Have a good laugh
[The weather was hot and the cabinet was turned upside down for half a day. Find out that all the famous short sleeves feel too high-profile to wear out, such as what China Telecom Ah Tianyi 4G Ah Mrs. Le Chi Jing Ah Lotus flower Ah Hai Tian soy sauce.The most precious one is that Stanley Compound Fertilizer tangled with Liu Neng who should wear it?You won't be said to be glamorous when you go out)
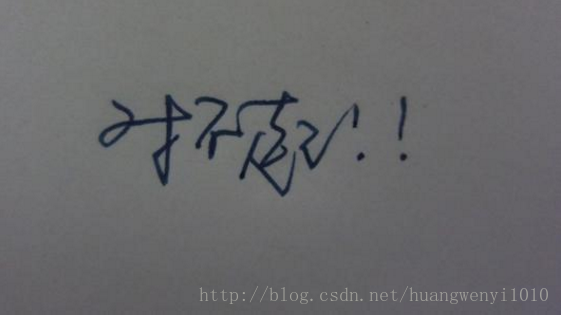
Ask questions
How do I use Java + FreeMarker to generate enumerated classes and related database scripts???
Solve the problem
Business Scenarios
When doing business development, we usually define many enumeration classes, write java enumeration classes, insert corresponding dictionary values in the database, etc., and then generate sql files to submit to the incremental script of SVN.To solve these problems, it is necessary to develop an enumeration and script auto-generated tool class, auto-generate enumeration classes and incremental scripts, and avoid simple and unnecessary errors.
This shared tool class is not for every company, because each company has a framework for each company, just to communicate an implementation: develop more tool classes for your company, reduce repetitive work, and focus more time and effort on important things.
code implementation
EnumGenerateUtils Tool Class
In our project, enumerations are categorized.
package com.evada.inno.pm.code.generate.util; import com.evada.inno.pm.code.generate.model.EnumDictCategoryDefinition; import com.evada.inno.pm.code.generate.model.EnumInfoDefinition; import freemarker.template.Template; import java.io.*; import java.text.SimpleDateFormat; import java.util.*; /** * Description: Enumerate class code generation tools * Created by Ay on 2017/5/5. */ public class EnumGenerateUtils { //Enumeration needs to be generated under that package private final String packageName = "com.evada.pm.process.manage"; //Enumerated classified code must be identical to categoryCode in dictionary table private final String enumCategoryCode = "TM_XXXX_XXXX_XXXX"; private final String enumCategoryName = "project management-Control mode-enumeration"; //Enumerate class names private final String enumClassName = "Color"; //Annotations on classes after enumeration class generation private final String enumAnnotation = "colour"; //It's important here private final String[][] ENUM_INFO = { {"BLUE","blue"}, {"YELLOW","yellow"}, {"BLACK","black"} }; //Authors on Classes private final String AUTHOR = "Ay"; //Date on class private final String CURRENT_DATE = new SimpleDateFormat("yyyy-MM-dd").format(new Date()); public static void main(String[] args) throws Exception{ EnumGenerateUtils enumGenerateUtils = new EnumGenerateUtils(); //Generate enumeration class java file enumGenerateUtils.generateEnumClassFile(); //Generate sql script file enumGenerateUtils.generateEnumSqlFile(); } private void generateEnumSqlFile() throws Exception{ final String suffix = ".sql"; final String path = "D://" + CURRENT_DATE + suffix; final String templateName = "EnumSQL.ftl"; File mapperFile = new File(path); Map<String,Object> dataMap = new HashMap<>(); List<EnumInfoDefinition> enumInfoDefinitionList = new ArrayList<>(); EnumInfoDefinition enumInfoDefinition = null; for(int i=0;i < ENUM_INFO.length;i++){ enumInfoDefinition = new EnumInfoDefinition(); enumInfoDefinition.setEnumUuid(EnumGenerateUtils.generateUUID()); enumInfoDefinition.setEnumCode(ENUM_INFO[i][0]); enumInfoDefinition.setEnumName(ENUM_INFO[i][1]); enumInfoDefinition.setEnumCategoryCode(enumCategoryCode); enumInfoDefinition.setEnumNumber(i + 1 + ""); enumInfoDefinition.setEnumSortOrder(i + 1 + ""); enumInfoDefinitionList.add(enumInfoDefinition); } EnumDictCategoryDefinition dictCategory = new EnumDictCategoryDefinition(); dictCategory.setEnumUuid(EnumGenerateUtils.generateUUID()); dictCategory.setEnumCategoryCode(enumCategoryCode); dictCategory.setEnumCategoryName(enumCategoryName); dataMap.put("enum_dict_catagory",dictCategory); dataMap.put("enum_list", enumInfoDefinitionList); generateFileByTemplate(templateName,mapperFile,dataMap); } private void generateEnumClassFile() throws Exception{ final String suffix = "Enum.java"; final String path = "D://" + enumClassName + suffix; final String templateName = "EnumClass.ftl"; File mapperFile = new File(path); Map<String,Object> dataMap = new HashMap<>(); List<EnumInfoDefinition> enumInfoDefinitionList = new ArrayList<>(); EnumInfoDefinition enumInfoDefinition = null; for(int i=0;i < ENUM_INFO.length;i++){ enumInfoDefinition = new EnumInfoDefinition(); enumInfoDefinition.setEnumUuid(EnumGenerateUtils.generateUUID()); enumInfoDefinition.setEnumCode(ENUM_INFO[i][0]); enumInfoDefinition.setEnumName(ENUM_INFO[i][1]); enumInfoDefinition.setEnumNumber(i + 1 + ""); enumInfoDefinition.setEnumSortOrder(i + 1 + ""); enumInfoDefinition.setEnumCategoryCode(enumCategoryCode); enumInfoDefinitionList.add(enumInfoDefinition); } dataMap.put("enum_list", enumInfoDefinitionList); generateFileByTemplate(templateName,mapperFile,dataMap); } private void generateFileByTemplate(final String templateName,File file,Map<String,Object> dataMap) throws Exception{ Template template = FreeMarkerTemplateUtils.getTemplate(templateName); FileOutputStream fos = new FileOutputStream(file); dataMap.put("author",AUTHOR); dataMap.put("date",CURRENT_DATE); dataMap.put("package_name",packageName); dataMap.put("enum_annotation",enumAnnotation); dataMap.put("enum_class_name",enumClassName); Writer out = new BufferedWriter(new OutputStreamWriter(fos, "utf-8"),10240); template.process(dataMap,out); } public static String generateUUID(){ return UUID.randomUUID().toString().replace("-", ""); } }
EnumClass.ftl Class
package ${package_name}.enums; /** * Description: ${enum_annotation} Type Enumeration * @author ${author} * @date ${date} */ public enum ${enum_class_name}Enum { <#if enum_list?exists> <#list enum_list as enum> ${enum.enumCode}("${enum.enumNumber}","${enum.enumName}")<#if enum_index == ((enum_list?size) - 1)>;<#else >,</#if> </#list> </#if> private final String code; private final String name; ${enum_class_name}Enum(String code, String name) { this.code = code; this.name = name; } public String getName() { return this.name; } @Override public String toString() { return this.code; } }
EnumSQL.ftl Class
-- ${enum_annotation}Enumeration Dictionary <#if enum_list?exists> <#list enum_list as enum> INSERT INTO "public"."sys_dict" ("id", "name", "code", "category_code", "parent_code", "sort_order", "is_updatable", "status") VALUES ('${enum.enumUuid}', '${enum.enumName}', '${enum.enumCode}', '${enum.enumCategoryCode}', NULL, '${enum.enumSortOrder}', NULL, '1'); </#list> </#if> -- ${enum_annotation}Enumerate Dictionary Classifications <#if enum_dict_catagory?exists> INSERT INTO "public"."sys_dict_category" ("id", "name", "code", "parent_code", "sort_order", "description", "is_updatable", "status") VALUES ('${enum_dict_catagory.enumUuid}', '${enum_dict_catagory.enumCategoryName}', '${enum_dict_catagory.enumCategoryCode}', '', '0', NULL, '0', '1'); </#if>
Reading Inspiration
From Dante's Divine Comedy
- Nothing is more painful than recalling the happiness of the past.
- Go your own way and let others talk.
- If you love, please love cleanly and give love to love.
- When a thing becomes more perfect, it becomes more painful and happy.
Classic Stories
[Once I went to the ocean with a friend.
A passenger asked the administrator, "How old will this shark grow?"
The administrator points to the aquarium and says, "It depends on how big your aquarium is."
The passenger asked again, "Will it be as big as the aquarium?"
The administrator said carefully: "If the shark is only a few meters in size in the aquarium, if it is in the ocean, it will be as big as swallowing a lion."
General Principle: Environment can change a person's mind.Environment can limit people's thoughts, and people can also limit their own.Don't frame yourself. When you can't change the environment, start by changing yourself.]
Common sense of life
There are six things you shouldn't do after exercising:
- Don't squat
- Don't be greedy about cold drinks
- Don't eat right away
- Do not drop your body temperature abruptly
- Don't smoke
- Do not drink water immediately
Great God articles
nothing
Other
If you have a little happiness to give you, let it continue to pass on. Welcome to praise, top, leave valuable comments and thank you for your support!