Concept of inheritance
Inheritance is one of the three characteristics of object-oriented. It can make subclasses have the properties and methods of parent classes. At the same time, it can redefine and append properties and methods in subclasses.
Format: class subclass extends parent class {}
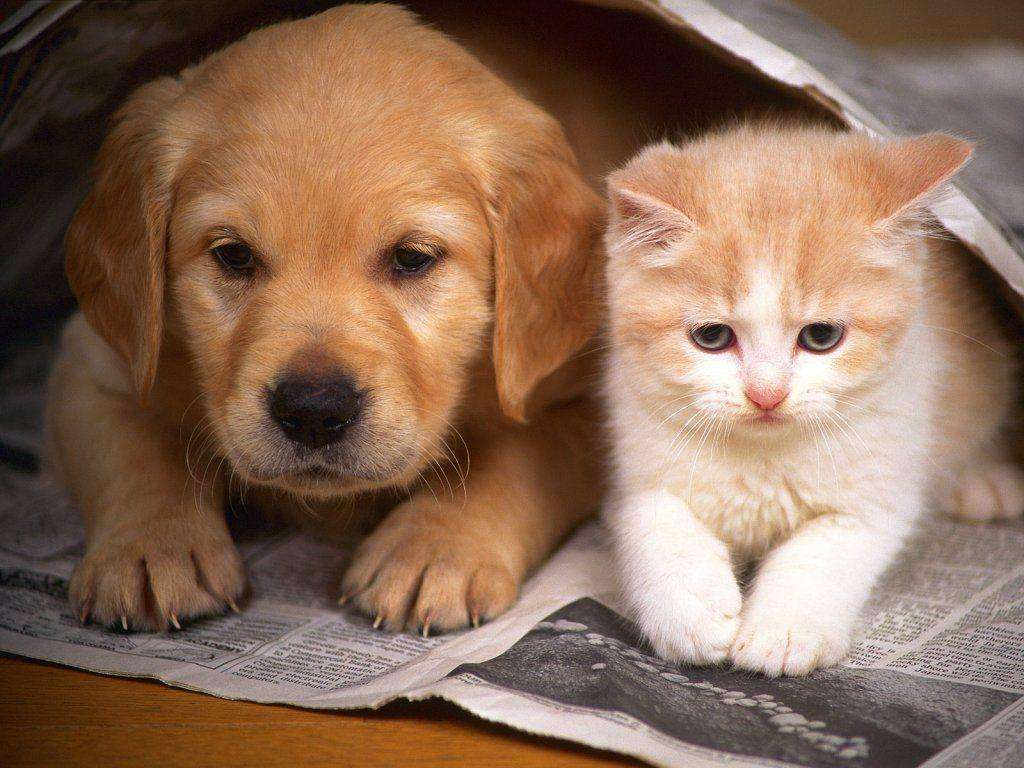
// Animal (parent) class Animal { public void eat() { System.out.println("Animals eat"); } } // Dogs (subclass) class Dog extends Animal { } // Cats (subclasses) class Cat extends Animal { } // Demo class public class InheritanceDemo { public static void main(String[] args) { Dog erha = new Dog(); erha.eat(); Cat tom = new Cat(); tom.eat(); } }
Operation results
Animals eat Animals eat
Code interpretation
- First, we define a parent class, Animal class, so that all animals have the same attribute, that is, eating, so we define an eat() method.
- Then we define two subclasses: Dog class and Cat class. Cats and dogs are also animals. Therefore, we use the extends keyword after dog class and Cat class to inherit the Animal class.
- Finally, during the test, we directly new the subclass. After the subclass object is new, because it inherits the parent Animal class, this subclass object can directly access the eat() method of the parent Animal class, which is inheritance.
Continue to explore
Since the methods of the parent class can be accessed, can the variables of the parent class also be accessed?
The answer is yes, on the code
// Animal (parent) class Animal { public int age = 2; public void eat() { System.out.println("Animals eat"); } } // Dogs (subclass) class Dog extends Animal { } // Cats (subclasses) class Cat extends Animal { } // Demo class public class InheritanceDemo2 { public static void main(String[] args) { Dog erha = new Dog(); erha.eat(); System.out.println(erha.age); Cat tom = new Cat(); tom.eat(); System.out.println(tom.age); } }
Operation results
Animals eat 2 Animals eat 2
New problems
In the above example, the subclass calls the eat() method, and the output is "animals eat". However, what cats and dogs like to eat is different. Can you show what cats and dogs eat in detail? It is obviously impossible to directly modify the methods in the parent class. Adding a new method in the child class obviously violates the original intention of inheritance. Then this is the time to rewrite the method. For details, please refer to Another note: Java Method Overriding.
Code directly on this side:
// Animal (parent) class Animal { public void eat() { System.out.println("Animals eat"); } } // Dogs (subclass) class Dog extends Animal { public void eat() { System.out.println("Dogs eat bones"); } } // Cats (subclasses) class Cat extends Animal { public void eat() { System.out.println("Cats eat fish"); } } // Demo class public class InheritanceDemo3 { public static void main(String[] args) { Dog erha = new Dog(); erha.eat(); Cat tom = new Cat(); tom.eat(); } }
Operation results
Dogs eat bones Cats eat fish
super keyword
If in a subclass, I want to call the method of the parent class or access the member variable in the parent class, this super keyword should be used.
// Animal (parent) class Animal { public int age = 2; public void eat() { System.out.println("Animals eat"); } } // Dogs (subclass) class Dog extends Animal { public int age = 3; public void eat() { System.out.println("Dogs eat bones"); super.eat(); // Get the eat() method of the parent class } public void getAge(){ System.out.println(super.age); // Gets the age of the parent class } } // Cats (subclasses) class Cat extends Animal { public int age = 4; public void eat() { System.out.println("Cats eat fish"); super.eat(); // Get the eat() method of the parent class } public void getAge(){ System.out.println(super.age); // Gets the age of the parent class } } // Demo class public class InheritanceDemo4 { public static void main(String[] args) { Dog erha = new Dog(); erha.eat(); erha.getAge(); Cat tom = new Cat(); tom.eat(); tom.getAge(); } }
Operation results
Dogs eat bones Animals eat 2 Cats eat fish Animals eat 2