preface
The previous chapter explained the basic usage of v-on monitoring events. This chapter introduces the event modifiers, which are mainly used to solve the problems of "prevent bubbling" and "prevent default events".
Event modifier:
- . stop stop bubbling
- . prevent block default events
- . capture use event capture mode when adding event listeners
- . self triggers a callback only when the event is triggered on the element itself (for example, not a child element)
- The. Once event is triggered only once
- Concatenation of event modifiers, for example: @ click prevent. Once, the default behavior will be blocked only once, and will not be blocked the second time. In addition, the two event modifiers have the same sequential effect.
The following is a case by case example of each modifier.
Example: Stop stop bubbling event
Write a button and Div. when the button is in the div, if you listen to the click event, the click event of the div will also start when you click the button by default. This is "event bubbling".
The example code is as follows:
<!DOCTYPE html> <html lang="en" xmlns:v-on="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>Title</title> <style> .inner{ width: 100%; height: 100px; background-color: aquamarine; } </style> </head> <body> <div id="app"> <div class="inner" @click="divHandler"> <input type="button" value="Button" @click="btnHandler"> </div> </div> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <script> // 2. Create an instance of Vue var vm = new Vue({ el: '#app', data: {}, methods:{ divHandler(){ console.log("trigger div of click event"); }, btnHandler(){ console.log("trigger btn of click event"); } } }) </script> </body> </html>
Execute the following in the browser:
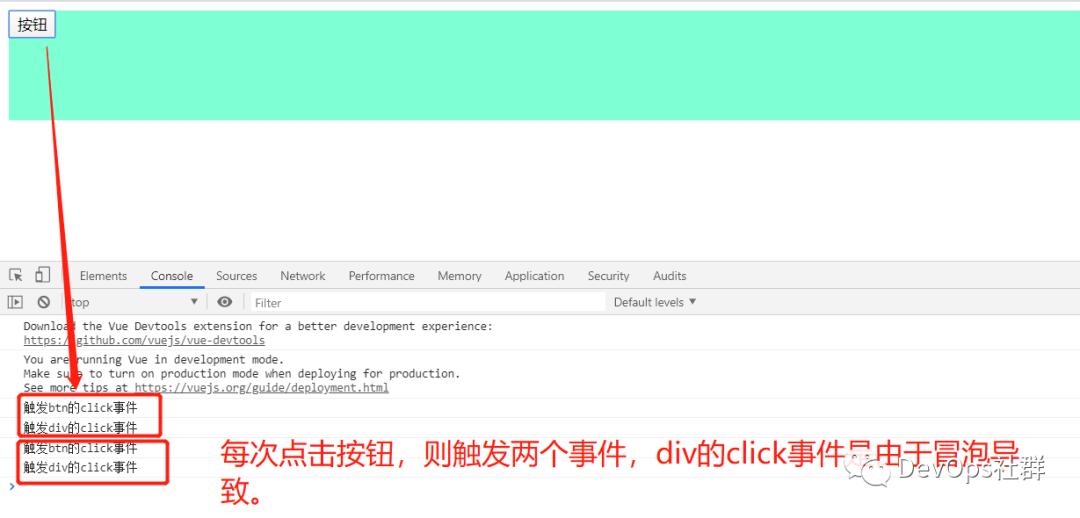
In order to "prevent bubbling events", you can use the "stop" event modifier as follows:
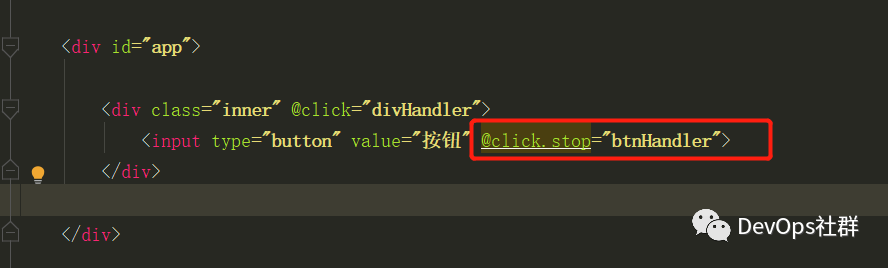
Click the button in the browser again to view the trigger events as follows:
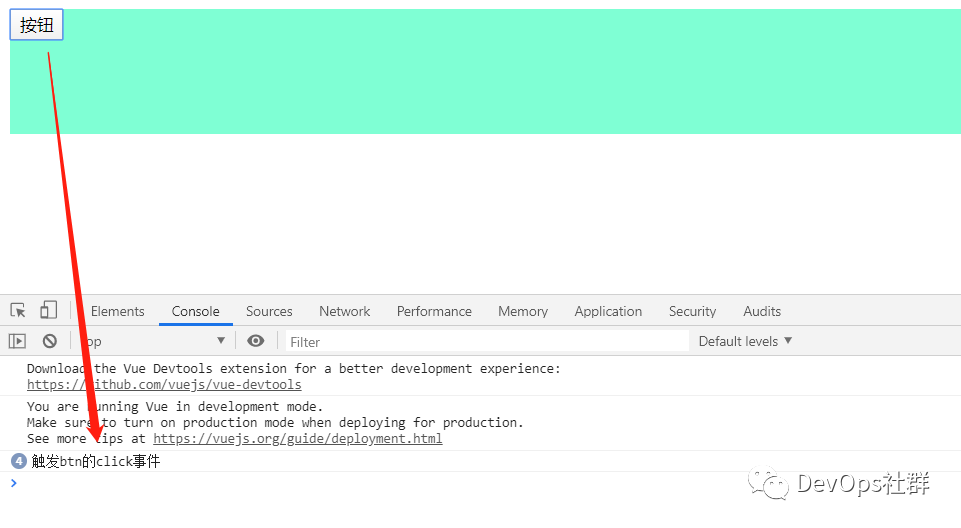
You can see that the click event of div has not been triggered, and the bubble of the event has been prevented.
Example: prevent block default events
Write an a tag and bind a click event to prevent the default href jump page behavior of a tag.
Examples are as follows:
<!DOCTYPE html> <html lang="en" xmlns:v-on="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8"> <title>Title</title> <style> .inner{ width: 100%; height: 100px; background-color: aquamarine; } </style> </head> <body> <div id="app"> <a href="https://www.baidu. Com "@ Click =" clickhandler "> visit Baidu</a> </div> <!-- 1.Import vue.js library --> <script src="lib/vue.js"></script> <script> // 2. Create an instance of Vue var vm = new Vue({ el: '#app', data: {}, methods:{ clickHandler(){ console.log("trigger click event"); }, } }) </script> </body> </html>
Click the a tag in the browser to automatically jump to Baidu page, as follows:
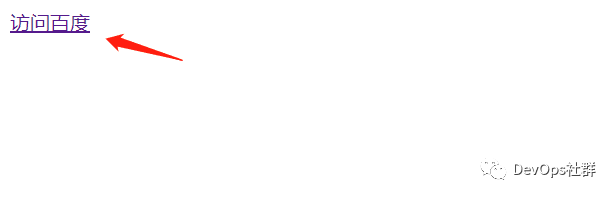
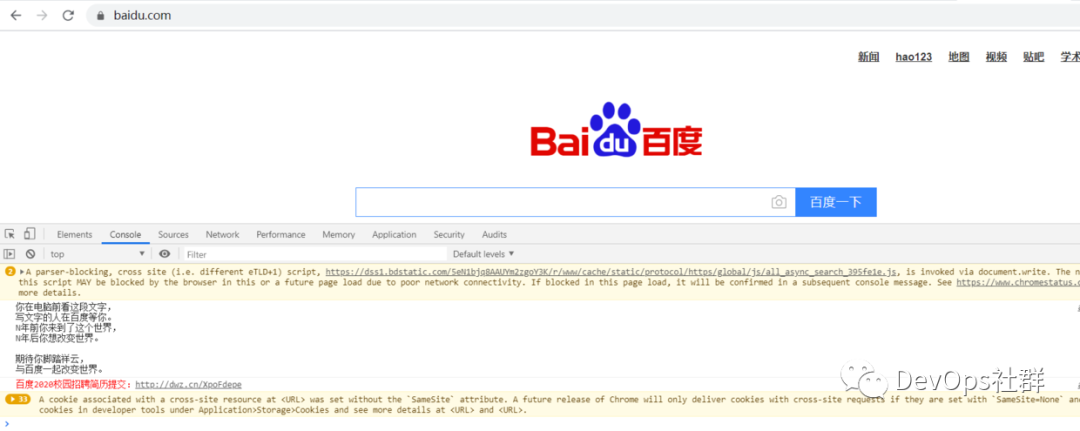
However, in many cases, the default behavior of a tag is not required. At this time, it is necessary to prevent the default behavior, as follows:
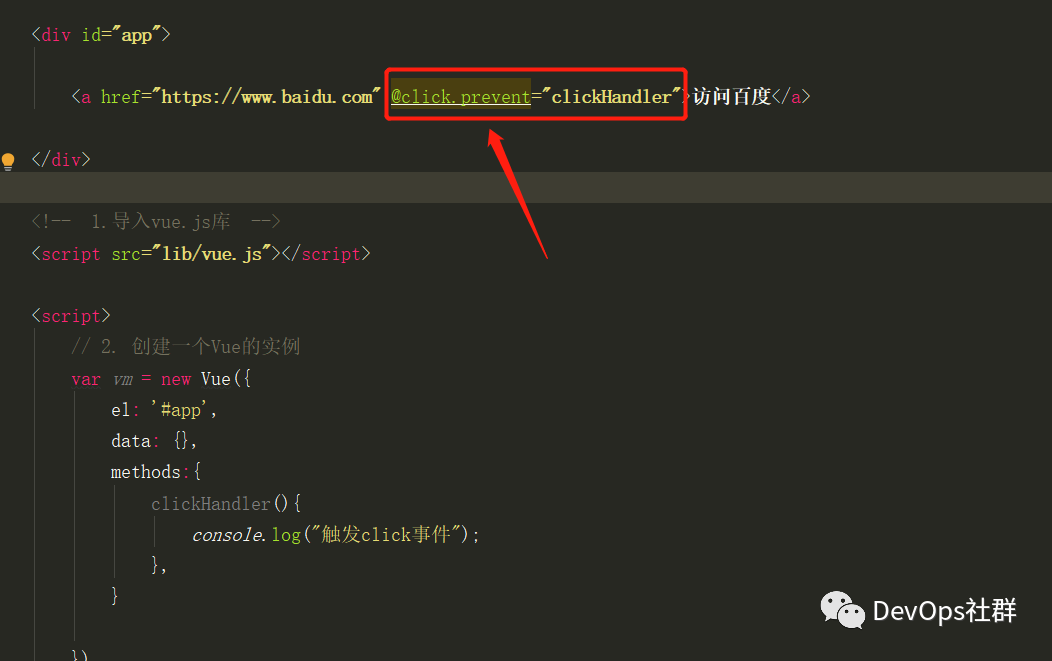
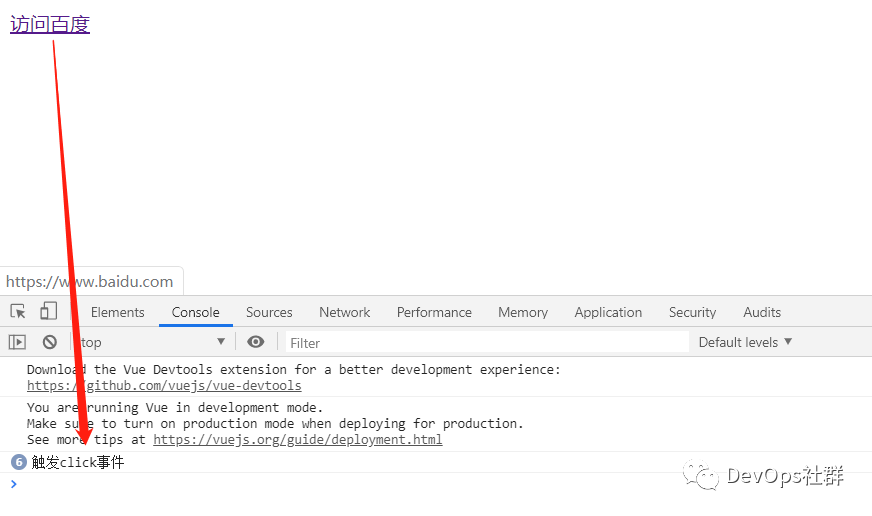
At this time, clicking the a tab will prevent the default behavior and only execute listening events.
Example: Capture uses event capture mode when adding event listeners
"Event capture mode" is actually the opposite event transmission mode of "bubble event", that is, the event trigger mode of "from outside to inside".
Let's take a look at the bubble example of the button and div just now:
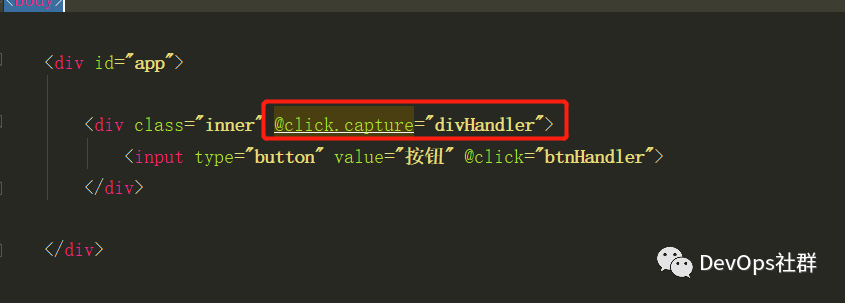
In the browser, click the button to view the sequence of triggering events, as follows:
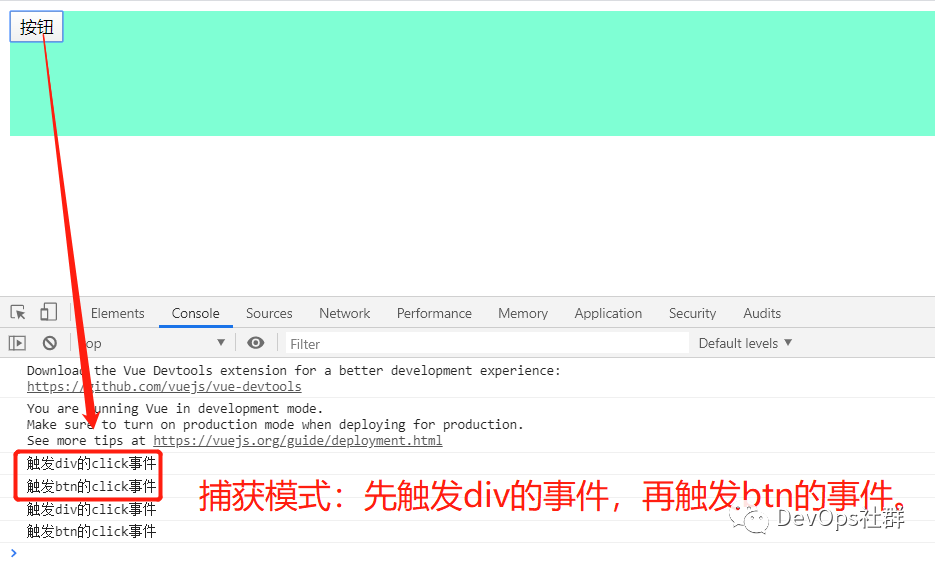
Example: self triggers a callback only when the event is triggered on the element itself (for example, not a child element)
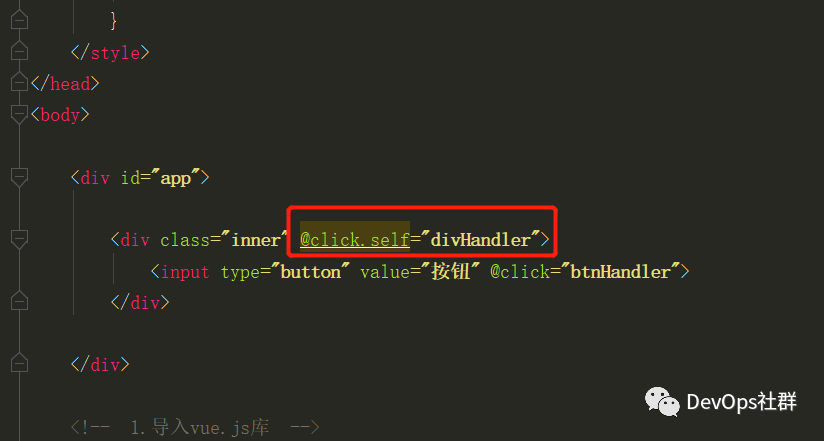
Click btn to view the trigger events as follows:
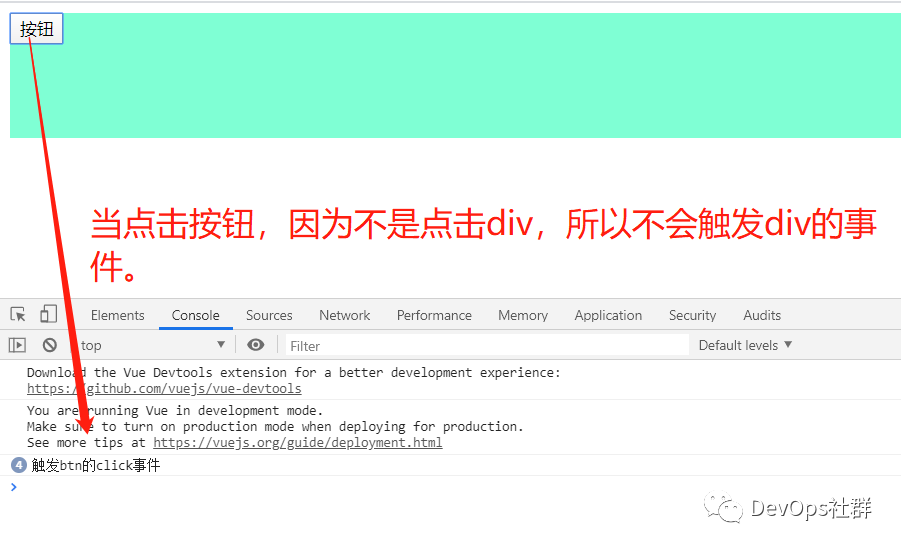
Click div to view the trigger event as follows:
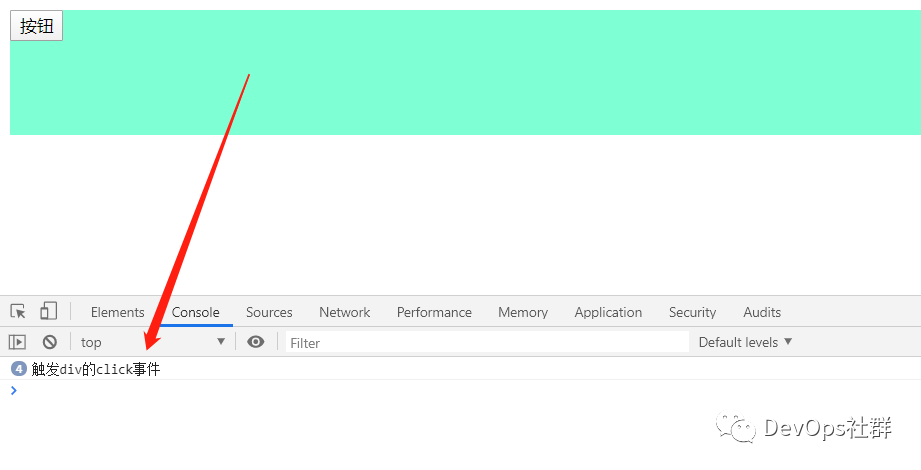
Example: The once event is triggered only once
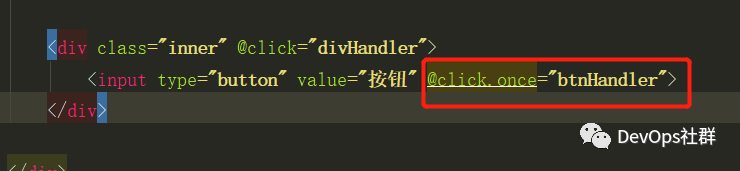
Click btn button several times to view the trigger event, as follows:
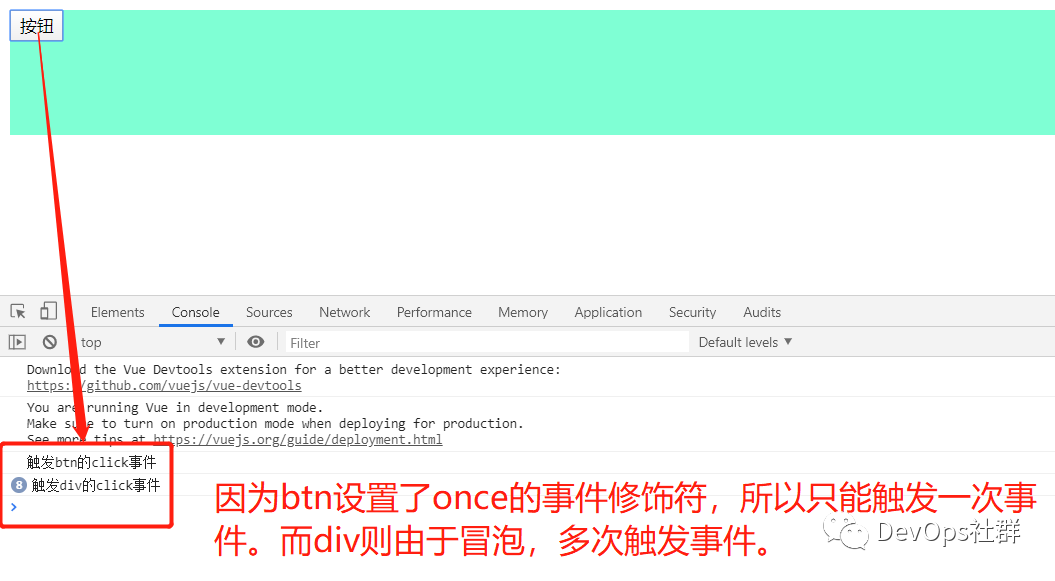
Example: concatenation of event modifiers, for example: @ click prevent. Once, the default behavior will be blocked only once, and will not be blocked the second time. In addition, the two event modifiers have the same sequential effect.

Click the a tab in the browser to view the trigger event, as follows:
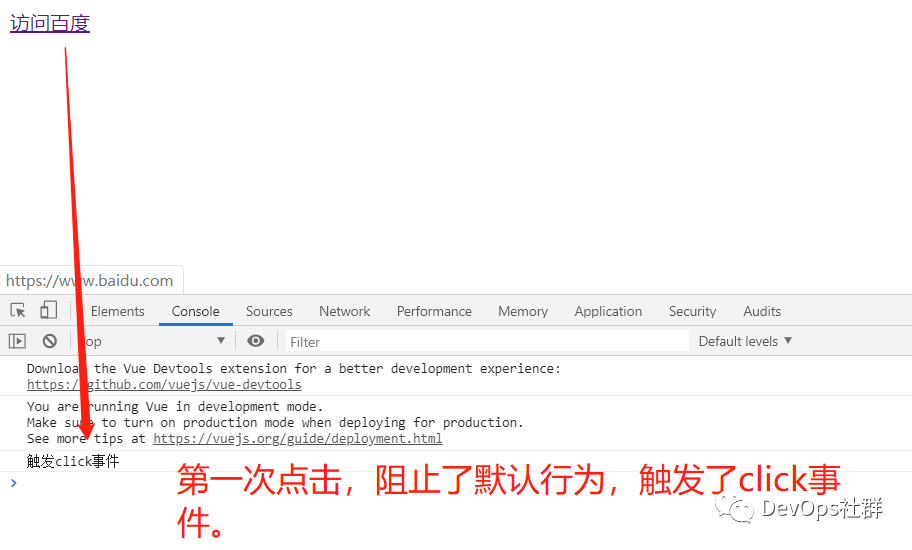
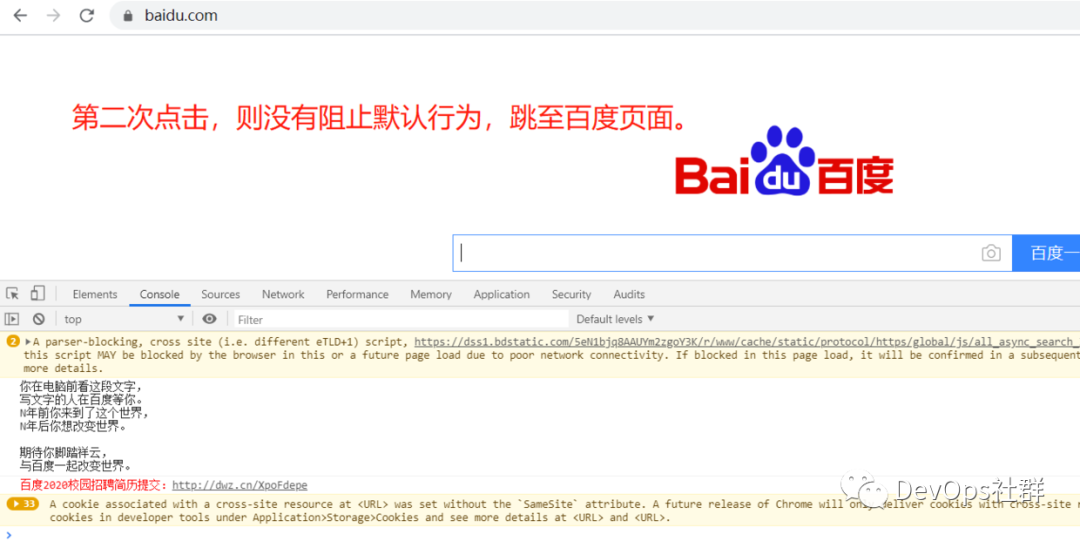