#vue3 knowledge point 5.3 vuecli3 project static resources
1, How to introduce static resources:
1. Static resource placement directory
vue-cli3.0 has two directories for static resources, public and src/assets.
1.public
public can store unchangeable files (equivalent to static in vue-cli2.x), external JS and CSS resources, and pictures.
The files in the public / directory will not be deleted Webpack Processing: they will be directly copied to the final packaging directory (dist/static by default). These files must be referenced by absolute path, which depends on the configuration of publicPath in vue.config.js. The default is /.
The static resources in the public directory will not be parsed by webpack when packaged, but can be directly referenced, that is, there is no need to write require(img.jpg). So it's best to put all static resources under public. js files with es6 cannot be placed.
1. <img src="/public/img/a.jpg"> 2. <img :src="imga"> data(){ imga:'/public/img/a.jpg' }
2.assets
assets stores files that may change and static resources such as pictures referenced by components img and BG image..
The files in the assets directory are deleted webpack Processing is resolved to module dependency, such as < img SRC = "..." >, Background: URL (), webpack will translate url(./img.png) into require('. / img.png')
The pictures stored in assets must be import ed first and then reused.
Only relative path form is supported.
2.1 webpack packaging tool
Webpack is a front-end resource loading / packaging tool. It will conduct static analysis according to the dependencies of modules, and then generate corresponding static resources according to the specified rules.
Webpack can convert various static resources js, css and less into a static file, reducing page requests.
When vue projects reference static resources, webpack is mainly resolved with require. In the project, enter index JS, the requirements in the loaded components belong to the parsing category of webpack.
2.2 require usage:
Only for directories placed in the assets folder:
Incorrect usage: you cannot assign a directory to a variable, and then require the variable.
// Code 1 error let url = "@/assets/images/carousel/logo.svg" require(url) // It is not advisable to simply pass a variable. The computer will scan all resources to find this.
Correct approach: you should specify the resource name with a variable, and then add this variable to the require d splicing directory.
// Code 2 is correct let url = "logo.svg" require("@/assets/images/carousel/"+url); // Although there are variables in require, the prefix is added to make the computer find them faster
3. Comparison between public and assets
Src / components / jingtaiziyuan5 in vue3demo1_ 3/5_ 31.vue
<template> <h4>1.Direct use</h4> <h6>public</h6> <img src="/public/favicon.ico" /> <h6>assets</h6> <img src="../../assets/logo.png" /> <h4>2.Binding variables</h4> <h6>public</h6> <img :src="img1" /> <h6>assets</h6> <img :src="img2" /> </template> <script> //Note here: img in assets must be imported before use. import imgurl from '../../assets/logo.png' export default { name: 'five_31', data() { return { img1:"/public/favicon.ico", img2:imgurl, } } } </script> <style> </style>
2, Import methods of js and css in components
Src / components / jingtaiziyuan5 in vue3demo1_ 3/5_ 32.vue
The following refer to static resources under public.
public
css
js
img
In the vuecli project, resources should follow the idea of modularization, export first and import when using. You can check the route index JS and main JS, we will find that index JS export first, main JS to import.
index.js export
main.js import
So let's summarize:
1. Introduce css into components
In the vue page where css needs to be imported, you can import it. css does not need to be exported.
<style scoped > @import url("../../../static/index/css/list.css"); </style>
scoped is used to make the css file private. It can only be used on the current component. Other components will not be used. If it is not needed, it can be omitted.
**Example: * * at 5_ Reference 5 in 32.vue_ 32. CSS, let < H5 > display red
5_ 32 Code:
<template> <h4>css and js Reference method of</h4> <h5>I am css References, rendering red</h5> </template> <script> export default { name: 'five_32', data() { return { } } } </script> <style scoped> @import url("../../../public/css/5_32.css"); </style>
vue file.
css file.
effect:
2. Introduce js into the component
Src / components / jingtaiziyuan5 in vue3demo1_ 3/5_ 33.vue
Generally, external js files are written by themselves because they use encapsulated functions. The defined js file must encapsulate the data and functions, export the encapsulated functions, and then import them into the vue component.
1.js file should encapsulate both data and functions. Then export(data,func).
5_32.js:
var data={ msg:'Import succeeded!', } var funclist={ func1:func1, func2:func2 } function func1(){ alert("func1--external js"+data.msg) } function func2(){ alert("func2---La La La") } export {data,funclist}
2. First introduce js under script, which is the exported object
In script, you call objects that are directly functions. Function.
When calling in the view layer, you need to execute the functions in methods first and bind the functions in methods.
5_32.vue
<template> <h4>css and js Reference method of</h4> <h5>I am css References, rendering red</h5> <!-- 3.When the view layer wants to use external js When you create a function, first put it in methods function a Execute in, and then call in the view layer a --> <button @click="funca">Click I execute 5_32.js in func1 function</button> </template> <script> //1. Introduce js first import {data,funclist} from '../../../public/js/5_32.js' // 2. It can be used directly under the script of vue component console.log(funclist.func2()) export default { name: 'five_32', data() { return { } }, methods:{ funca(){ funclist.func1() } } } </script> <style scoped> @import url("../../../public/css/5_32.css"); </style>
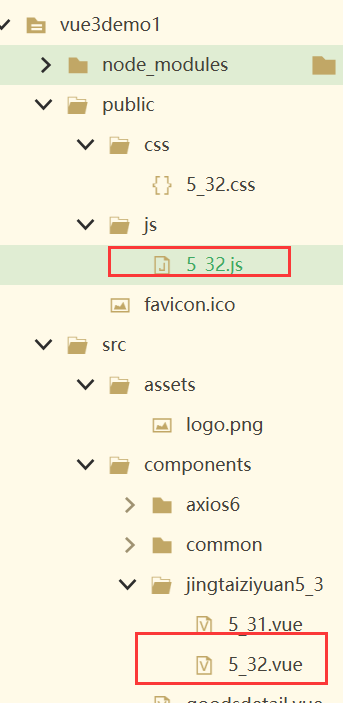