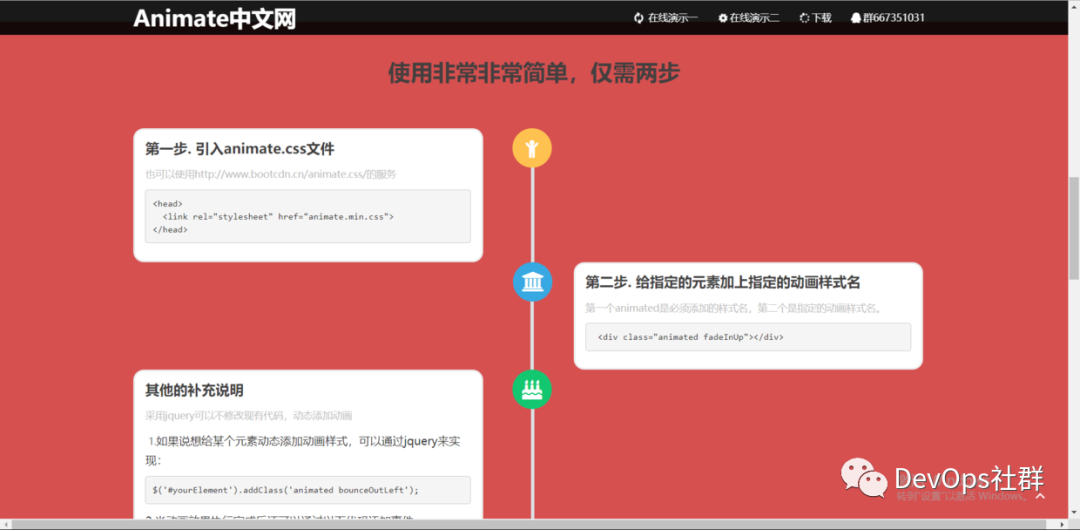
You can see the introduction. Using the animate library is very simple. Let's take a look at how to use it.
Download the animation library
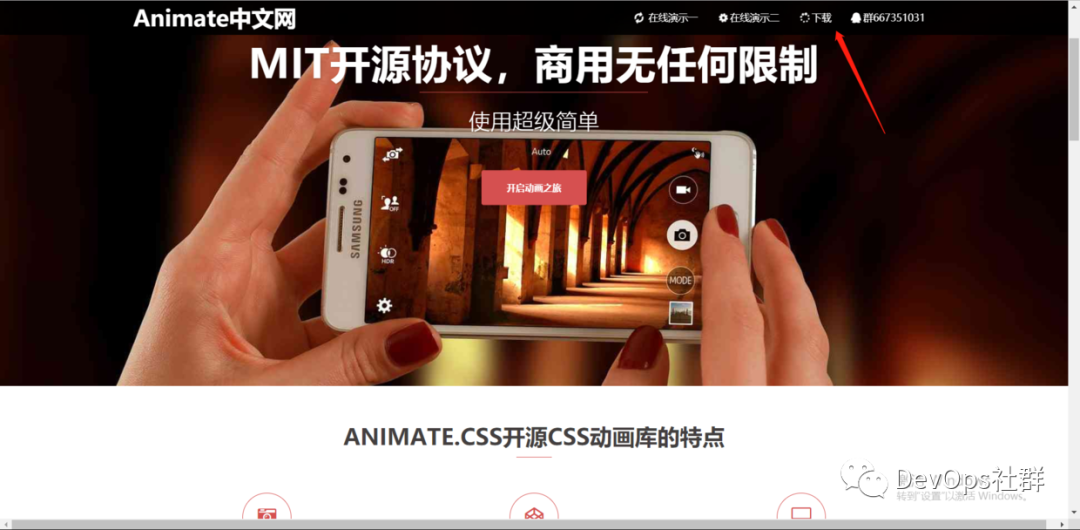
Download address: https://daneden.github.io/animate.css/
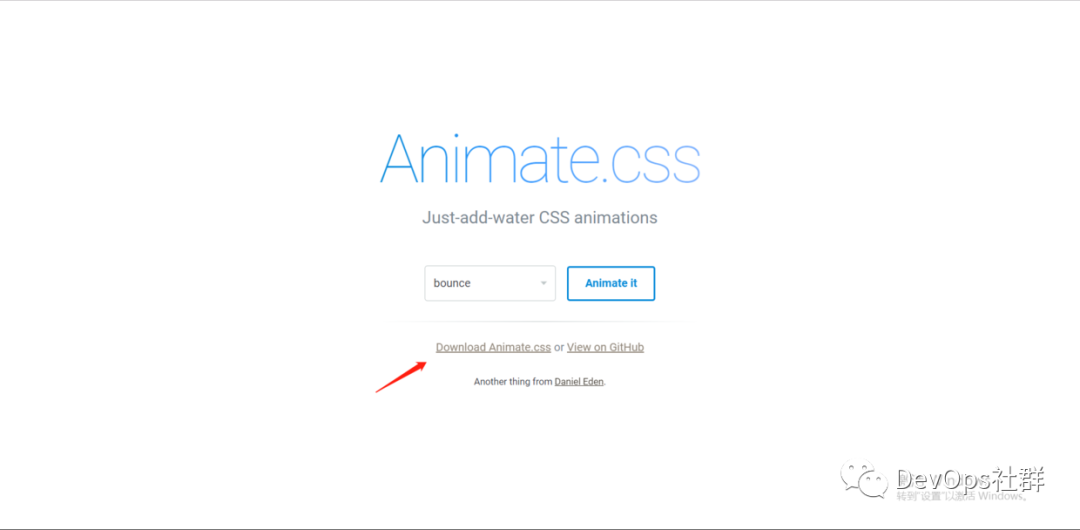
If I directly click this address to download, I will fail to visit the page at present. Then I silently went to the release page in Github to download it.
https://github.com/daneden/animate.css/releases
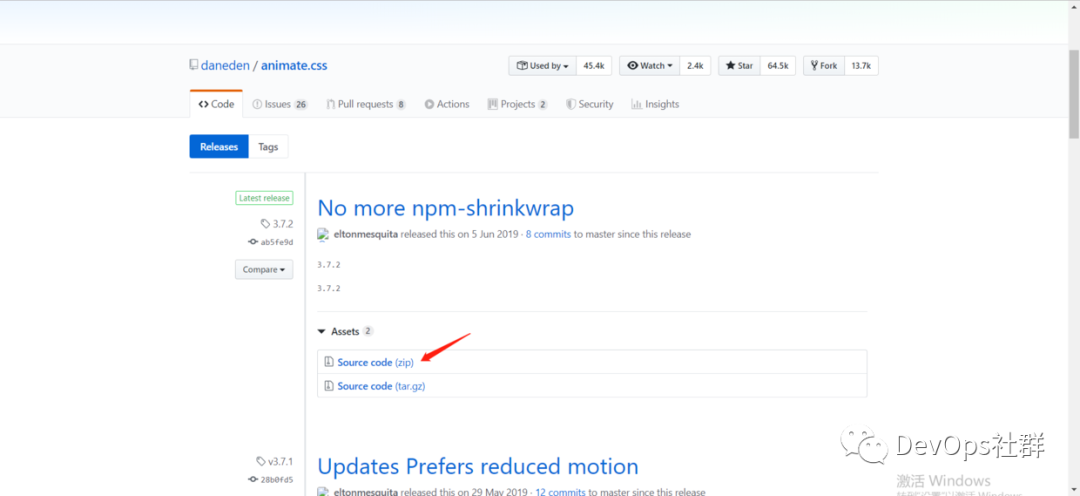
Unzip the downloaded zip package, and you can see animate CSS related files:
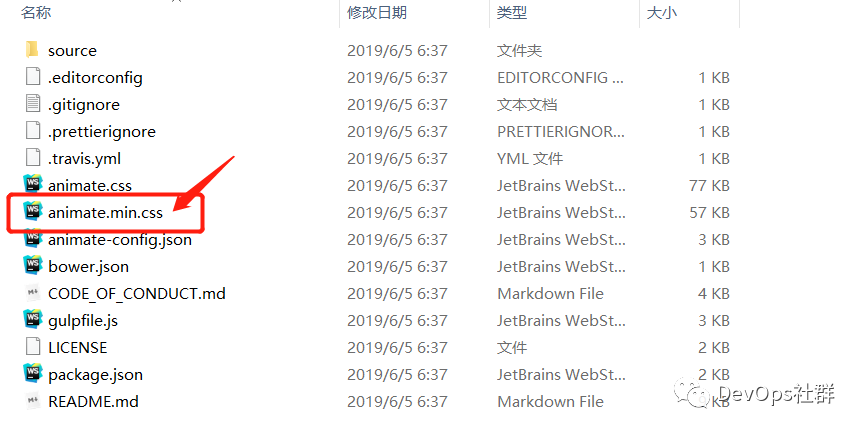
In project development, you only need to import the animation Just compress the min.css file.
Use example 1
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <!-- First step. introduce animate.css file --> <link rel="stylesheet" href="lib/animate.css-3.7.2/animate.min.css"> </head> <body> <!-- use animate Library fadeInUP Animation effect --> <div class="animated fadeInUp"> <h1>hello world</h1> </div> </body> </html>
The execution effect of the browser is as follows:
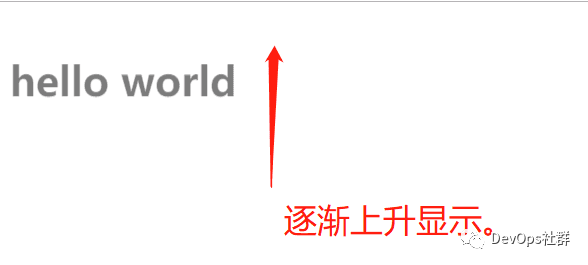
After the above example adds class to the element and refreshes the page, you can see the animation effect. animated is similar to global variable, which defines the duration of animation; bounce is the name of the specific animation effect of the animation. You can choose any effect.
If the animation is played infinitely, you can add class infinite as follows:
<!-- use animate Library fadeInUP Animation effect --> <div class="animated fadeInUp infinite"> <h1>hello world</h1> </div>
Use example 2
You can also add these class es to elements through JavaScript or jQuery, such as:
$(function(){ $('#dowebok').addClass('animated bounce'); });
Some animation effects will eventually make the elements invisible, such as fading out, sliding left, etc. you may need to delete the class, such as:
$(function(){ $('#dowebok').addClass('animated bounce'); setTimeout(function(){ $('#dowebok').removeClass('bounce'); }, 1000); });
animate. The default settings of CSS may not be what we want sometimes, so you can reset them, such as:
#dowebok { animate-duration: 2s; //Animation duration animate-delay: 1s; //Animation delay time animate-iteration-count: 2; //Animation execution times }
Note that the browser prefix is added.
-ms- compatible IE browser -moz- compatible firefox -o- compatible opera -webkit- compatible chrome and safari
The complete example code is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <!-- First step. introduce animate.css file --> <link rel="stylesheet" href="lib/animate.css-3.7.2/animate.min.css"> <!-- to write js control animate Animation class --> <script src="lib/jquery/jquery-3.4.1.min.js"></script> <script> $(function(){ $('#dowebok').addClass('animated bounce'); setTimeout(function(){ $('#dowebok').removeClass('bounce'); }, 1000); }); </script> </head> <body> <!-- use js control animated Animation class for --> <div id="dowebok"> <h1>Bounce</h1> </div> </body> </html>
The browser displays as follows:
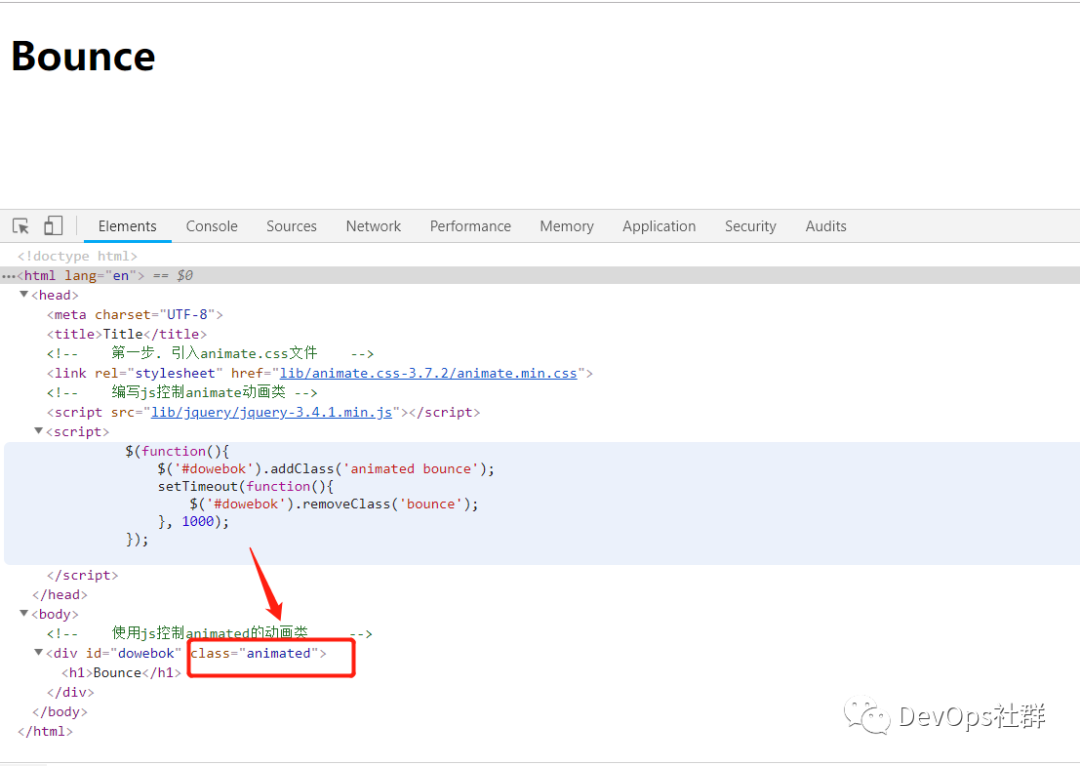
More animation effects can be viewed according to the online demonstration, as follows:
https://daneden.github.io/animate.css/
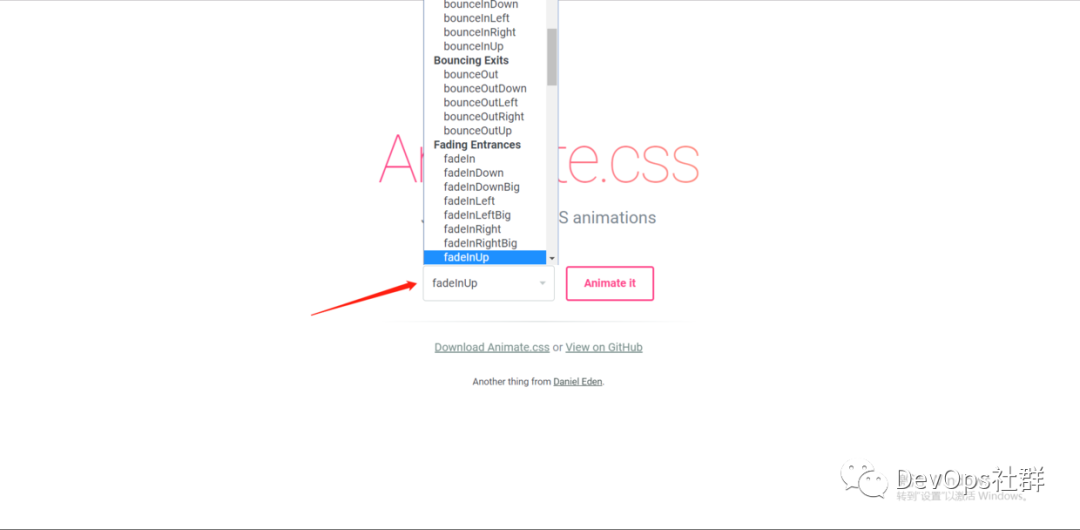
Let's take a look at how to apply it in the Vue framework.
Apply animate. In the Vue framework CSS Library
Apply css animation using enter active class and leave active class
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <!-- 2.Import animate.css --> <link rel="stylesheet" href="lib/animate.css-3.7.2/animate.min.css"> </head> <body> <div id="app"> <!-- use v-on binding click Event execution switching show variable,Used to control the following p Tagged v-if --> <button @click="show = !show"> Toggle render </button> <!-- set up transition,use enter-active-class Set the effect of admission, use leave-active-class Set the effect of departure --> <transition enter-active-class="animated bounceIn" leave-active-class="animated bounceOut" > <p v-if="show">hello</p> </transition> </div> <script> // 2. Create an instance of Vue var vm = new Vue({ el: '#app', data: { show: true }, }) </script> </body> </html>
The browser displays as follows:
When you click to hide "hello", use "bounce out" to display the effect of departure.
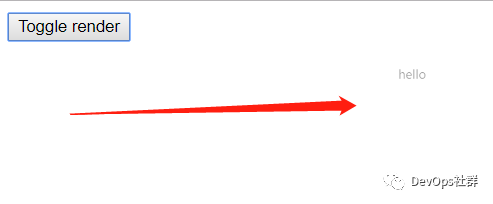
When clicking "hello", use "bounce in" to display the effect of admission.
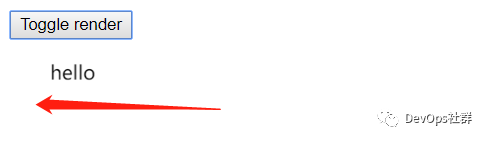
You can see from the above that animated needs to be written in both class es, as follows:
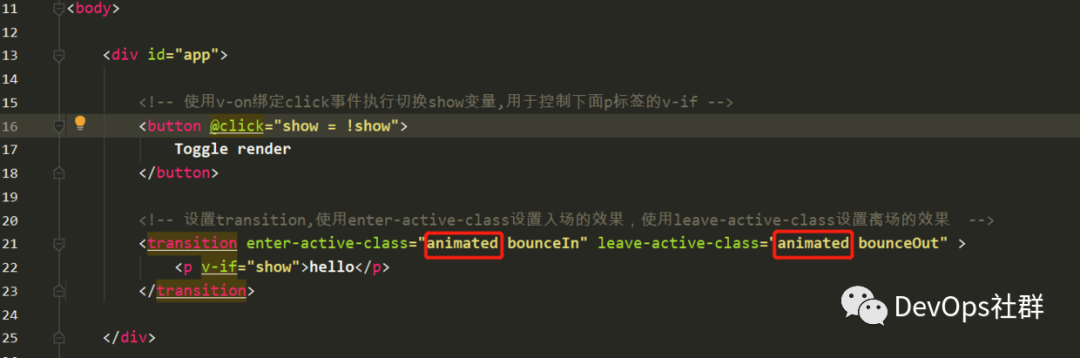
Can you optimize it? You don't have to write every class. It's so troublesome.
Optimize the filling position of animeted
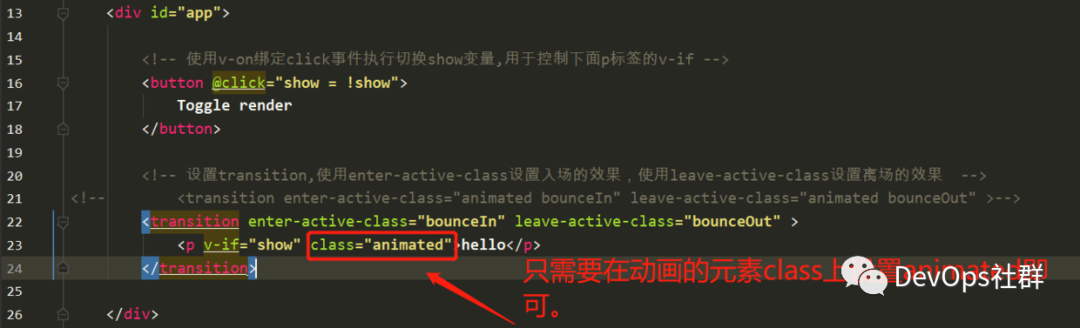
<!-- set up transition,use enter-active-class Set the effect of admission, use leave-active-class Set the effect of departure --> <transition enter-active-class="bounceIn" leave-active-class="bounceOut" > <p v-if="show" class="animated">hello</p> </transition>
Dominant transition duration
❝ 2.2.0 NEW ❞
In many cases, Vue can automatically determine the completion time of the transition effect. By default, Vue waits for its first transitionend or animationend event on the root element of the transition effect. However, it can not be set this way - for example, we can have a carefully arranged series of transition effects, in which some nested internal elements have delayed or longer transition effects than the root element of the transition effect.
In this case, you can customize an explicit transition duration (in milliseconds) with the duration attribute on the component:
<transition :duration="1000">...</transition>
You can also customize the duration of entry and exit:
<transition :duration="{ enter: 500, leave: 800 }">...</transition>
Use: duration to set a uniform run time for animation
The above only sets some animation effects, but if you need to set the running time of animation, you need to set "duration", as follows:

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <!-- 2.Import animate.css --> <link rel="stylesheet" href="lib/animate.css-3.7.2/animate.min.css"> </head> <body> <div id="app"> <!-- use v-on binding click Event execution switching show variable,Used to control the following p Tagged v-if --> <button @click="show = !show"> Toggle render </button> <!-- set up transition,use enter-active-class Set the effect of admission, use leave-active-class Set the effect of departure --> <transition enter-active-class="bounceIn" leave-active-class="bounceOut" :duration="200"> <p v-if="show" class="animated">hello</p> </transition> <hr> <transition enter-active-class="bounceIn" leave-active-class="bounceOut" :duration="1500"> <p v-if="show" class="animated">hello</p> </transition> </div> <script> // 2\. Create an instance of Vue var vm = new Vue({ el: '#app', data: { show: true }, }) </script> </body> </html>
The browser executes as follows:
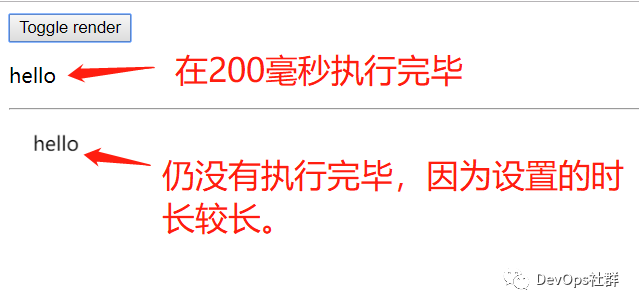
The above settings have the same running time for admission and departure. If splitting is required, it can be set separately as follows.
Use: duration to set the running time of the entrance and exit of the animation separately
Using the dictionary, you can set the running time of entry and exit separately.

<transition enter-active-class="bounceIn" leave-active-class="bounceOut" :duration="{ enter:200, leave:1500 }"> <p v-if="show" class="animated">hello</p> </transition>