1. get request
Get requests are the ones we use most for fetching data.Here are a few examples of our get request parameter delivery.
1. Basic type parameters
This is the most basic way to pass parameters for get requests, and there's nothing particularly interesting to say.
2. Entities as parameters
Is it possible if we want to pass entity objects as parameters directly to the background when a get request is made?Let's have a look.
test Result: When a get request is made, we pass the json object directly as an entity to the background, which is not received.Why is that?Let's look at the corresponding http request
Originally, when a get request was made, the default was to put all the parameters in the url and pass them directly as string s, which would naturally not be received in the background.
Reason Analysis: Do you remember the interview question asking the difference between get and post requests?One difference is that the data requested by the get is appended to the URL (that is, the data is placed in the HTTP protocol header), while the post request is placed in the package of the HTTP protocol package.
As suggested by the gardeners, you can get the object directly by adding [FromUri] to the parameter when making a Get request.Or paste code:
Result:
If you don't want to use [FromUri] as a "bizarre" way of writing that adds attributes to parameters, you can also use serialization followed by back-end deserialization.
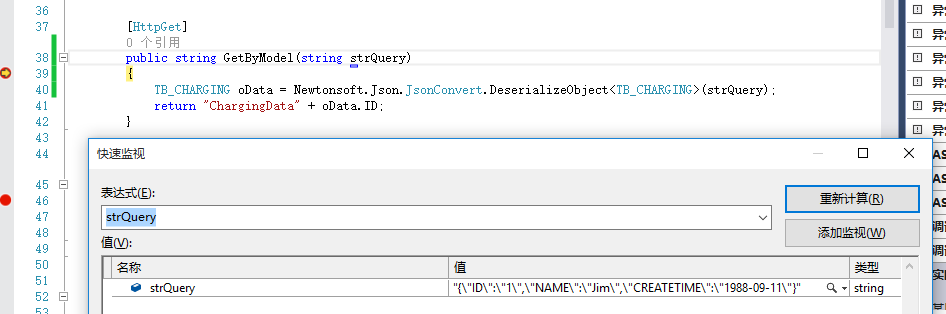
This gives us the object we serialized in the background, and then deserializes it.
In the url we can see that it automatically adds a code to the object:
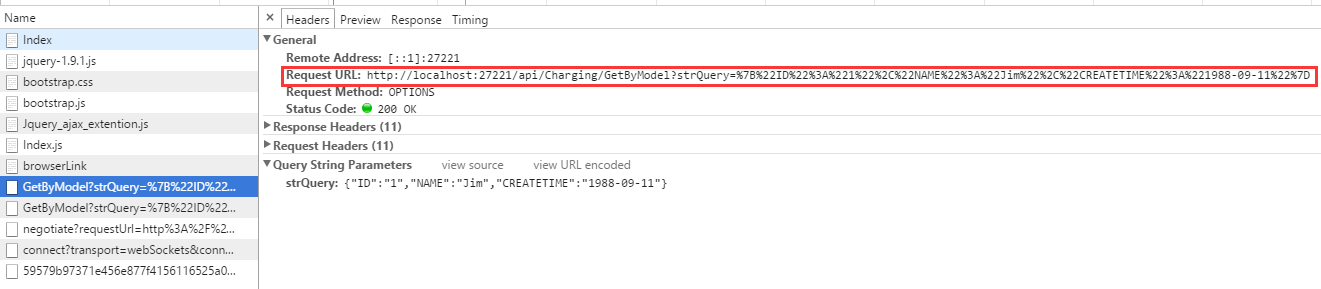
3. Array as parameter
Arrays are not recommended for general get requests because we know that get requests pass parameters with a limited size, a maximum of 1024 bytes, and passing them as parameters may result in loss of parameter overruns when there is more content in the array.
4.'Strange'get requests
Why would you say get requests are "weird"?Let's first look at the comparison of the two writing methods below.
(1) WebApi method names start with get
This is the standard notation, plus [HttpGet] in the background, and the parameters are normally obtained:
For comparison, I'll remove [HttpGet] and call it again
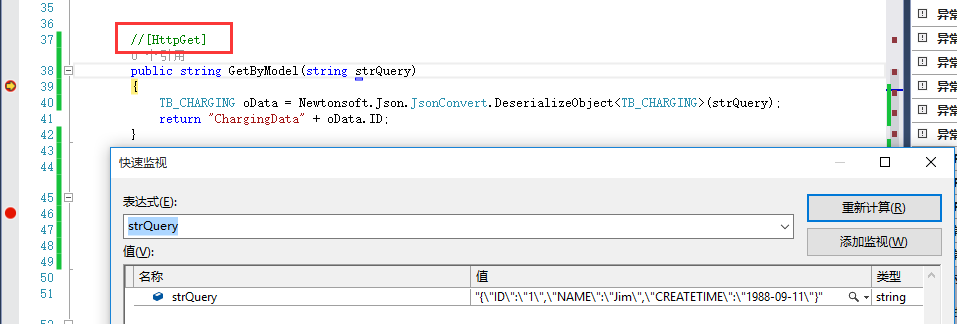
It looks like there's nothing wrong with it!Someone wonders if all get requests can omit the label [HttpGet].Let's try it.
(2) WebApi method names do not start with get
It's perfectly normal that we change the method name from GetByModel to FindByModel. Many queries don't want to start with Get, and they start with Query.Does this matter?It's okay. Let's tell the truth.
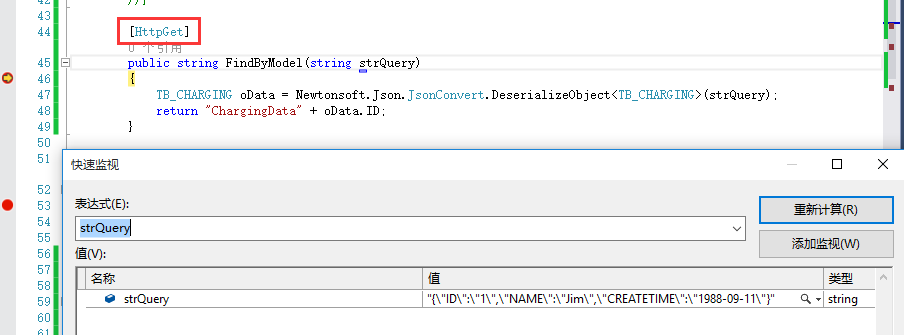
It looks like it's possible. There's nothing wrong with it.Based on the above deduction, it is also possible to remove [HttpGet]. Okay, let's comment out [HttpGet] and run it for a try.
The result is no breakpoints, some people don't believe, and we look at http requests in our browser:
Heh, that's strange. I changed the method name. As for that?Really!
The blogger's understanding is that the method name starts with Get, and WebApi automatically defaults that this request is a get request. If you start with a different name without labeling the method's request, the server finds this method at this time, but because the request is not sure, it returns you 405 directly - the method is not allowed.
Finally, the conclusion is that all WebApi methods are best served with a request ([HttpGet]/[HttpPost]/[HttpPut]/[HttpDelete]) and don't be lazy, as this will prevent similar errors and help maintain the method so that others can see what the request is.
That's why many people in the garden ask why a method name can't be called without [HttpGet]!
2. post Request
In the RESETful style of WebApi, the additions and deletions of API services correspond to the post/delete/put/get requests of http, respectively.Let's talk about how the post request parameter is passed.
1. Basic type parameters
The parameters of the basic type of post request and the get request are slightly different. We know that the parameters of the get request are passed through a url, while the post request is passed through the body of the http request, and the WebApi post request also needs to take the parameters from the body of the http request.
(1) Wrong writing
This is a very correct way of writing, but the reality is:
(2) Correct usage
This is a way of writing that many other people have a headache, but there is no way to get our results:
Our usual mechanism for taking parameters through a URL is a key-value pair, where a key equals a value, unlike our usual mechanism for taking parameters through a url, FromBody here has a mechanism of = value, no concept of key, and if you write a key (such as {NAME:"Jim"} for your ajax parameter), the NAME you get behind the scenes is null.Don't believe you can try it.
So what if we need to pass more than one base type?Based on the above inference, is it possible to write this ([FromBody]string NAME, [FromBody]string DES)?Just try it.
(1) Wrong Writing
Get results
This means we can't get values from multiple [FromBody], and this method fails.
(2) Correct usage
Since that doesn't work, how do we transfer multiple underlying types of data?Many solutions are to create a new class to contain the passed parameters, which the blogger feels is not flexible enough because if we create a new class every post request that passes multiple parameters in front and back, how many of these parameter classes will our system have in time?Maintaining it is quite a hassle!So bloggers think using dynamic is a good choice.Let's try it.
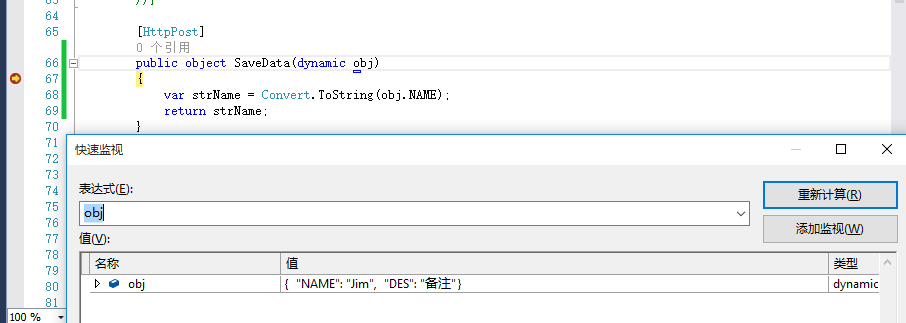
There is no sense of freshness in passing ajax parameters without using the {""value"} notation of"pointless". ~ It is important to note that the parameter type Json, contentType:'application/json', needs to be added to ajax requests here.
(3) Recommended usage
By requesting the passing of the underlying type parameter from post above, we understand the convenience of dynamic to avoid the cumbersome addition of {":""value"} to [FromBody].The blogger recommends that all base types be passed using dynamic, which facilitates the transfer of one or more parameters of the base type, as shown above.If the gardeners have better ways, welcome to discuss.
3. put Request
put requests in WebApi are typically used for object updates.It is essentially the same as a post request.[FromBody] is also supported, as is dynamic.
1. Basic type parameters
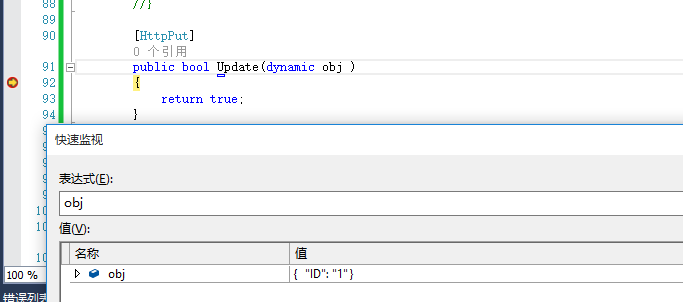
2. Entities as parameters
Same as post request.
3. Array as parameter
Same as post request.
IV. delete Request
As the name implies, the delete request must be for the delete operation.The parameter delivery mechanism and post are basically the same.Here is a simple example, other cases referring to post requests.
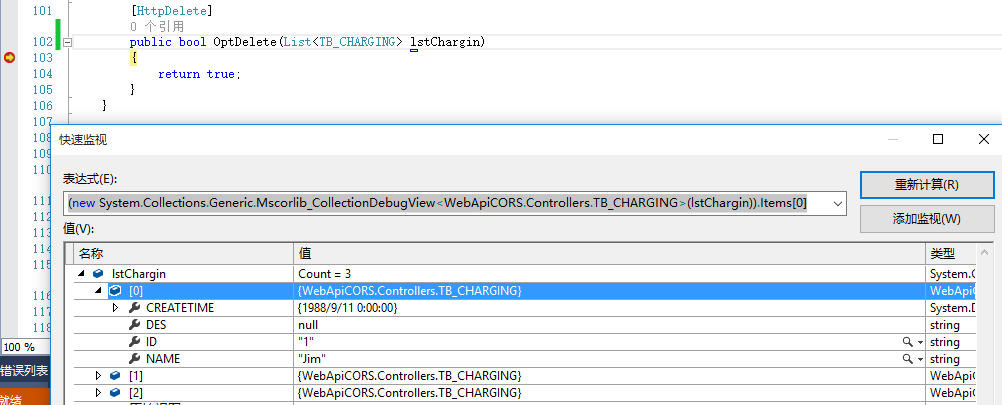
V. Summary
This is a more detailed summary of the various parameter passes for various requests for WebApi.Every situation has been tested by the blogger's actual code, and the content is not difficult, but if it takes a little time to get familiar with something like this, here's a summary, hoping to help some new WebApi friends.If this article can help you, you might as well recommend that your recommendation be the motivation for bloggers to continue summarizing!