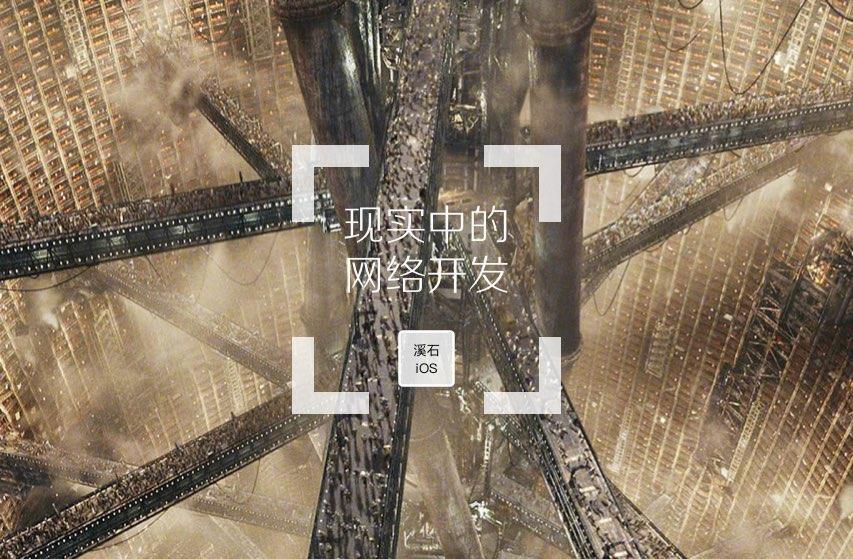
Network API in Reality
json has become the standard data format of the modern network api, such as the query interface of the National Meteorological Administration.
http://www.weather.com.cn/data/sk/101010100.html
{ "weatherinfo": { "city": "Beijing", "cityid": "101010100", "temp": "18", "WD": "Southeast wind", "WS": "1 level", "SD": "17%", "WSE": "1", "time": "17:05", "isRadar": "1", "Radar": "JC_RADAR_AZ9010_JB", "njd": "No facts at present", "qy": "1011", "rain": "0" } }
The format of json is as follows:
- Object: An object begins with {and ends with}
- collection: Use between name and value: Separate, generally in the form of:
{name:value}
iOS Network Request in Reality
Because the method provided by iOS's native framework, NSURLSession, requires many underlying parameters, which are not intuitive to use and unstable to handle errors. In actual development, we will choose the network framework. AFNetworking is the first choice in this respect. It not only encapsulates the request of NSURLSession, but also automatically assigns the request queue, formats the return value, and converts the json string into a dictionary. All in all, it saves time and effort.
There are objects everywhere like air.
In iOS development, any data is based on OC objects, so the question arises: how can the requested json data be converted into usable objects?
One practice is to define a set of objects to be used by the APP side, and then implement the method of conversion from dictionary one by one. Each request needs to implement a set of objects by itself. This coding process is cumbersome (and repetitive), not to mention, the number of interfaces is too large, and it is easy to confuse.
In reality, we still use some excellent frameworks to help us make these transformations. Here we choose YYModel, which is a sub-project of YYKit and can be used alone.
Using the introduction of pod, add in the Podfile file:
pod 'YYModel'
Function:
pod update
Introduce header files:
#import <YYModel.h>
First, you need to define objects:
@interface WeatherInfo : NSObject @property(nonatomic, copy) NSString *city; @property(nonatomic, copy) NSString *cityid; @property(nonatomic, copy) NSString *temp; @property(nonatomic, copy) NSString *WD; @property(nonatomic, copy) NSString *WS; @property(nonatomic, copy) NSString *SD; @property(nonatomic, copy) NSString *WSE; @property(nonatomic, copy) NSString *time; @property(nonatomic, copy) NSString *isRadar; @property(nonatomic, copy) NSString *Radar; @property(nonatomic, copy) NSString *njd; @property(nonatomic, copy) NSString *qy; @property(nonatomic, copy) NSString *rain; @end
Property names are consistent with json fields so that they can be converted correctly.
Next, AFNetworking requests the network interface:
AFHTTPSessionManager *manager = [AFHTTPSessionManager manager]; manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"text/plain",@"text/html",nil]; [manager GET:@"http://www.weather.com.cn/data/sk/101010100.html" parameters:nil progress:nil success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) { NSLog(@"%@", responseObject); WeatherInfo *weather = [WeatherInfo yy_modelWithDictionary:responseObject[@"weatherinfo"]]; NSLog(@"%@", weather); } failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) { }];
The key is WeatherInfo * weather = [WeatherInfo yy_model WithDictionary: responseObject [@ "weatherinfo"]; directly converting json (dictionary here) into a WeatherInfo object.
Sometimes the field names returned by the network do not conform to the OC code specification. YYModel also provides a solution with care:
#import "WeatherInfo.h" @implementation WeatherInfo + (NSDictionary *)modelCustomPropertyMapper { return @{@"windDirection" : @"WD"}; } @end
By implementing the modelCustomPropertyMapper method, we can manually specify the transformed attribute name.
In this way, most of the network requests in real-world development can be automatically completed. Finally, json can be automatically converted into object declarations through the ModelMaker tool:

Download address:
https://pan.baidu.com/s/1slEss13
Unzip password: xishiios